In MATLAB, the line of best fit can be determined using linear regression with the `polyfit` function to achieve a linear approximation of data points. Here's a simple example of how to do it:
% Example data points
x = [1, 2, 3, 4, 5];
y = [2.2, 2.8, 3.6, 4.5, 5.1];
% Calculate the coefficients of the line of best fit
p = polyfit(x, y, 1);
% Generate the line of best fit
yfit = polyval(p, x);
% Plot the data and the line of best fit
scatter(x, y); % Original data points
hold on;
plot(x, yfit, 'r-'); % Line of best fit
hold off;
title('Line of Best Fit');
xlabel('X-axis');
ylabel('Y-axis');
legend('Data Points', 'Line of Best Fit');
Understanding the Concept of Linear Regression
What is Linear Regression?
Linear regression is a powerful statistical tool that analyzes the relationship between two or more variables by fitting a linear equation to observed data. Essentially, it helps us understand how changes in one variable (the independent variable) are related to changes in another (the dependent variable). The line of best fit, often derived from linear regression, represents the best approximation of this relationship in a scatter plot, minimizing the distance between the observed data points and the line itself.
Applications of Linear Regression
Linear regression is widely applicable across various fields:
- Economics: Analyzing trends and forecasting financial data, such as sales and revenue.
- Engineering: Modeling relationships between variables in systems for design and optimization.
- Healthcare: Establishing the relationship between lifestyle factors and health outcomes.
These applications demonstrate how crucial the line of best fit in MATLAB can be, allowing practitioners to make informed decisions based on data analysis.
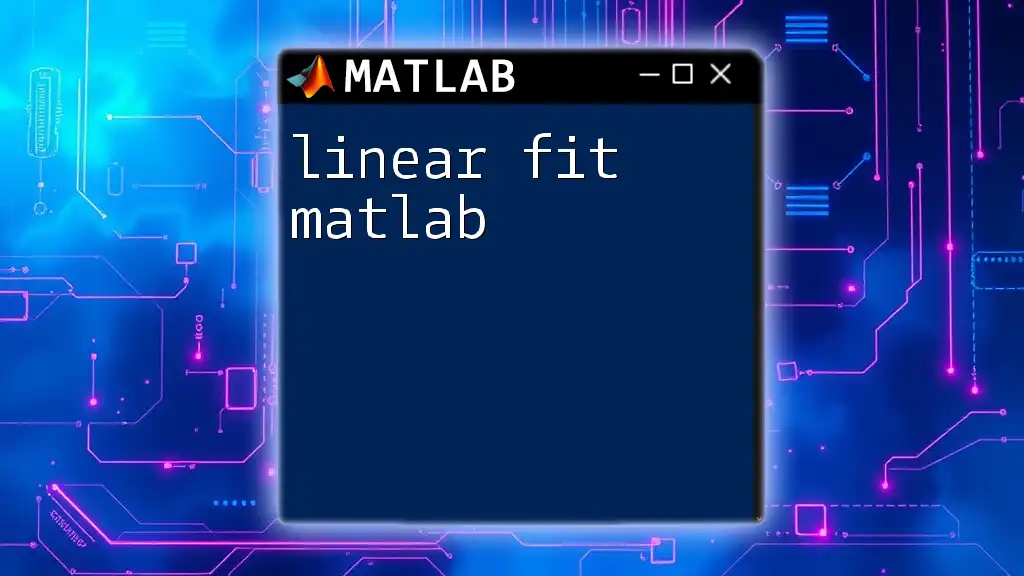
Getting Started with MATLAB
MATLAB Overview
MATLAB, short for "Matrix Laboratory," is a high-performance programming environment used primarily for numerical computing. Its versatility in data analysis, visualization, and algorithm development makes it an essential tool for anyone working with data.
Setting Up Your Environment
Before diving into the specifics of the line of best fit in MATLAB, ensure you have MATLAB installed, preferably with the Statistics and Machine Learning Toolbox, as it provides additional tools and functions for data analysis. Once installed, open MATLAB to start coding.
