A best fit line in MATLAB is determined using linear regression, which minimizes the difference between the observed data points and the line itself, allowing for a clearer understanding of the relationship between variables.
Here’s a code snippet to calculate and plot the best fit line:
% Sample data
x = [1, 2, 3, 4, 5];
y = [2.2, 2.8, 3.6, 4.5, 5.1];
% Calculate the best fit line using polyfit
p = polyfit(x, y, 1);
% Generate values for the best fit line
y_fit = polyval(p, x);
% Plot the data and the best fit line
figure;
scatter(x, y, 'filled'); % Original data
hold on;
plot(x, y_fit, 'r-'); % Best fit line
xlabel('X-axis');
ylabel('Y-axis');
title('Best Fit Line');
legend('Data points', 'Best fit line');
hold off;
Understanding the Basics
What is a Best Fit Line?
A best fit line (or trend line) is a straight line that best represents the data on a scatter plot. It is the result of applying a linear regression model to a set of data points, aiming to minimize the distance between the points and the line itself. The most commonly used method to estimate the best fit line is the least squares method, which minimizes the sum of the squares of the vertical distances of the points from the line.
Why Use a Best Fit Line?
Using a best fit line is pivotal in data analysis for several reasons:
- Summarizes Data: It provides a simple representation of complex data, making it easier to interpret trends.
- Predictive Analysis: A best fit line can predict values beyond the observed data points by extrapolating the relationship.
- Visual Insight: It allows quick visual insight into how two variables relate to each other, whether positively or negatively.

Getting Started with MATLAB
Setting Up MATLAB
To analyze and visualize data effectively, the first step is to set up MATLAB. Download the installation from the official MATLAB website and follow the setup instructions to install it on your computer. Once installed, familiarize yourself with the MATLAB interface, including the command window, script editor, and workspace.
Basic MATLAB Commands
Before diving into specific tasks, it's beneficial to know a few key commands to manipulate data in MATLAB. Here are some fundamental commands:
- `load` and `readtable`: For importing data into the MATLAB workspace.
- `plot`: For creating basic plots.
- `title`, `xlabel`, and `ylabel`: For adding titles and labels to your graphs.
These commands set the foundation for more advanced techniques in the MATLAB environment.
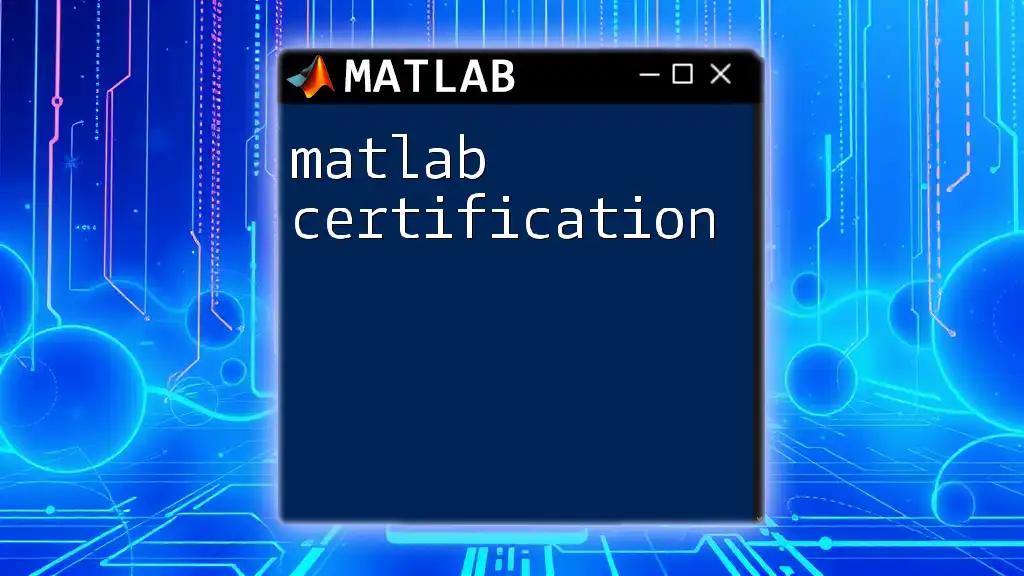
Creating a Best Fit Line in MATLAB
Step 1: Importing Data
The first step in creating a best fit line is importing the data you'll analyze. Data can be imported in various formats. For example, if you have a dataset stored in a `.mat` file or a CSV file, you can load it into your workspace using:
% Load a MAT file
load('datafile.mat');
% Load a CSV file
data = readtable('datafile.csv');
Step 2: Plotting the Data
Once the data is loaded, it's crucial to visualize it. Using the `scatter` function allows you to create a scatter plot, which serves as a visual baseline for your best fit line.
