A while loop in MATLAB repeatedly executes a block of code as long as a specified condition is true, enabling iterative processing of tasks until the condition changes.
% Example of a while loop in MATLAB
i = 1;
while i <= 5
disp(['Iteration: ', num2str(i)]);
i = i + 1;
end
What is a While Loop?
In programming, a while loop is a control flow statement used for repeated execution of a block of code as long as a specified condition remains true. It is particularly useful for scenarios where the number of iterations is not known in advance. Instead, the loop continues until a specified condition is no longer met.
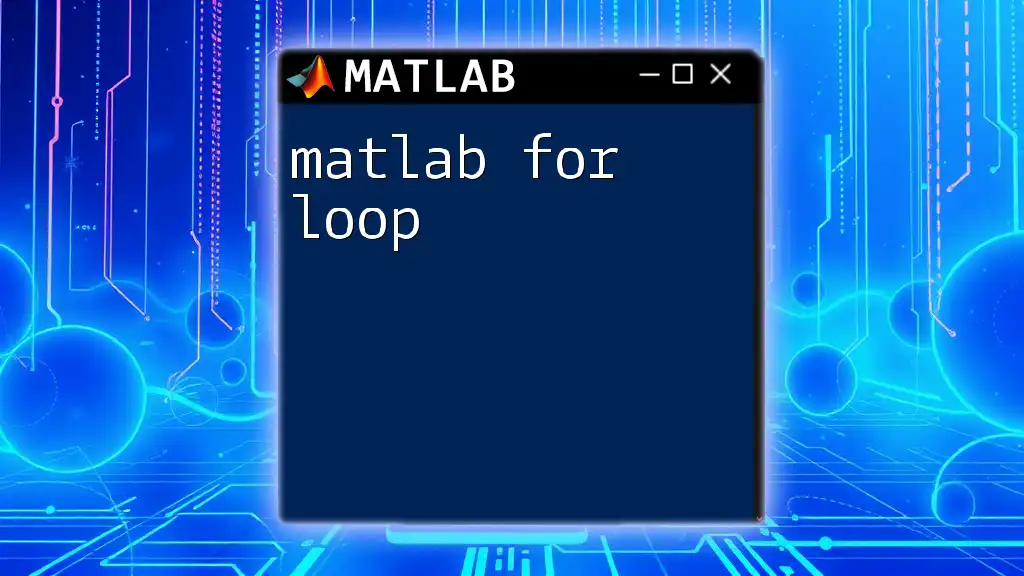
Why Learn While Loops in MATLAB?
Learning how to effectively use while loops in MATLAB is vital for programmers and engineers alike. While loops allow for flexible iterations which can adapt to varying conditions, making them invaluable in tasks such as data analysis, simulations, and automating processes. Understanding while loops will enhance your coding abilities and allow you to tackle real-world problems.
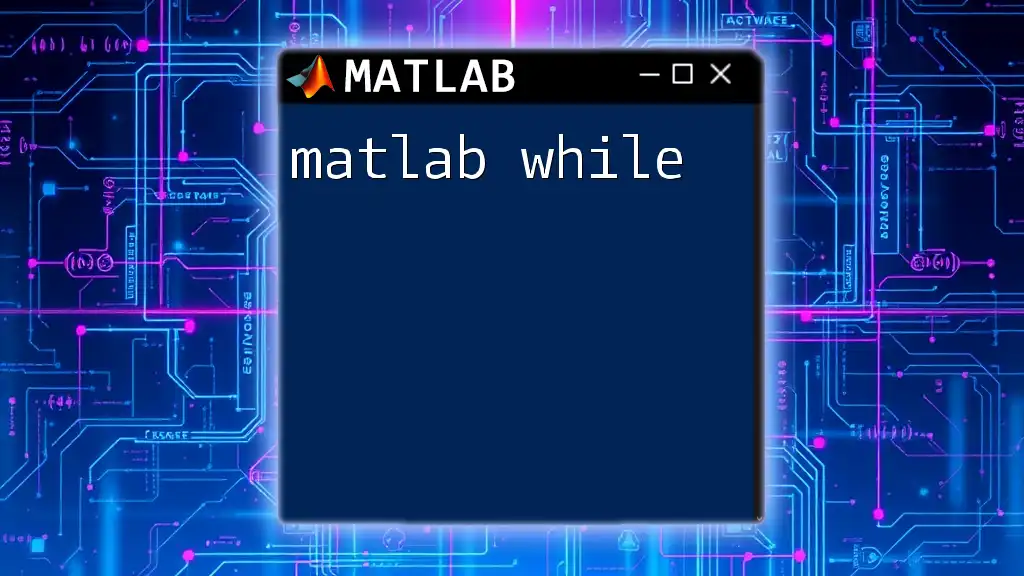
Basic Syntax of While Loops
The basic syntax for a while loop in MATLAB is straightforward. The general structure can be expressed as follows:
while condition
% code to execute
end
Within this syntax:
- `condition` determines whether the loop executes.
