"MATLAB for Dummies" is a beginner-friendly approach to mastering MATLAB through simple commands and practical examples that help demystify its functionality.
Here’s a basic code snippet to illustrate a straightforward operation in MATLAB:
% This code calculates the sum of two numbers
a = 5;
b = 10;
sum = a + b; % Outputs 15
disp(sum);
What is MATLAB?
Definition and Purpose
MATLAB, short for Matrix Laboratory, is a high-level programming language and interactive environment designed primarily for numerical computing and data visualization. It excels in working with matrices, making it an invaluable tool in many fields including engineering, science, finance, and education.
MATLAB allows you to explore numerical data, create algorithms, and develop models with ease. By mastering the fundamentals, you can leverage MATLAB to solve complex problems efficiently.
Key Features of MATLAB
MATLAB is known for its rich feature set that aids both novices and experienced programmers. Some of its key features include:
-
Interactive Environment: Users can enter commands directly into the Command Window and see results immediately. This interactivity simplifies debugging and exploring data.
-
High-Performance Capabilities: MATLAB is optimized for numerical computations. Its built-in functions can handle large datasets and perform complex matrix calculations rapidly.
-
Extensive Mathematical Function Library: MATLAB comes equipped with an extensive library of built-in mathematical functions, enabling users to perform sophisticated operations with minimal code.
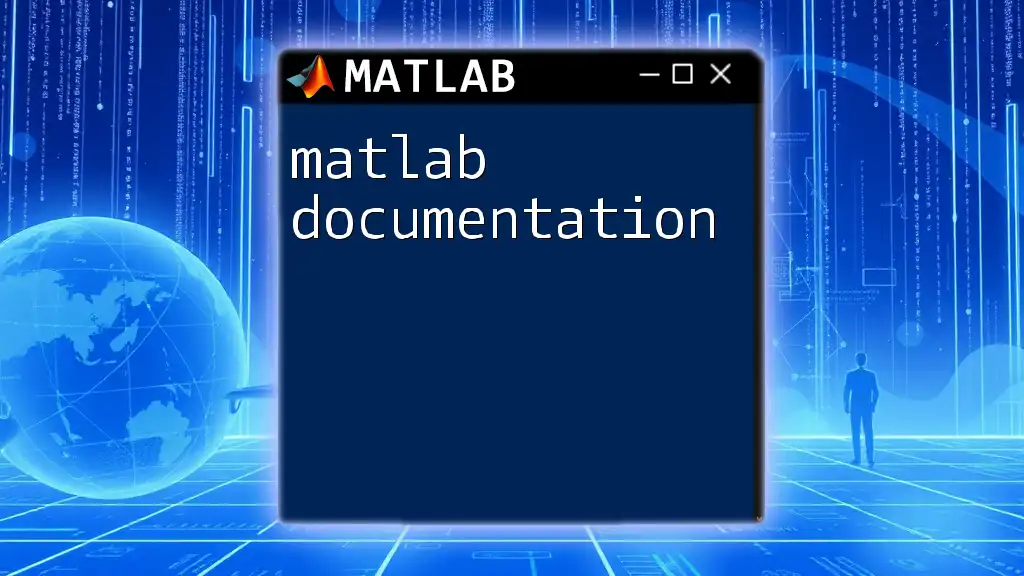
Getting Started with MATLAB
Setting Up MATLAB
To dive into MATLAB, the first step is installation. The process may vary depending on your operating system:
-
Windows: Simply download the installer from the MathWorks website and follow the on-screen instructions.
-
Mac: After downloading, open the file and drag MATLAB to your Applications folder.
-
Linux: Extract the files from the downloaded archive and run the installer from the command line.
Once installed, launch MATLAB and complete the initial setup by following the prompts.
The MATLAB Interface
Understanding the interface is crucial for beginners. When you open MATLAB, you'll encounter several key components:
-
Command Window: This is your primary workspace where you can input commands and receive immediate feedback. It’s particularly useful for testing small pieces of code.
-
Workspace: This area gives you insights into variables currently in use, allowing you to monitor your data effortlessly.
-
Command History: The Command History keeps a log of all commands you’ve executed, making it easy to track your work and repeat commands as needed.
-
Editor: When writing scripts or complex functions, the Editor enables users to compose and save code files for future use. You’ll find features like syntax highlighting that assist in readability.