% Example of plotting data points
x = [1, 2, 3, 4, 5];
y = [2, 3, 5, 7, 11];
scatter(x, y, 'filled');
title('Data Points');
xlabel('X-axis');
ylabel('Y-axis');
This plot allows you to see the distribution of your data visually.
Step 3: Calculating the Best Fit Line
Using Polyfit
To calculate the best fit line, you will employ the `polyfit` function. This function fits a polynomial of a specified degree to your data. For a linear fit, you’ll use a first-degree polynomial:
% Best fit line calculation
p = polyfit(x, y, 1); % '1' for a linear fit (first-degree polynomial)
Here, `p` will return the coefficients of the linear equation in the form y = mx + b, where m is the slope and b is the y-intercept.
Using the Linear Model Function
Alternatively, MATLAB’s `fitlm` function is a powerful way to create a linear regression model:
% Using fitlm for linear model
mdl = fitlm(x', y');
The model object `mdl` gives you comprehensive details about the fit, including coefficients, p-values, and R-squared values.
Step 4: Plotting the Best Fit Line
After calculating the best fit line, you’ll want to visualize it alongside your original data points. You can use the `plot` function to achieve this:
% Generate points for fit line
yfit = polyval(p, x); % Evaluate polynomial at given x
hold on; % Hold current plot
plot(x, yfit, '-r', 'LineWidth', 2); % Plot line in red
hold off;
legend('Data Points', 'Best Fit Line');
This code snippet displays the best fit line in red, instantly highlighting the relationship between your data points and the fitted model.
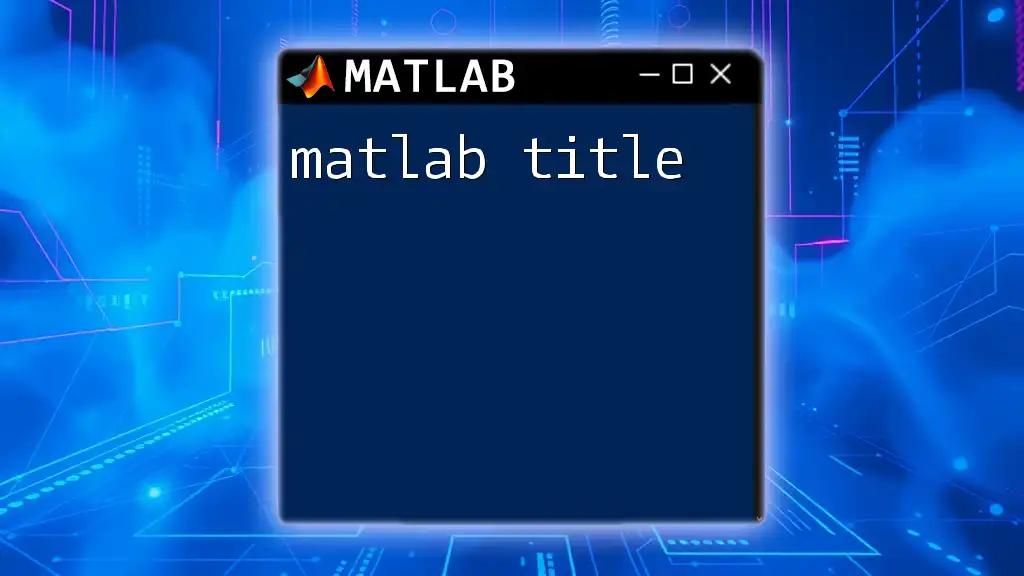
Evaluating the Fit
Interpretation of Results
Once you have the best fit line plotted, it's essential to interpret the results. The coefficients obtained from `polyfit` or `fitlm` inform you about the relationship between the variables. A positive slope indicates a direct relationship, while a negative slope suggests an inverse relationship.
