You can add error bars to a bar graph in MATLAB by using the `bar` function alongside the `errorbar` function to display variability in your data.
Here’s a simple code snippet demonstrating how to create a bar graph with error bars:
% Sample data
data = [10, 15, 20];
errors = [1, 2, 1]; % Error values
% Create a bar graph
b = bar(data);
% Hold on to add error bars
hold on;
% Get the x locations of the bars
x = b.XData;
% Add error bars
errorbar(x, data, errors, 'k', 'linestyle', 'none');
% Hold off to stop adding
hold off;
% Labels and title
xlabel('Categories');
ylabel('Values');
title('Bar Graph with Error Bars');
Understanding Error Bars
What are Error Bars?
Error bars are graphical representations that show the variability or uncertainty of data in a graph. They convey the range of uncertainty around a data point, often indicating how much the observed values may differ from the true values. Commonly used in scientific research and data analysis, error bars can represent various statistical measures such as:
- Standard Deviation: Illustrates how spread out the data points are from the average.
- Standard Error: Represents the precision of the sample mean estimate of a population mean.
- Confidence Intervals: Indicates the range within which a certain percentage of the data points would fall.
Why Use Error Bars in Graphs?
Using error bars is crucial for effective data visualization. They provide an immediate visual cue to the reader about data reliability and variability. Error bars can significantly influence data interpretation—even small error bars can suggest that data points are consistent, whereas large error bars may raise questions about the validity of the data.
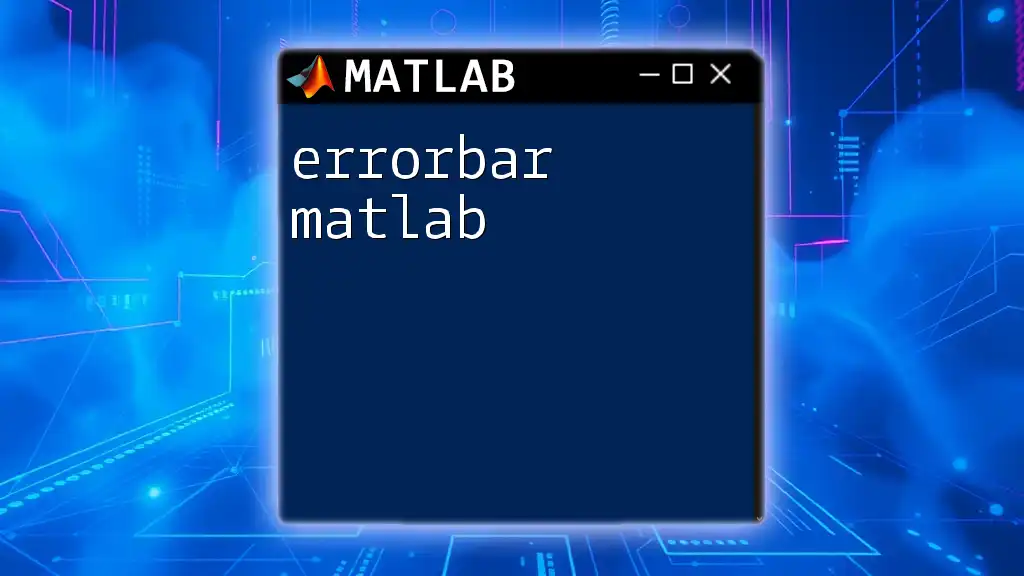
Creating a Basic Bar Graph in MATLAB
Setting Up Your Environment
Before you dive into creating bar graphs with error bars in MATLAB, make sure you have MATLAB open and ready to go. One of MATLAB's strengths is its ease of use for graphical data representations.
Code Snippet for a Basic Bar Graph
To create a basic bar graph, you can use the following code:
data = [5, 10, 15]; % Sample data
bar(data); % Create a bar graph
title('Basic Bar Graph');
xlabel('Categories');
ylabel('Values');
In this example:
- `bar(data)` creates a simple bar graph using the sample data provided.
- `title`, `xlabel`, and `ylabel` functions add standard labels to the graph.
Executing this code will generate a straightforward bar graph.