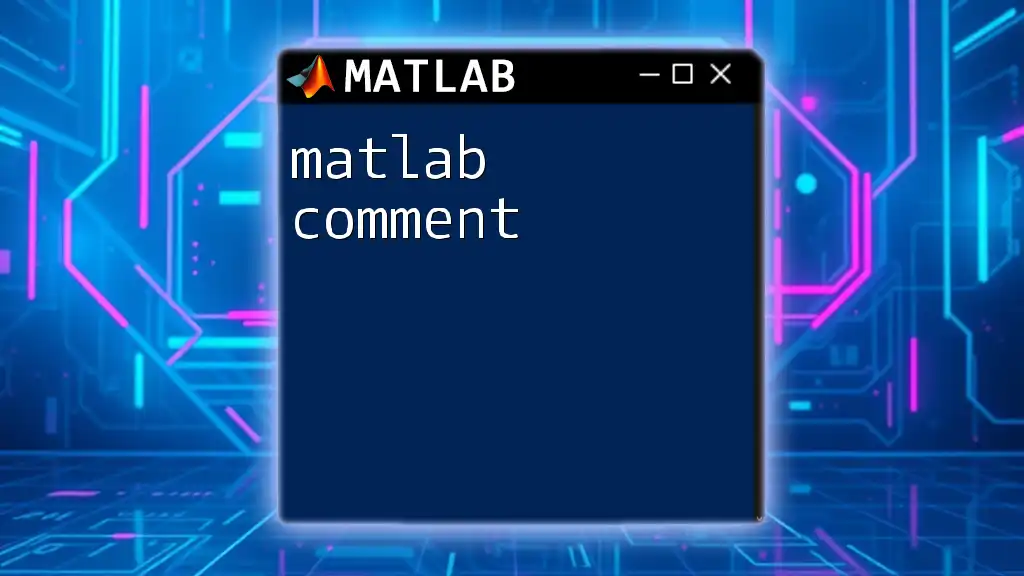
Basic Operations and Functions
Working with Variables
In MATLAB, variables are fundamental to storing and manipulating data. Assigning values to variables is straightforward. For instance:
x = 10; % Assigning 10 to variable x
This command creates a variable `x` with the value of 10. MATLAB supports a variety of data types, including arrays, structures, and cell arrays, providing flexibility in how data can be organized.
Simple Arithmetic Operations
Basic arithmetic operations are intuitive in MATLAB. For instance, addition, subtraction, multiplication, and division can be performed using common operators.
Example of performing basic arithmetic:
a = 5;
b = 2;
sum = a + b; % Example of addition
In this case, variable `sum` will hold the value of 7 after executing the command.
Matrix Operations
MATLAB's strength lies in its native support for matrix operations. Creating matrices and performing calculations are seamless.
For example:
A = [1, 2; 3, 4];
B = [5, 6; 7, 8];
C = A * B; % Matrix multiplication
Here, variable `C` will hold the result of the matrix multiplication of matrices `A` and `B`. You can also perform operations like transposing and inversing matrices easily in MATLAB.
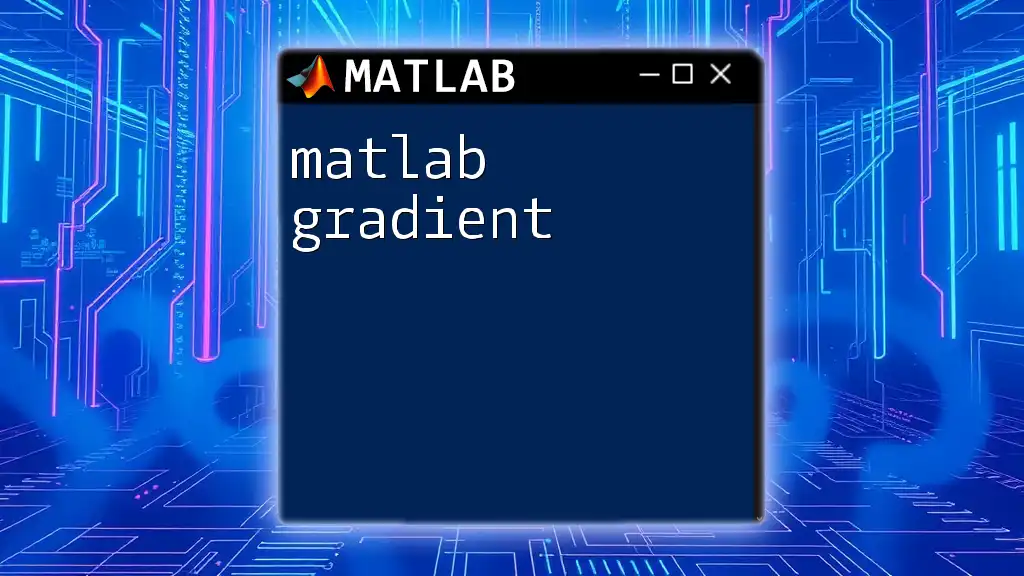
Writing Scripts and Functions
Introduction to Scripts
Scripts in MATLAB are essentially collections of commands executed in sequence. They are useful for automating tasks and can be easily created using the Editor.