Additionally, you can assess how well the line fits the data using the R-squared value. This value ranges from 0 to 1, with 1 indicating a perfect fit. Higher values generally denote better fits, while low values suggest the model may not adequately represent the data.
Residual Analysis
Examining the residuals (the difference between the observed and predicted values) provides more insight into the model's accuracy. A random scatter of residuals around zero indicates a well-fitted model.
You can visualize the residuals using:
% Calculate and plot residuals
residuals = y - yfit;
figure;
scatter(x, residuals);
title('Residuals');
xlabel('X-axis');
ylabel('Residuals');
A plot of residuals helps identify any patterns suggesting a poor model fit.

Advanced Techniques
Multiple Linear Regression
When you have multiple independent variables, multiple linear regression becomes necessary. Using `fitlm`, you can accommodate additional predictors effectively:
% Example with multiple predictors
x1 = [1, 2, 3, 4, 5];
x2 = [5, 4, 3, 2, 1];
y = [2, 3, 5, 7, 11];
mdl_multi = fitlm([x1', x2'], y');
Polynomial Regression
In instances where data exhibits non-linear relationships, polynomial regression is advantageous. By increasing the degree from 1 to 2 or higher, you can effectively model curves:
p2 = polyfit(x, y, 2); % Quadratic fit
yfit2 = polyval(p2, x);
plot(x, yfit2, '-g', 'LineWidth', 2); % Plot quadratic fit in green
Nonlinear Fits
For more complex relationships, nonlinear fits can be implemented using `nlinfit`. This function is ideal when your data doesn't conform to linear assumptions, permitting a customized fit based on a specified model.
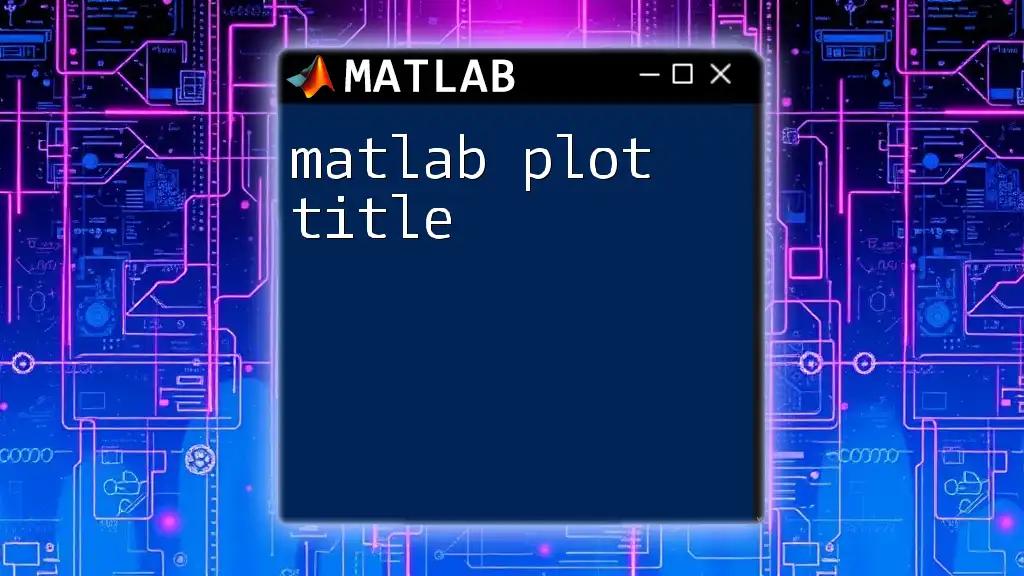
Troubleshooting Common Issues
When dealing with best fit lines, several common issues may arise:
- Data Quality: Ensure your data is clean and free from outliers, which can skew the fit.
- Overfitting or Underfitting: Evaluate the complexity of your model; overfitting occurs when the model is too complex, while underfitting happens with too simple a model.
- Interpretation Errors: Be cautious when interpreting coefficients; correlation does not imply causation.