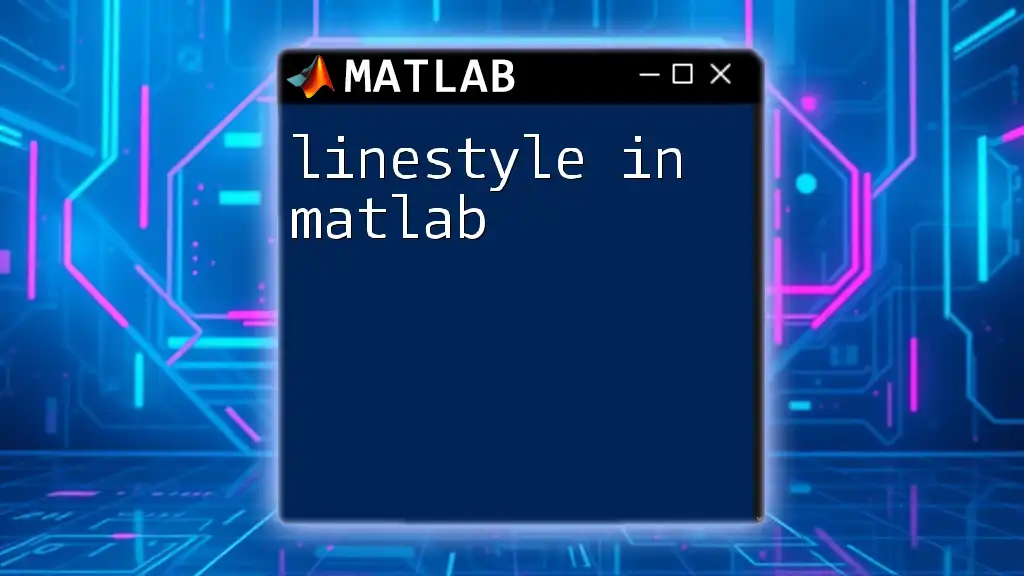
Importing Data into MATLAB
Loading Data
Before you can analyze your data, you'll need to import it into MATLAB. This can be achieved using a variety of functions, such as `load()` for simple datasets or `readtable()` for structured data, such as CSV files.
For example, if you have a data file named `data.csv`, you can load it as follows:
data = readtable('data.csv');
Exploring Data
After loading your data, it is crucial to inspect it to ensure it’s formatted correctly and to understand its structure. MATLAB provides several functions for this, including:
- `head(data)`: Displays the first few rows of the dataset.
- `summary(data)`: Provides a summary, including mean, standard deviation, and other statistics.
- `display(data)`: Displays the entire dataset in the command window.
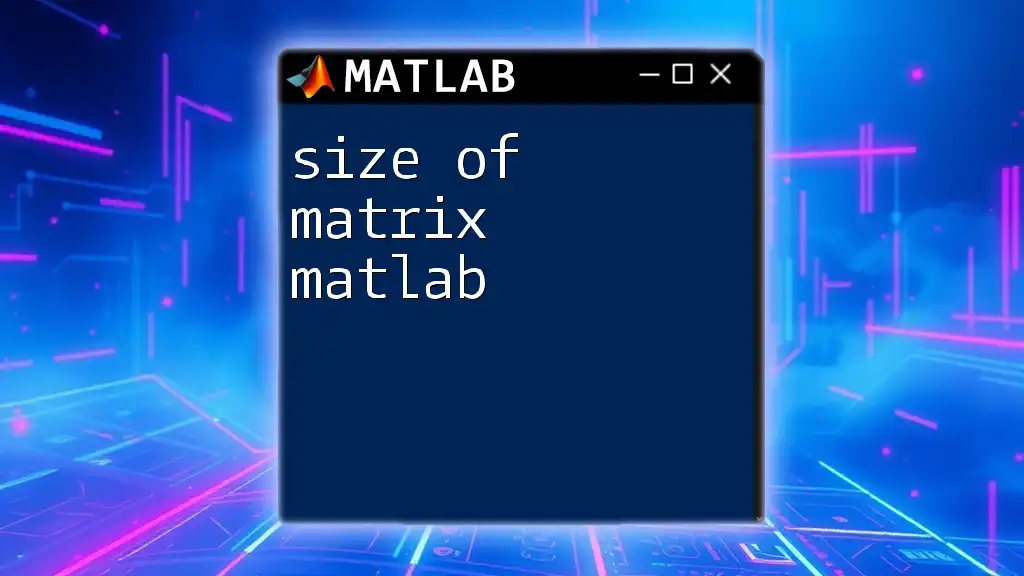
Visualizing Data
Plotting Data
Before fitting a line of best fit, visualizing your data helps identify patterns, trends, or potential outliers. A scatter plot is a typical method for visualizing the relationship between two quantitative variables.