To create and run a simple script, consider the following example:
% MyFirstScript.m
disp('Hello, MATLAB!'); % Displays text in Command Window
When this script is run, it will print “Hello, MATLAB!” in the Command Window, demonstrating how scripts can output results to users.
Creating Functions
Unlike scripts, functions allow you to encapsulate logic that can be reused multiple times. The syntax for defining a function is straightforward. Here’s an example of a simple function:
function output = squareNumber(x)
output = x^2; % Returns the square of x
end
This function named `squareNumber` takes an input `x` and returns its square. Functions can take multiple inputs and outputs, enhancing versatility in your code.
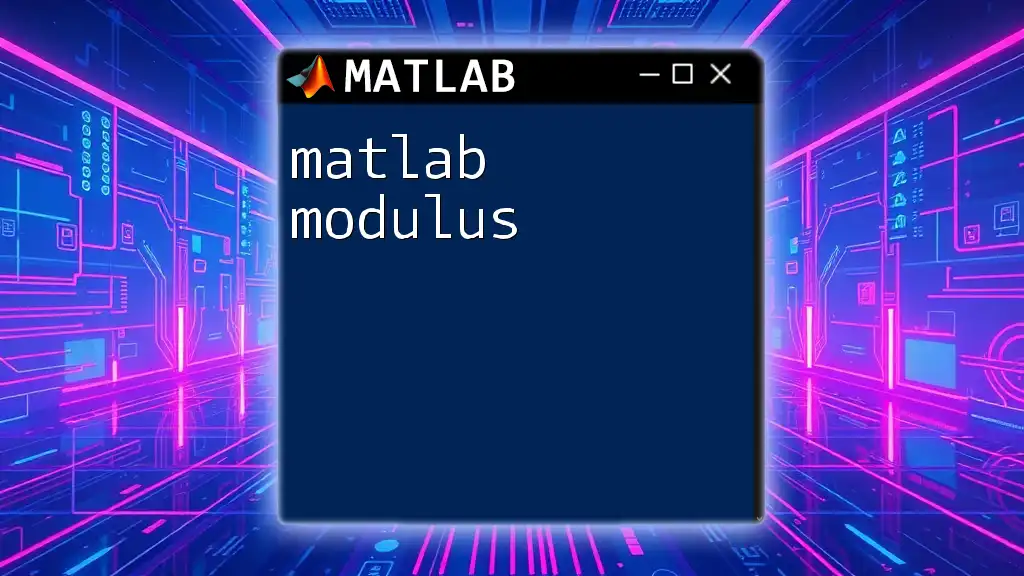
Data Visualization
Plotting Basics
Visual representation of data plays a crucial role in analysis. MATLAB provides a variety of plotting functions to visualize data effectively.
For a basic 2D plot, consider the following example:
x = 0:0.1:10; % Generate a range of values
y = sin(x); % Calculate the sine of each value
plot(x, y); % Basic 2D line plot
Executing this code generates a sine wave, illustrating how MATLAB can be utilized to visualize mathematical functions.
Advanced Plotting Techniques
As you become more comfortable with basic plotting, you can explore advanced techniques such as using `subplot`, `scatter`, and `bar` plots for better data representation.
For example, to create a bar chart:
categories = {'A', 'B', 'C'};
values = [4, 3, 5];
bar(values);
set(gca, 'xticklabel', categories); % Setting custom category labels
This snippet generates a vertical bar chart where each bar corresponds to a category.
