The Gauss-Seidel method is an iterative technique used to solve systems of linear equations, which can be effectively implemented in MATLAB with the following code snippet:
function x = gaussSeidel(A, b, x0, tol, maxIter)
n = length(b);
x = x0;
for k = 1:maxIter
x_old = x;
for i = 1:n
sum1 = A(i, 1:i-1) * x(1:i-1);
sum2 = A(i, i+1:n) * x_old(i+1:n);
x(i) = (b(i) - sum1 - sum2) / A(i, i);
end
if norm(x - x_old, inf) < tol
break;
end
end
end
Understanding the Gauss-Seidel Method
What is the Gauss-Seidel Method?
The Gauss-Seidel method is an iterative technique used to solve systems of linear equations. This method is especially useful when dealing with large systems where direct methods may be computationally expensive. Unlike the Jacobi method, which updates all variables independently, the Gauss-Seidel method updates each variable sequentially, using the most recently updated values. By doing so, the method can converge faster under certain conditions.
In mathematical terms, the Gauss-Seidel method can be represented as:
\[ x_i^{(k+1)} = \frac{b_i - \sum_{j=1}^{i-1} a_{ij} x_j^{(k+1)} - \sum_{j=i+1}^n a_{ij} x_j^{(k)}}{a_{ii}} \]
where:
- \( x_i^{(k+1)} \) is the updated value of the variable.
- \( b_i \) is the entry from the right-hand side vector.
- \( a_{ij} \) are the coefficients from the coefficient matrix.
Key terms to remember include convergence (the process of approaching a limit), iteration (the repetition of a process), and the system of linear equations — a set of equations where each equation is linear.
Application of the Gauss-Seidel Method
The Gauss-Seidel method is widely used in engineering fields, especially in structural analysis, fluid mechanics, and thermal systems. Some advantages of using this method include its simplicity and efficiency when consistent convergence conditions are met. It is especially effective for sparse matrices and large systems where computational load is critical.
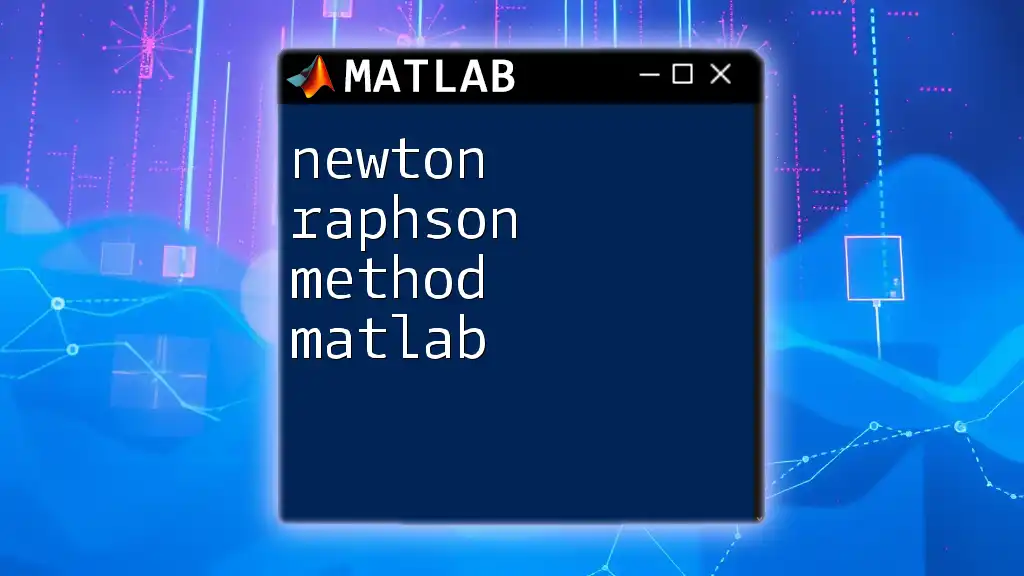
Setting Up MATLAB for the Gauss-Seidel Method
Installation and Environment Setup
To begin, ensure you have MATLAB installed on your system. You can download it from the MathWorks website. After installation, launch MATLAB to access its features. Familiarize yourself with the workspace layout, including the command window for direct commands, the script editor for coding, and the variable workspace for managing your data.