To create a scatter plot in MATLAB, you can use the following code:
scatter(data.X, data.Y);
title('Scatter Plot of Data');
xlabel('X-axis');
ylabel('Y-axis');
This visualization prepares you for the next steps in determining the line of best fit.
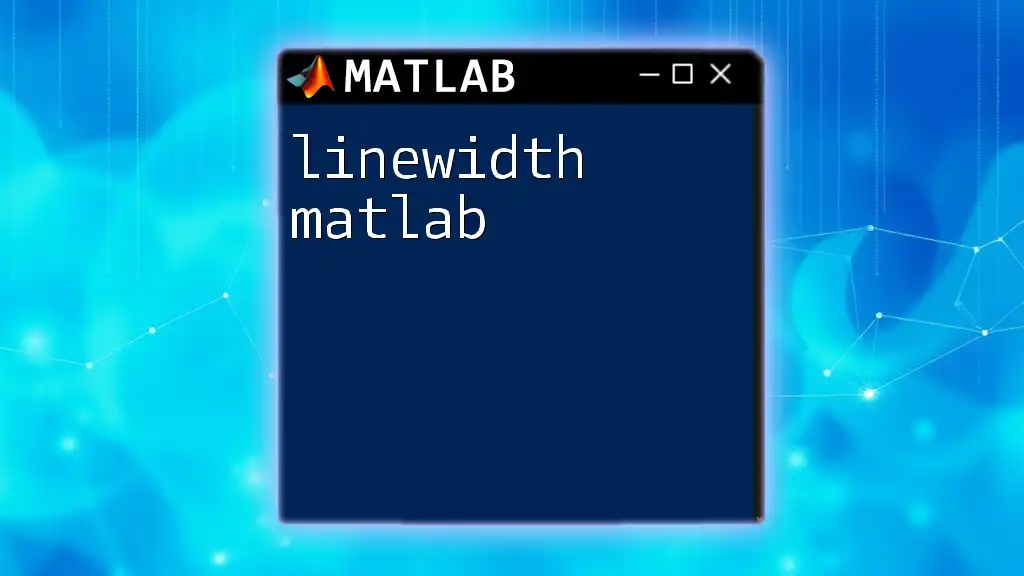
Calculating the Line of Best Fit in MATLAB
Using MATLAB Functions
MATLAB simplifies the process of fitting a line of best fit through built-in functions like `polyfit()` and `polyval()`. These functions facilitate the computation of polynomial coefficients and the evaluation of the polynomial.
Code Example: Simple Linear Regression
To calculate the line of best fit for a dataset in MATLAB, consider the following example:
% Example Data
x = [1, 2, 3, 4, 5];
y = [2, 3, 5, 7, 11];
% Calculating coefficients
p = polyfit(x, y, 1);
% Generating line of best fit
yfit = polyval(p, x);
% Plotting
scatter(x, y);
hold on;
plot(x, yfit, '-r');
title('Line of Best Fit');
xlabel('X-axis');
ylabel('Y-axis');
legend('Data Points', 'Line of Best Fit');
hold off;
Explanation of the Code
- Data Definition: The `x` and `y` vectors contain the coordinates of your data points.