- The loop body comprises the code that runs while the condition evaluates to true.
- The `end` statement marks the conclusion of the loop.
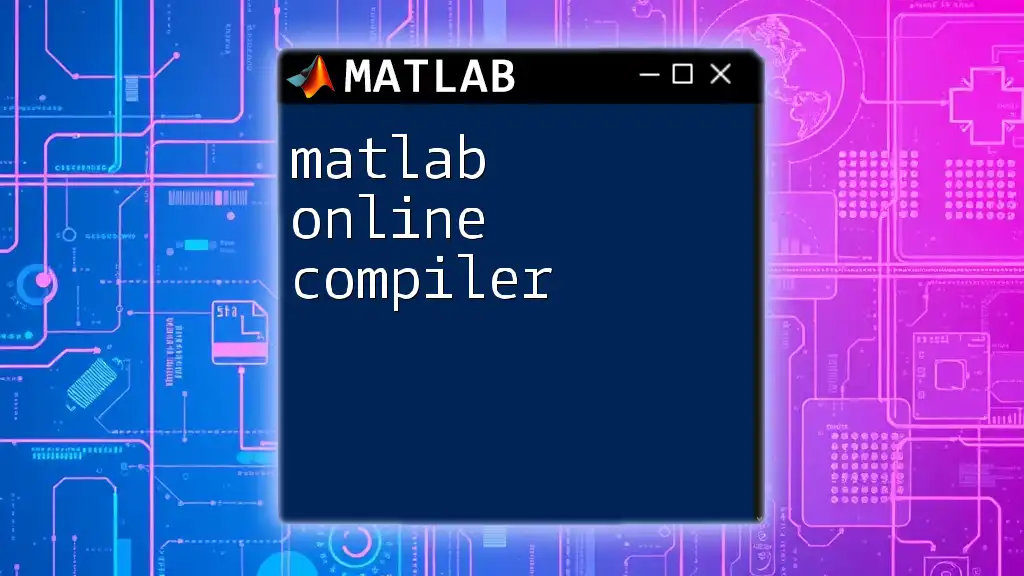
Setting Up a Simple While Loop
Let’s explore how to set up a simple while loop by considering a scenario where we want to count from 1 to 5.
count = 1;
while count <= 5
disp(count);
count = count + 1;
end
In this example:
- We initialize a variable `count` to 1.
- The while loop checks if `count` is less than or equal to 5.
- Inside the loop, `disp(count)` will print the current value.
- Finally, we increment `count` by 1. The loop will iterate until `count` exceeds 5.
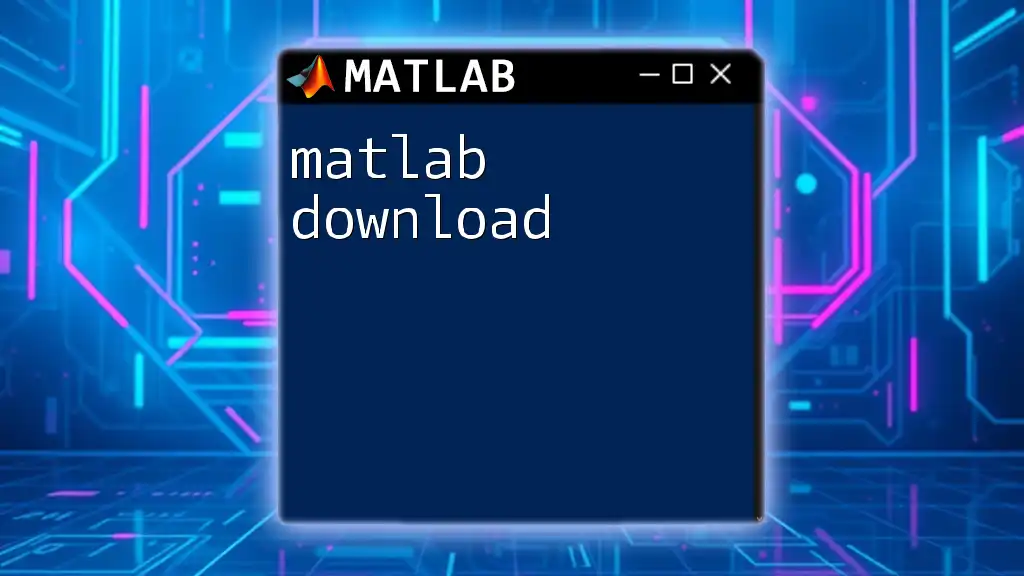
Nested While Loops
While loops can be nested within one another. This is especially helpful for complex tasks. For instance, let’s create a multiplication table using nested while loops:
outer = 1;
while outer <= 5
inner = 1;
while inner <= 5
fprintf('%d * %d = %d\n', outer, inner, outer * inner);
inner = inner + 1;
end
outer = outer + 1;
end
In this example:
- The outer loop iterates through each number from 1 to 5.