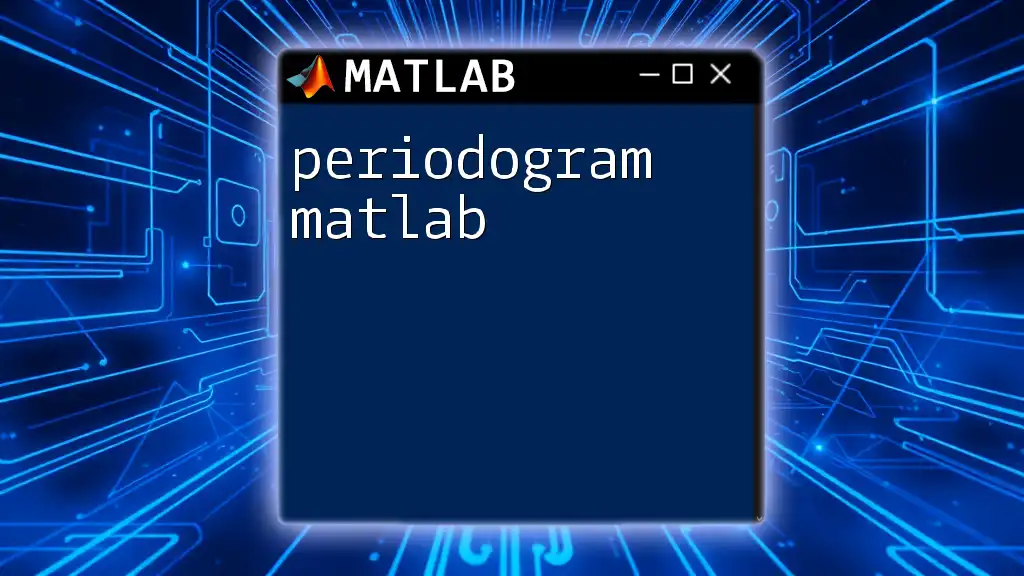
Adding Error Bars to a Bar Graph
Preparing Your Data
To effectively incorporate error bars, you need two sets of data: your main data points and the corresponding error values. For instance, if you measured the heights of three different plants and have the means along with their respective error margins, you would format them as follows:
data = [5, 10, 15]; % Sample data
errors = [1, 2, 1]; % Error values
Code Snippet for Adding Error Bars
To add error bars to your bar graph, combine your main data with the error values using the `errorbar` function:
data = [5, 10, 15]; % Sample data
errors = [1, 2, 1]; % Error values
bar_handle = bar(data); % Create a bar graph
hold on; % Hold on to the current graph
errorbar(bar_handle.XData, data, errors, 'k', 'linestyle', 'none'); % Add error bars
title('Bar Graph with Error Bars');
xlabel('Categories');
ylabel('Values');
hold off; % Release the graph
In this code:
- You first create the bar graph with `bar(data)`.
- The `hold on` command retains the current graph while you add the error bars.
- The `errorbar` command plots error bars directly above the corresponding bars. The parameters include color and a specification to avoid connecting lines between error bars (`'linestyle', 'none'`).
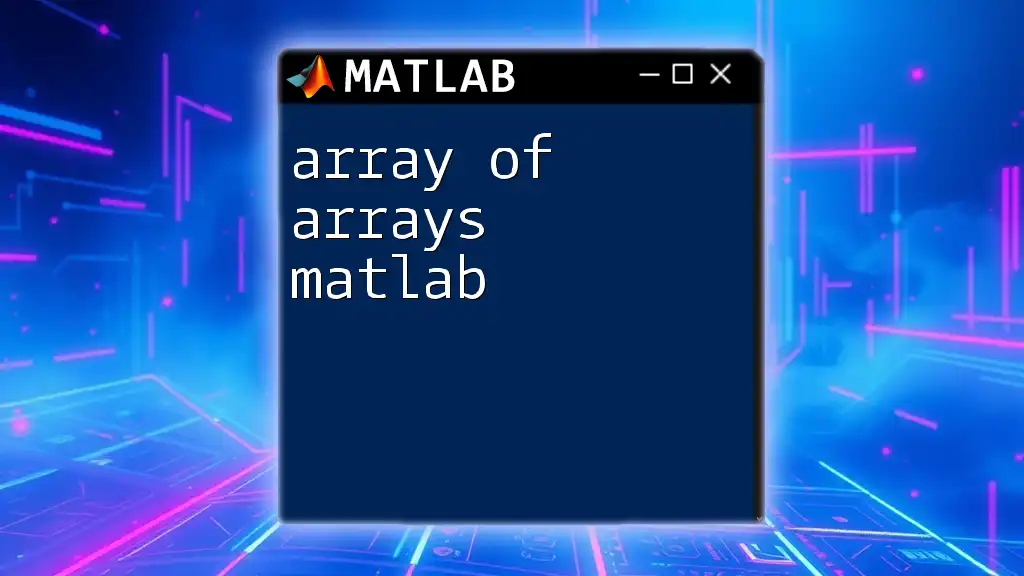
Customizing Your Bar Graph with Error Bars
Changing Bar Colors and Styles
MATLAB allows for extensive customization of graphical elements. You can modify bar colors and styles easily:
bar_handle = bar(data, 'FaceColor', 'flat');
bar_handle.CData(1,:) = [0 0.5 1]; % Specify color for first bar
This code sets the color of the first bar to a custom blue tone. You can tweak `CData` for each bar as needed to enhance visual distinction among categories.