- Coefficient Calculation: `polyfit(x, y, 1)` computes the coefficients for the linear regression line (1 denotes a linear fit).
- Line Generation: `polyval(p, x)` uses the coefficients `p` to calculate the fitted `y` values.
- Plotting the Results: A scatter plot shows the original data points, while `plot(x, yfit, '-r')` overlays the line of best fit in red.
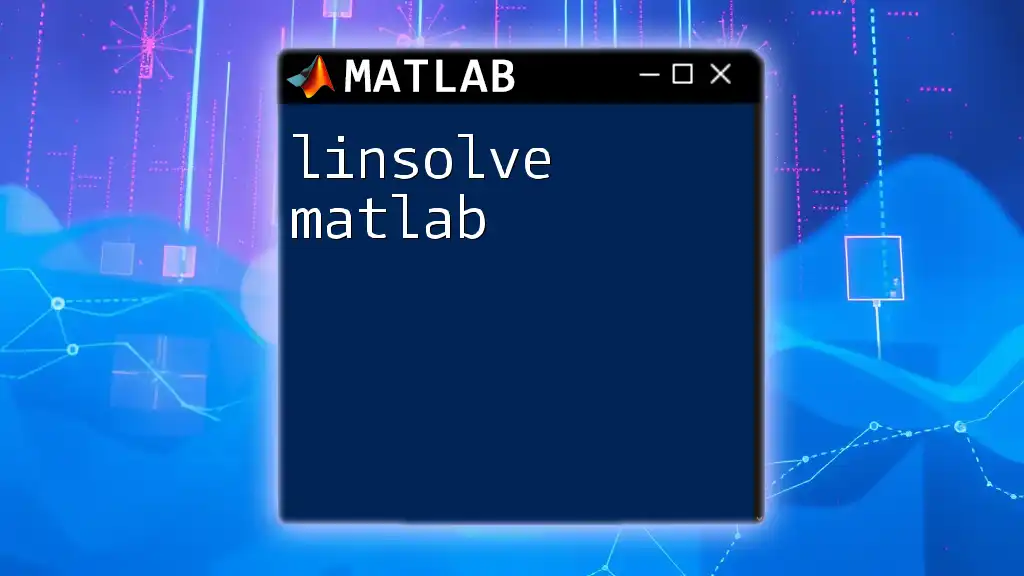
Evaluating the Line of Best Fit
Goodness of Fit Metrics
To understand the effectiveness of the line of best fit, several metrics can be calculated. Two critical metrics are:
- R-squared: Indicates how well the independent variable explains the variability of the dependent variable, with values closer to 1 signifying a better fit.
- Root Mean Square Error (RMSE): Measures the average magnitude of the error between predicted and observed values.
Code Example: Calculating R-squared
You can calculate the R-squared value in MATLAB using the following code:
% Calculate R-squared
SS_res = sum((y - yfit).^2);
SS_tot = sum((y - mean(y)).^2);
R2 = 1 - (SS_res / SS_tot);
disp(['R-squared: ', num2str(R2)]);
This gives a quick quantifiable measure of how well your line fits the data.