Creating a MATLAB Script
Creating a new script in MATLAB is straightforward.
- Open the script editor from the toolbar or by typing `edit` in the command window.
- You can start coding immediately, as MATLAB reads the script line by line.
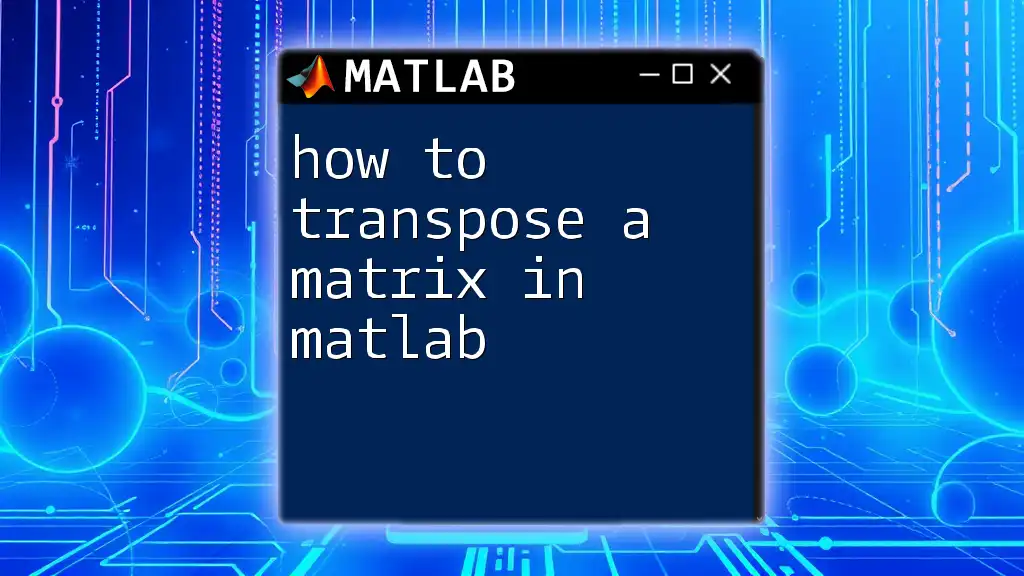
Coding the Gauss-Seidel Algorithm in MATLAB
Defining the System of Equations
To implement the Gauss-Seidel method, you first need to represent your system of equations in matrix form. For example, consider the following equations:
- \( 4x + y + z = 12 \)
- \( x + 3y + 2z = 10 \)
- \( 2x + y + 3z = 14 \)
These equations can be expressed in matrix format as:
Coefficient Matrix (A): \[ A = \begin{bmatrix} 4 & 1 & 1 \\ 1 & 3 & 2 \\ 2 & 1 & 3 \end{bmatrix} \]
Right-Hand Side Vector (b): \[ b = \begin{bmatrix} 12 \\ 10 \\ 14 \end{bmatrix} \]
Implementing the Algorithm
Now, we can implement the Gauss-Seidel algorithm in MATLAB. The algorithm involves several key steps:
- Initialize the variables.
- Define the maximum number of iterations and a tolerance level to check convergence.
- Update the values of variables iteratively.
Here’s a basic implementation:
A = [4, 1, 1; 1, 3, 2; 2, 1, 3]; % Coefficient matrix
b = [12; 10; 14]; % Right-hand side
x = zeros(size(b)); % Initial guess
max_iterations = 100; % Maximum number of iterations
tolerance = 1e-6; % Tolerance level
for k = 1:max_iterations
x_old = x; % Store previous iteration values
for i = 1:length(b)
x(i) = (b(i) - A(i, [1:i-1, i+1:end]) * x_old([1:i-1, i+1:end])) / A(i,i);
end
% Convergence check
if norm(x - x_old, inf) < tolerance
break; % Exit if the solution converges
end
end
Additional Features
Convergence Criteria
Convergence is crucial in the Gauss-Seidel method. The algorithm will terminate either when it reaches the maximum number of iterations or when the difference between consecutive approximations is less than the predefined tolerance level. Adjusting this tolerance allows you to balance precision and performance according to your needs.