Customizing Error Bars
Just as you can customize the appearance of the bars, you can modify the properties of error bars as well. Here's how to change the color and style:
errorbar(bar_handle.XData, data, errors, 'Color', 'r', 'LineStyle', 'none', 'LineWidth', 1.5);
In this example, the error bars are set to red with a specified line width for increased visibility. This can help your errors stand out and be easily spotted by viewers.
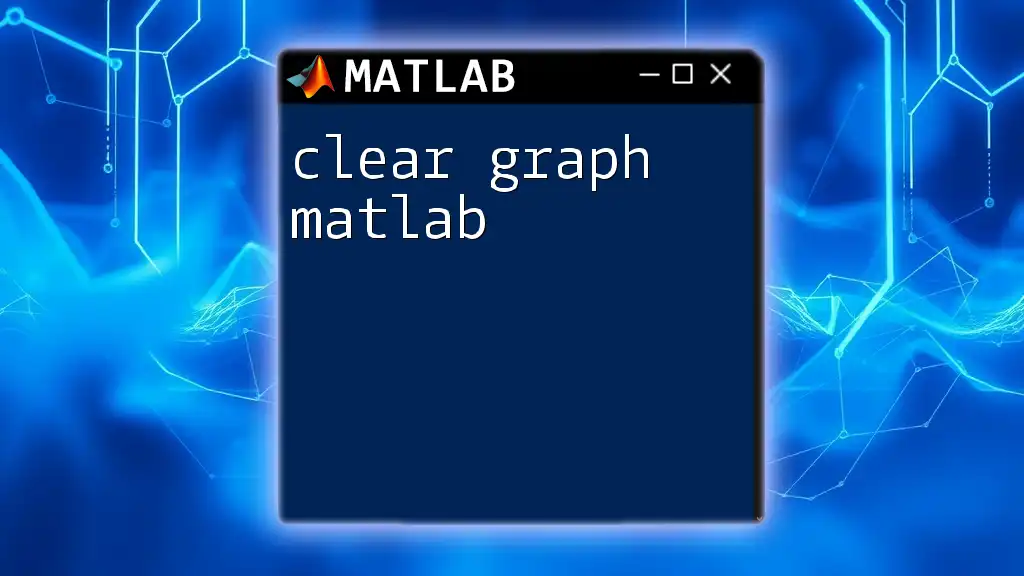
Example Use Case
Real World Example: Analyzing Experimental Data
Imagine you're conducting an experiment to measure the growth of three different plant species under controlled conditions. Your collected data might look like this:
% Example data
categories = {'A', 'B', 'C'};
data = [50, 75, 100];
errors = [5, 10, 15];
In this case, `data` represents the average heights of plants across three categories, while `errors` indicates the error margins.
% Create the bar graph
figure;
bar(data);
hold on;
% Add error bars
errorbar(1:length(data), data, errors, 'k', 'linestyle', 'none');
% Customizing the graph
title('Experimental Data with Error Bars');
set(gca, 'XTickLabel', categories);
xlabel('Category');
ylabel('Measurements');
hold off;
This complete example creates a bar graph representing plant growth along with error bars that indicate measurement variability. The graph’s title and axis labels aid in clarifying the content that represents your experimental results.
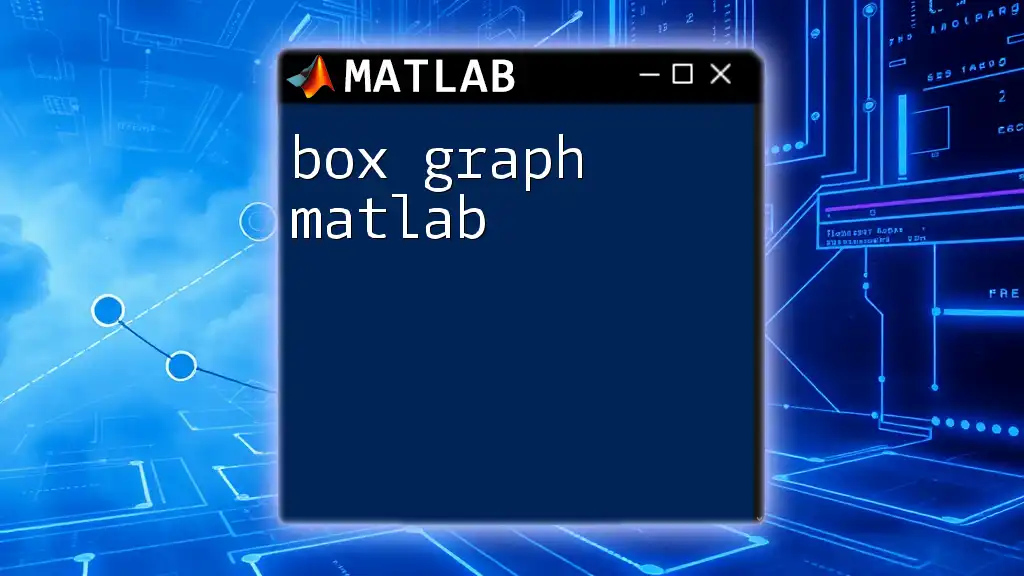
Conclusion
Incorporating error bars in your bar graphs using MATLAB not only enhances data visualization but also enriches the narrative behind the data. Understanding how to implement and customize these graphs empowers you to make informed recommendations and insights in your analyses.