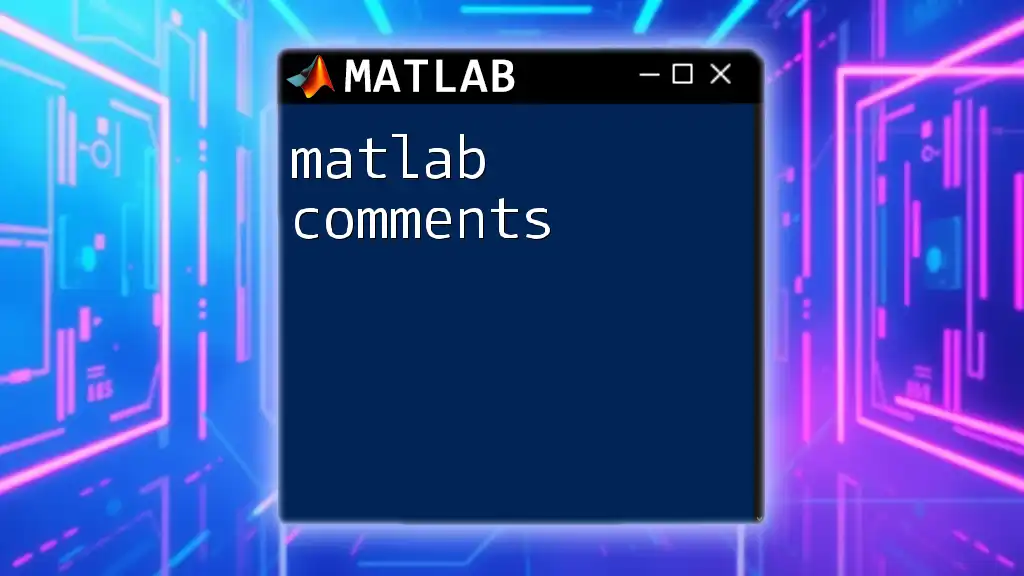
Importing and Exporting Data
Reading from Files
One of MATLAB’s strong suits is its ability to import and manage data from external files. For instance, to read data from a CSV file, you can use:
data = readtable('data.csv'); % Imports data from a CSV file
This command not only reads the data but also formats it into a table, making it easy to manipulate and analyze.
Writing Data to Files
Equally important is exporting data after analysis. You can save your results back to a file using straightforward commands, such as:
writetable(data, 'output.csv'); % Exports data to a CSV file
This saves your data in a format that can be easily shared or used in other applications.
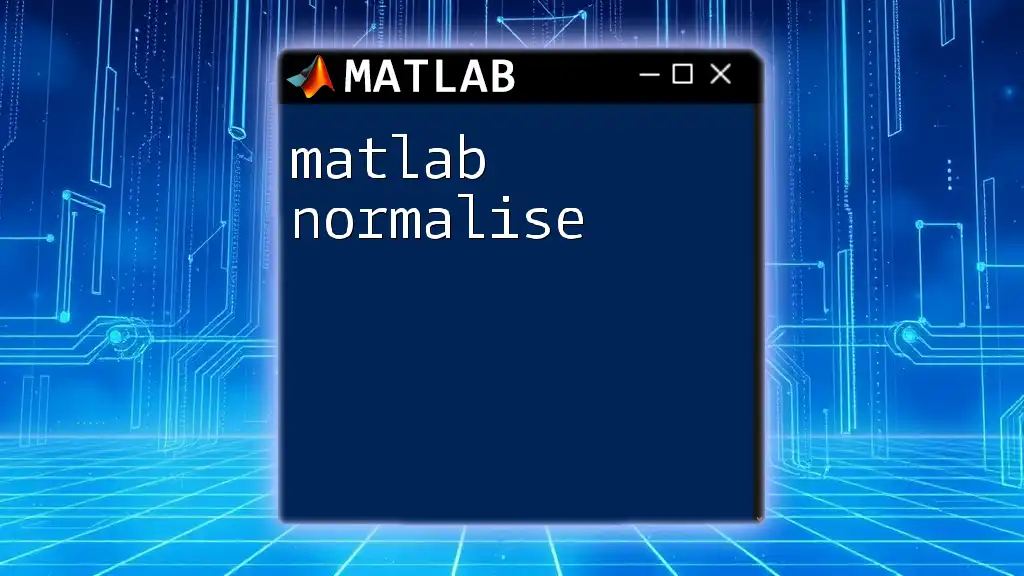
Debugging and Error Handling
Common Errors and Troubleshooting
Every programmer encounters errors, so understanding them is crucial. Syntax errors occur when the code does not conform to MATLAB’s grammar, while runtime errors happen when executing invalid operations (like division by zero).
MATLAB provides useful error messages that can guide you towards fixing issues, which can significantly help in your learning process.
Using the Debugger
MATLAB comes equipped with a built-in debugger to assist in troubleshooting your code. You can set breakpoints to pause execution, allowing you to inspect variable values in the workspace.
This step-by-step execution can reveal the flow of your program and help identify logical errors or any discrepancies in variable values.