Stopping Conditions
In addition to maximum iterations and tolerance levels, you might want to implement custom stopping conditions, such as specific limits for each variable or applying a different norm. Consider what kind of results you are looking for, as these conditions can impact the efficiency and reliability of the results.
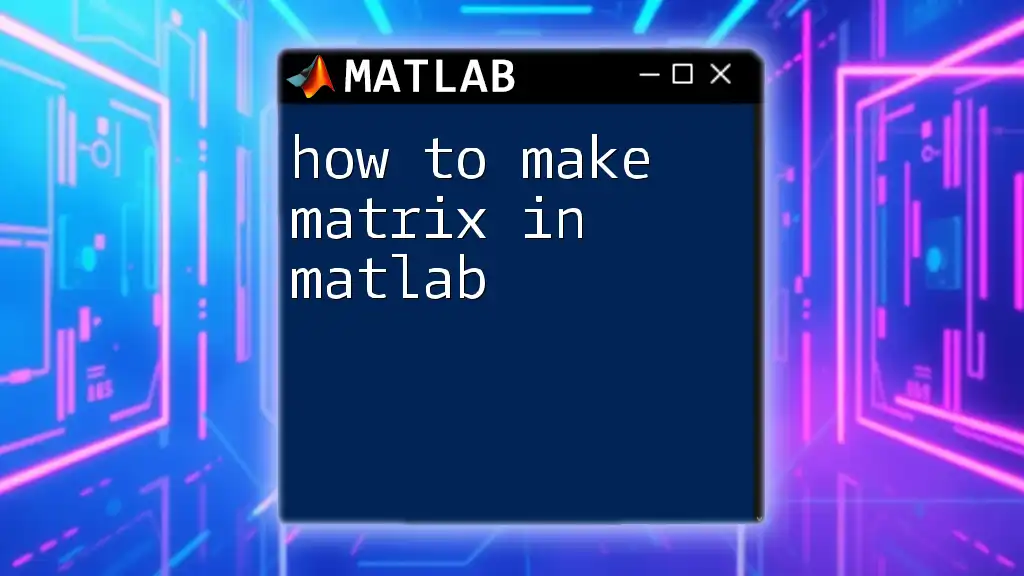
Testing and Validating the Implementation
Running the MATLAB Script
To run your script, simply press the "Run" button in the script editor or type the script name in the command window. If you encounter errors, check for syntax mistakes, such as mismatched dimensions in matrix operations or improper indexing.
Analyzing Results
Once the script runs successfully, you will see the final values of \( x \), \( y \), and \( z \) in the command window, representing the solution to your original set of equations.
For example, if the output yields:
x =
1
2
3
This indicates that \( x = 1 \), \( y = 2 \), and \( z = 3 \) is the solution to the equations in the system.