- The inner loop, for each iteration of the outer loop, goes through numbers 1 to 5 multiplying and displaying the result.
- This allows us to produce a complete multiplication table for numbers 1 through 5.
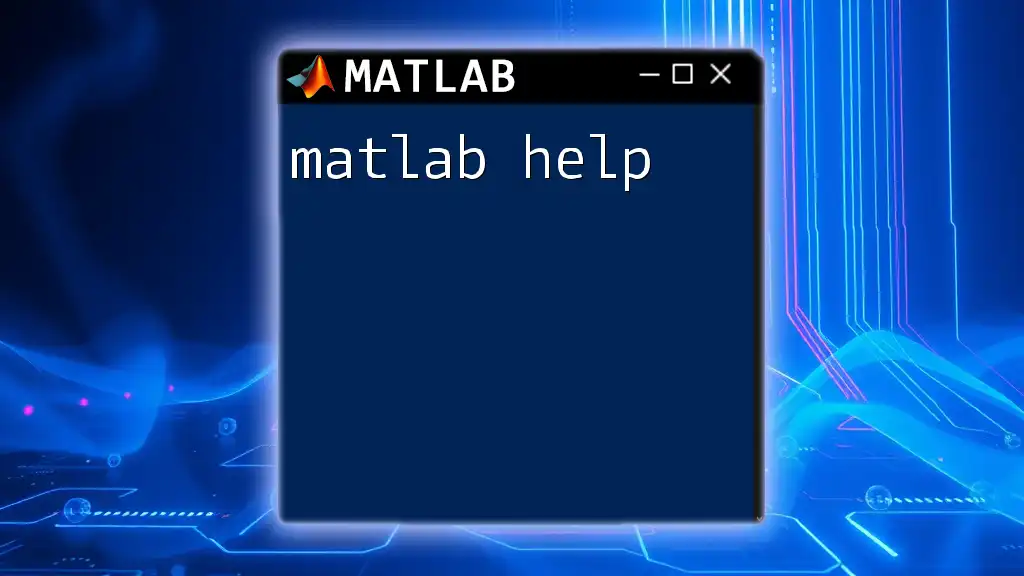
Best Practices When Using While Loops
Avoiding Infinite Loops
One of the most common issues with while loops is the risk of creating an infinite loop, where the condition never becomes false, causing the loop to execute indefinitely. To prevent this, it’s critical to ensure that the loop control variable is updated appropriately within the loop body.
Using Break Statements
In some situations, you may want to exit a while loop prematurely. This can be accomplished using the `break` statement. Here’s how it works:
count = 1;
while count <= 10
if count == 6
break; % Exit the loop when count reaches 6
end
disp(count);
count = count + 1;
end
In this example, when `count` reaches 6, the loop is terminated early, demonstrating how `break` can be used to control loop execution.