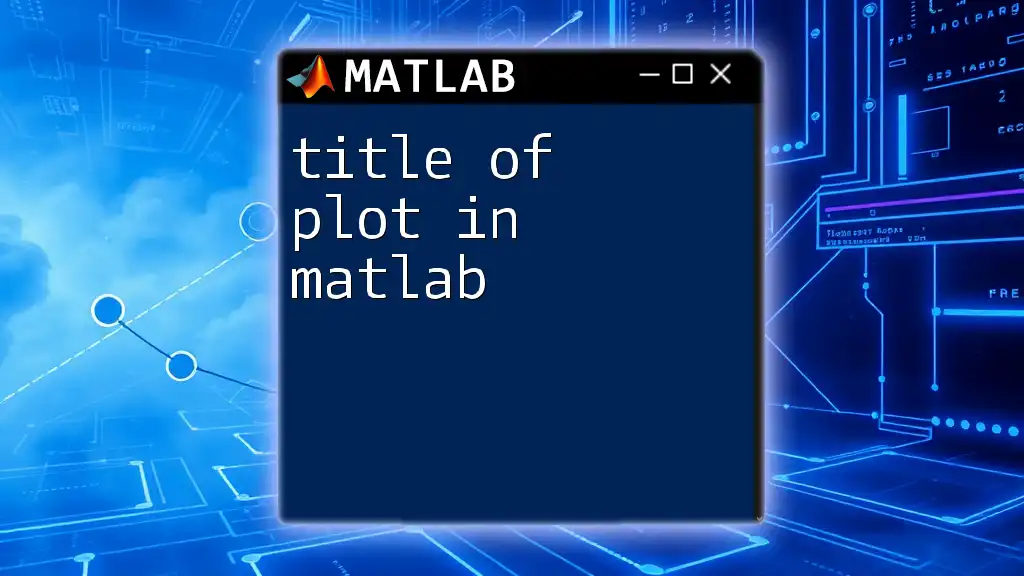
Advanced Techniques
Polynomial Regression
When your data does not fit well with a straight line, polynomial regression allows you to fit a polynomial equation of degree greater than one. To implement polynomial regression, you can adjust the degree in the `polyfit()` function.
% Polynomial Regression of Degree 2
p2 = polyfit(x, y, 2);
yfit2 = polyval(p2, x);
% Plotting
scatter(x, y);
hold on;
plot(x, yfit2, '-g'); % Green line for polynomial fit
title('Polynomial Line of Best Fit');
xlabel('X-axis');
ylabel('Y-axis');
legend('Data Points', 'Polynomial Fit');
hold off;
Multivariable Linear Regression
For cases with multiple predictors, MATLAB’s `regress()` function enables conducting multivariable linear regression. The independent variables must be combined into a single matrix alongside the dependent variable.
% Multivariable Regression Example
X = [ones(size(data.X)), data.X1, data.X2]; % Adding a column of ones for the intercept
y = data.Y;
% Regression
b = regress(y, X);
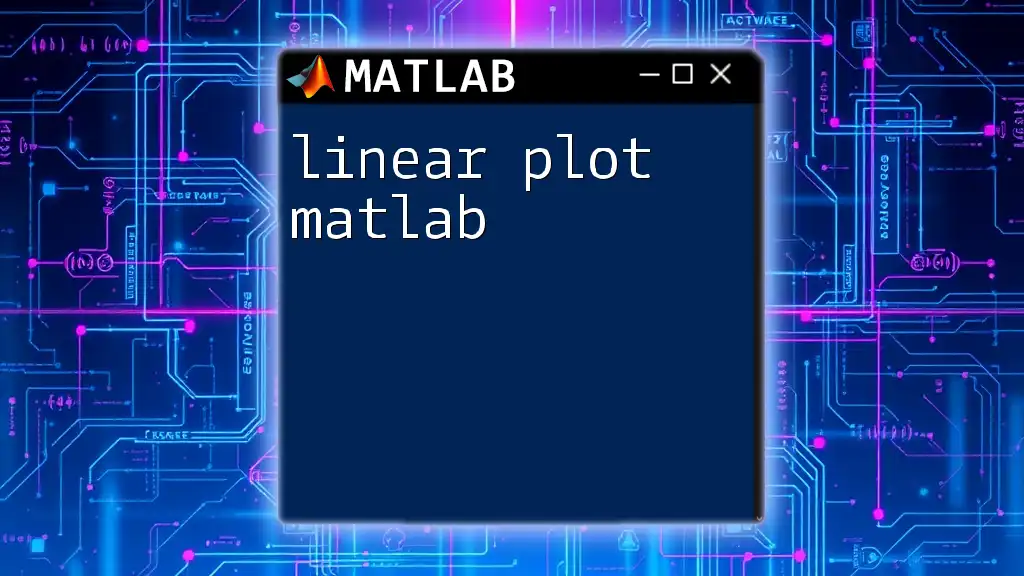
Common Challenges and Solutions
Overfitting vs. Underfitting
One of the key challenges in regression analysis is balancing overfitting (creating a model that's too complex and captures noise rather than the underlying trend) and underfitting (failing to capture the inherent trend). Techniques like cross-validation can help evaluate model performance and avoid excessive complexity.