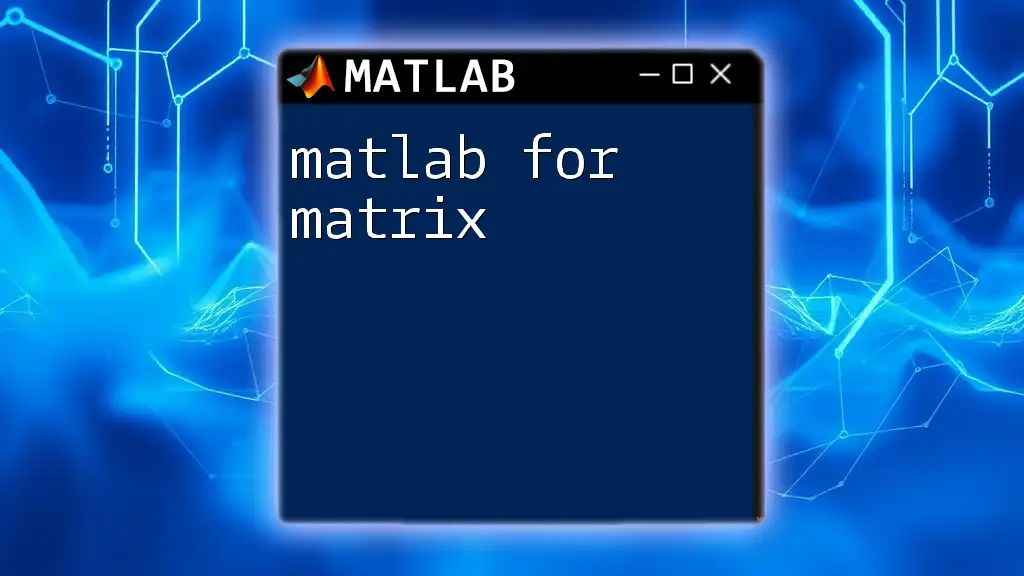
Conclusion
Congratulations! You now have a foundational understanding of MATLAB that will enable you to handle basic operations, visualize data, and even debug your code. As you continue your learning journey, don’t hesitate to explore more advanced features of MATLAB, such as toolboxes for specific applications in fields like signal processing, image processing, and machine learning.
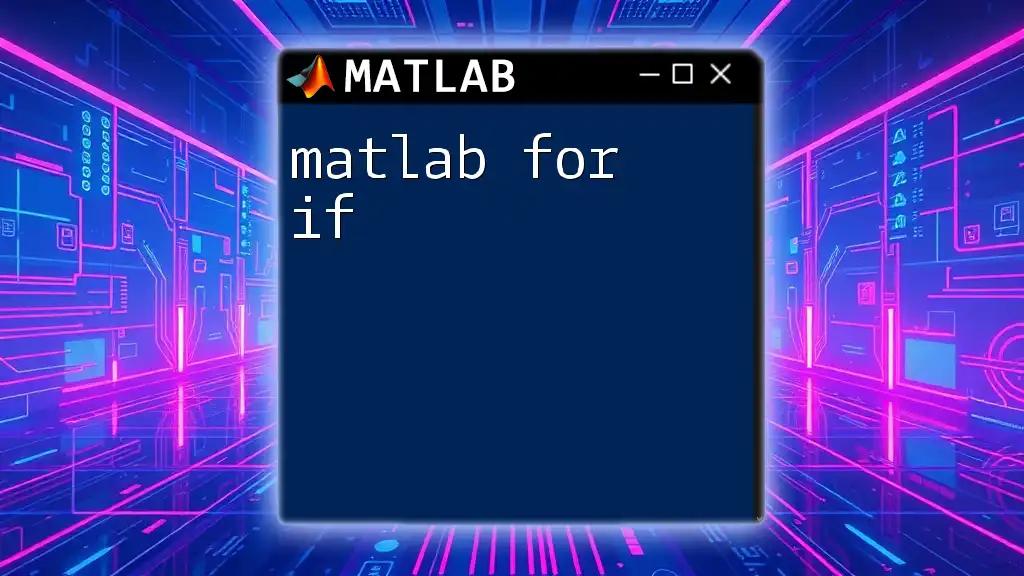
Call to Action
If you're eager to deepen your MATLAB skills, join our educational program where we provide hands-on training and resources tailored for beginners. Subscribe to our updates for more tips and tutorials to make your MATLAB learning experience both effective and enjoyable!