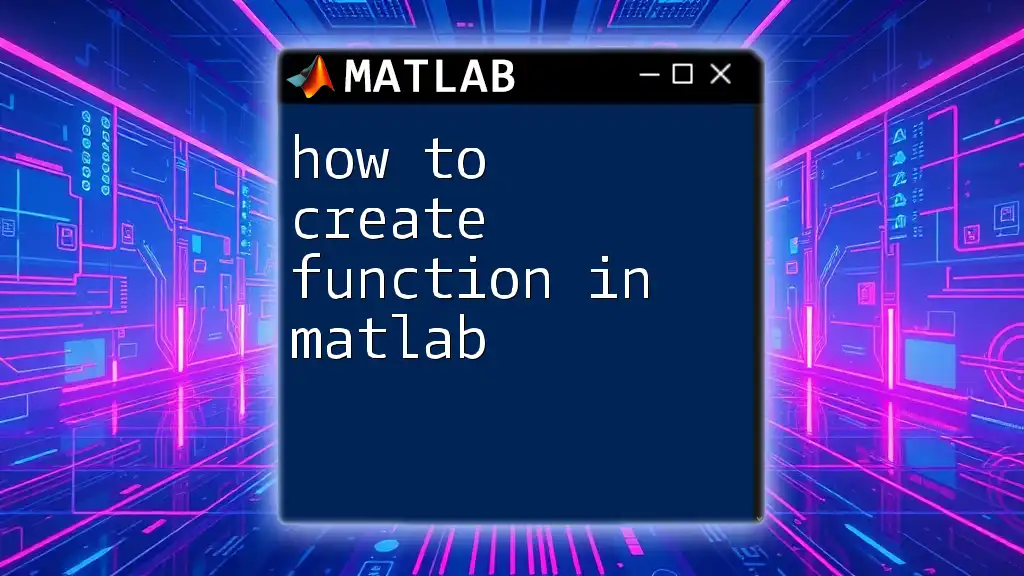
Conclusion
In this guide, we discussed how to code the Gauss-Seidel method in MATLAB, offering insights into its implementation and application. By mastering such numerical techniques, you will enhance your problem-solving skills in engineering and computational mathematics. I encourage you to explore various systems of equations and modify the algorithm to further deepen your understanding.
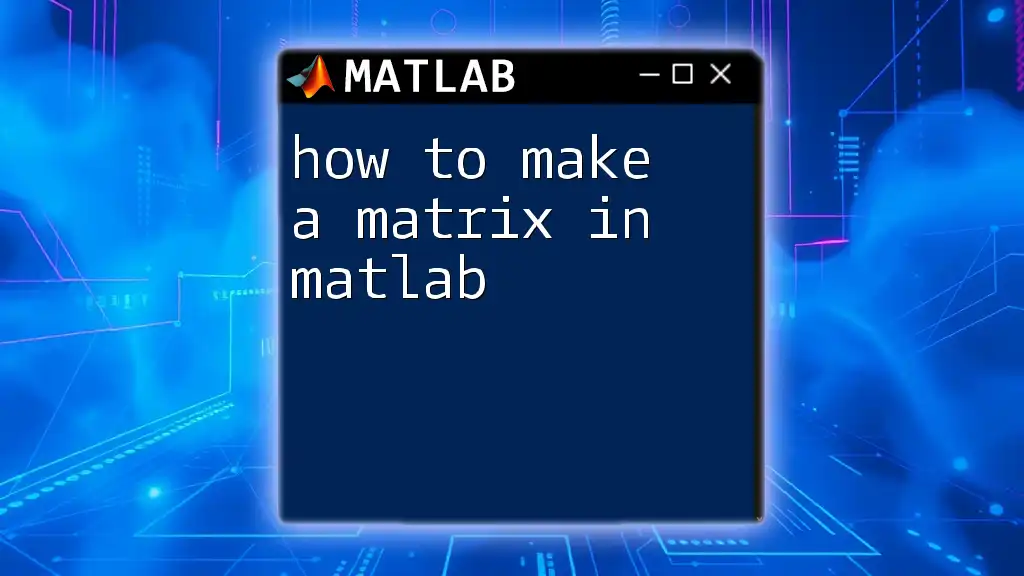
Additional Resources
Further Reading
For those looking to dive deeper, consider exploring textbooks on numerical analysis or online resources that elaborate more on linear algebra topics and iterative solutions.
Exercises
Practice by solving different systems of equations using the Gauss-Seidel method. Experiment with larger matrices or adjust parameters to observe how the convergence changes.
Community and Forums
Engage with MATLAB communities and forums for support and to share ideas. Sites like MathWorks’ own user community or platforms like Stack Overflow can be invaluable for troubleshooting and learning.