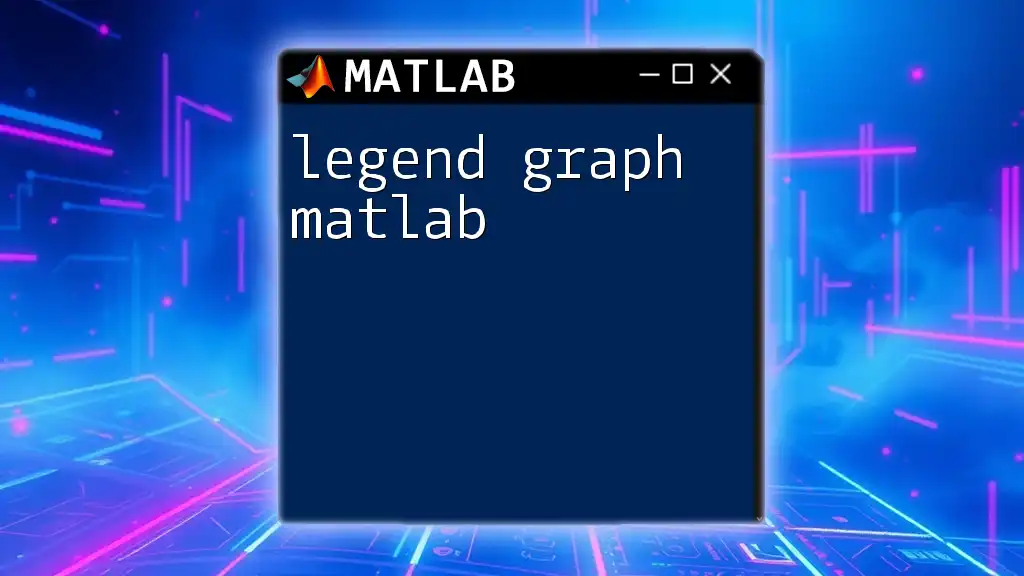
Additional Resources
For those who wish to explore more about MATLAB graphing features, the official MATLAB documentation offers exhaustive information. Additionally, pursuing structured learning through available MATLAB courses can deepen your understanding.
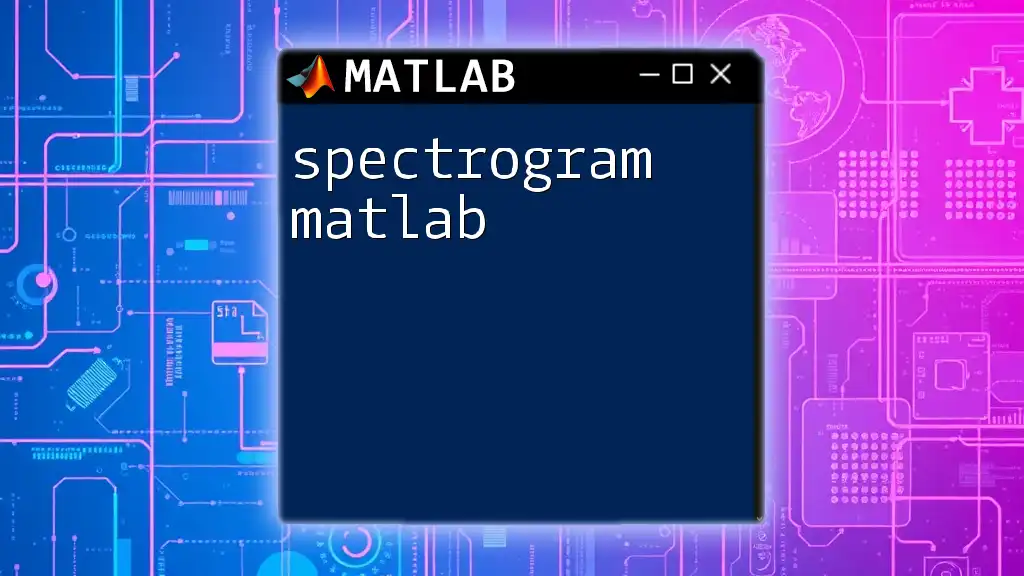
Call to Action
If you want to master error bars and bar graphs and elevate your data visualization skills, consider subscribing to our platform for concise MATLAB tutorials. We're here to help you on your journey with free consultations and demos!