Handling Outliers
Outliers can heavily skew results in regression analysis. Identifying and managing outliers is essential for precise modeling. Techniques include visual inspection through plots or using statistical measures (e.g., Z-scores).
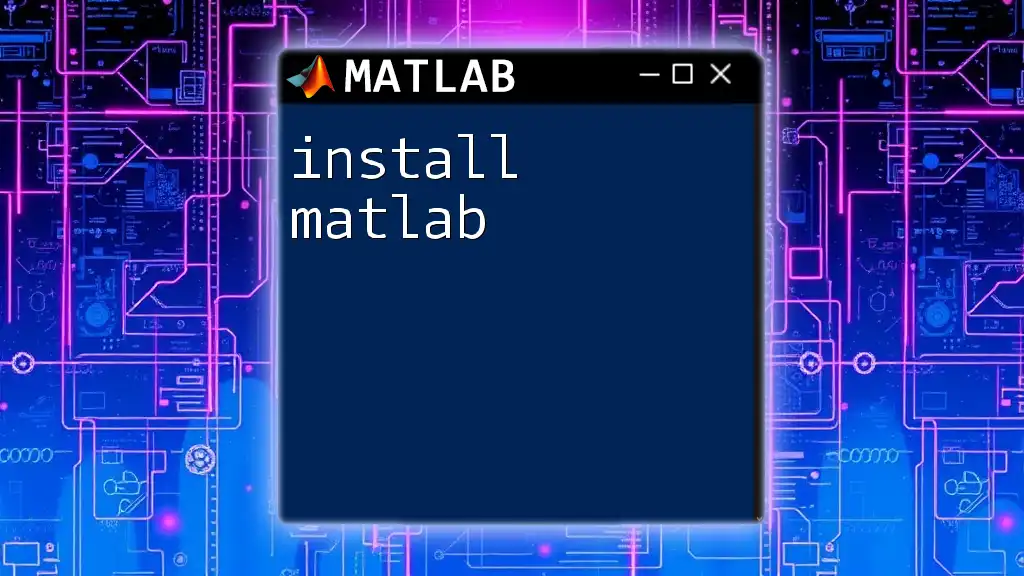
Conclusion
Utilizing the line of best fit in MATLAB is invaluable for statistical analysis and data interpretation. Understanding how to compute, evaluate, and refine your models empowers you to extract meaningful insights from your data. As you become more proficient in MATLAB, you will uncover additional tools and methods to further enhance your analytical skills.