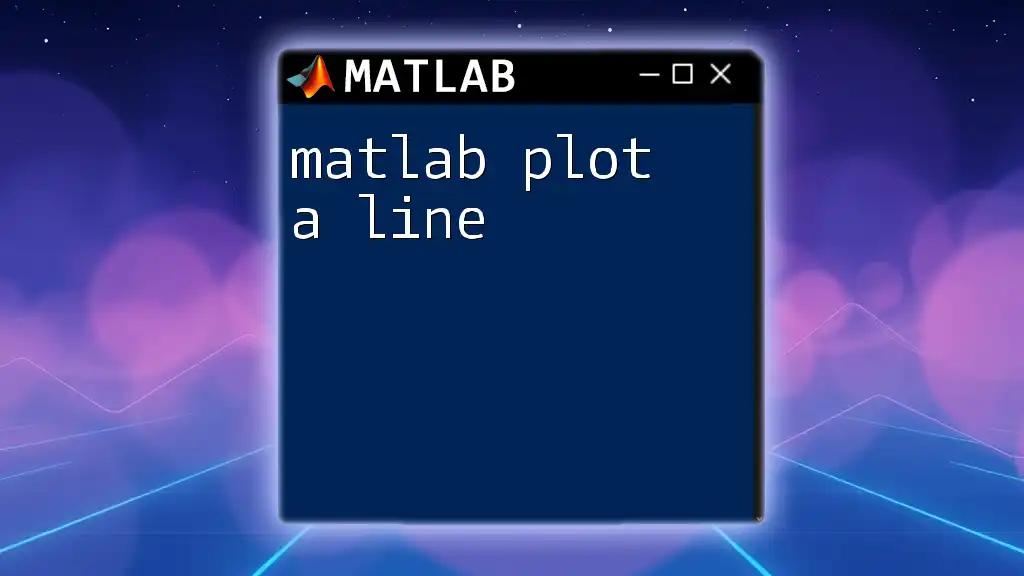
Conclusion
By following the steps outlined in this guide, you can efficiently create, plot, and interpret best fit lines in MATLAB. Understanding how to manipulate and analyze your data through best fit lines opens the door to deeper insights and the ability to make informed predictions. As you progress, practice using different datasets and models to enhance your MATLAB skills.
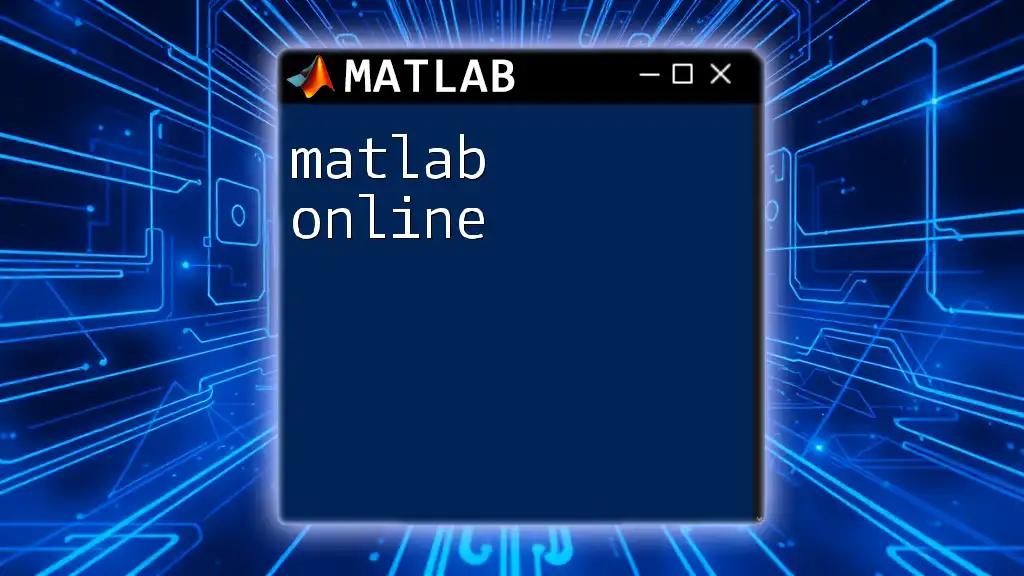
Additional Resources
For further learning, refer to the official MATLAB documentation for detailed explanations of functions and techniques. Consider additional courses that dive more deeply into MATLAB programming and data analysis to continue developing your expertise.