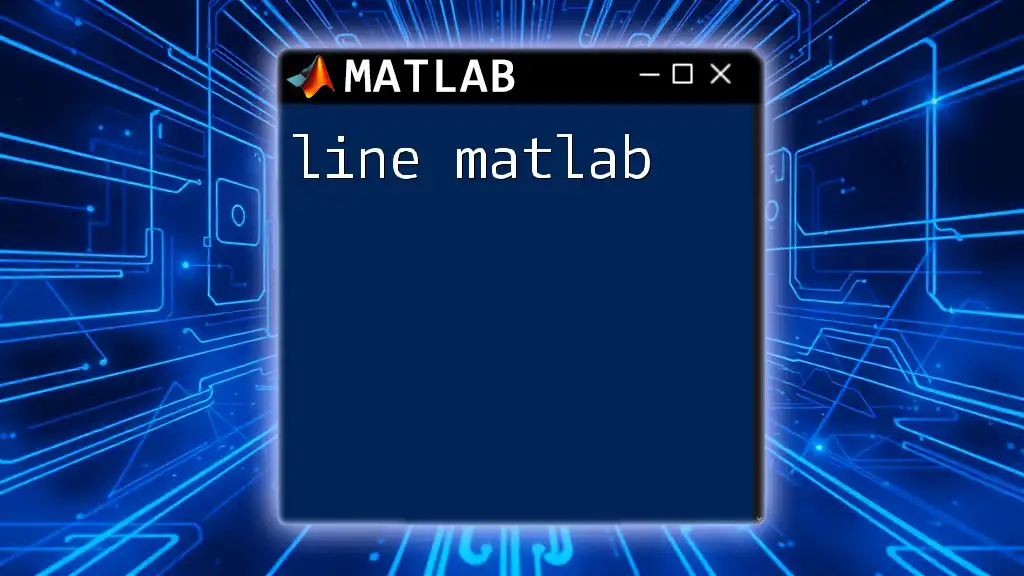
FAQs
What is the difference between a line of best fit and a trend line?
The terms are often used interchangeably; however, a line of best fit specifically refers to a line that minimizes the residuals between the observed values and the values predicted by the model.
Can I use a line of best fit for non-linear data?
Yes, a line of best fit can be used for non-linear data by employing polynomial regression or other types of regression that accommodate more complex curves.
How do I interpret the coefficients from a linear regression in MATLAB?
The coefficients give the slope and intercept of the regression line. The slope indicates the change in the dependent variable for a unit change in the independent variable, while the intercept represents the expected value of the dependent variable when the independent variable equals zero.
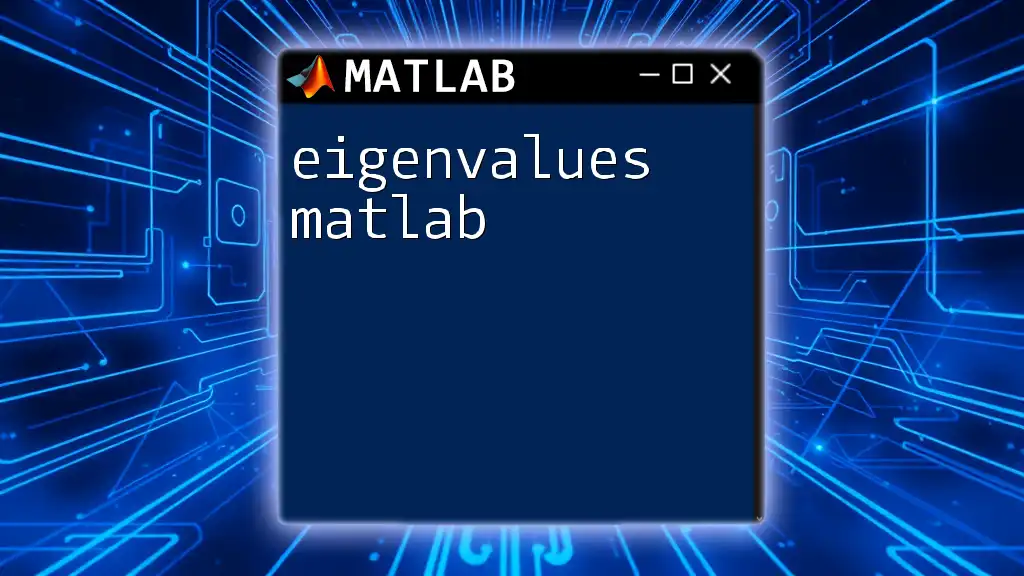
Call to Action
If you’re eager to deepen your understanding of MATLAB and enhance your data analysis skills, consider joining our training program. We provide comprehensive lessons tailored to help you master MATLAB commands efficiently!