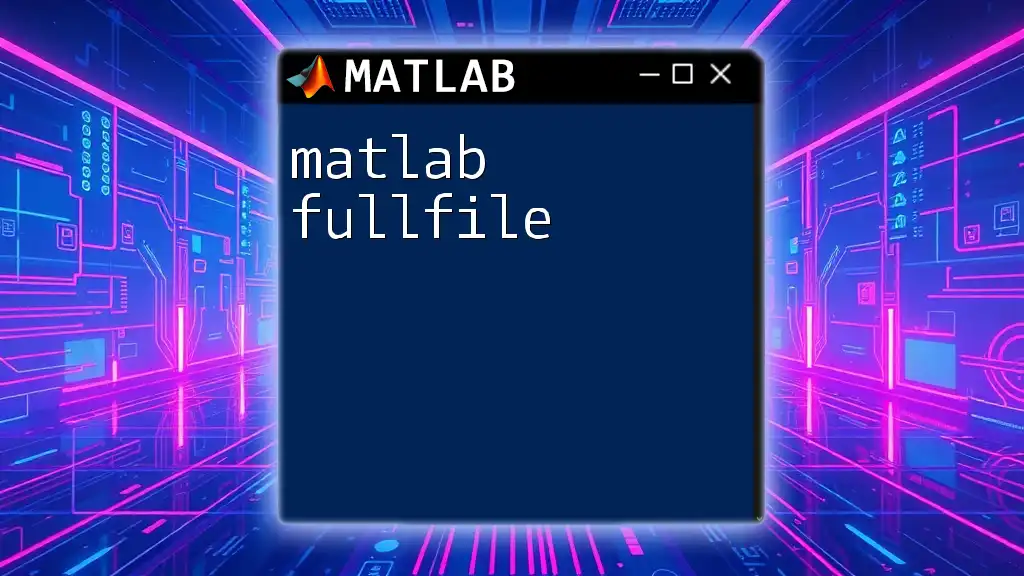
Common Mistakes with While Loops
Incorrect Conditions
A common mistake is setting an incorrect condition for the while loop, which may lead to unexpected results or even infinite loops. Always ensure the condition logically reflects when you want the loop to stop.
Failure to Update Loop Variables
Another frequent issue occurs when you forget to update the loop variable inside the loop. This critical oversight can create an infinite loop since the condition will never change.
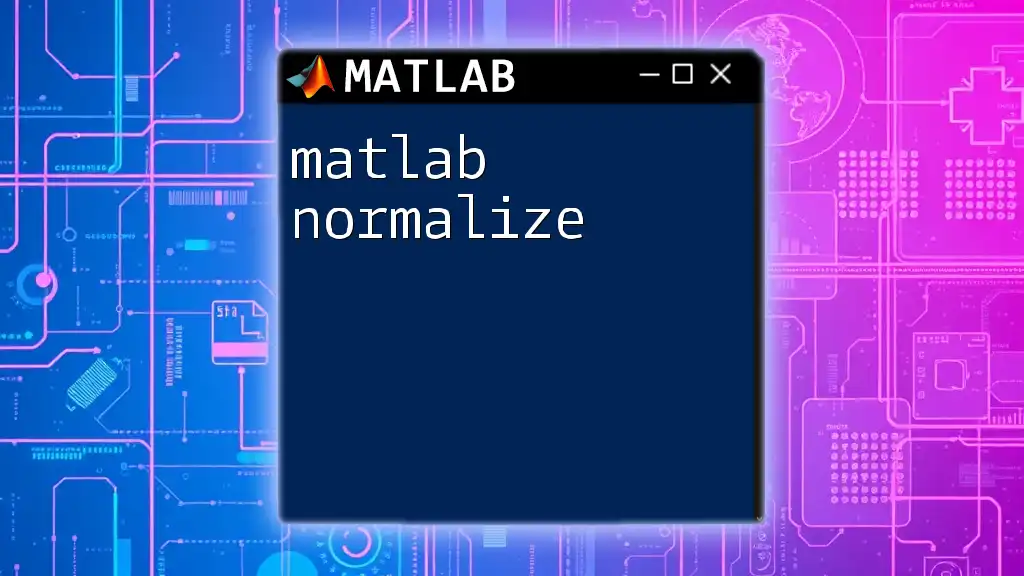
Advanced Topics in While Loops
Creating Efficient While Loops
Efficiency is crucial when running while loops, especially in data-heavy applications. To create efficient loops, consider minimizing the number of operations within the loop and utilizing vectorized operations whenever possible. While loops should generally be a last resort when tasks cannot be accomplished through vectorization.
Combining With Other Control Structures
While loops can be effectively combined with other control structures like if-else statements or even nested for-loops. Such combinations can offer more control and flexibility in handling complex programming tasks.
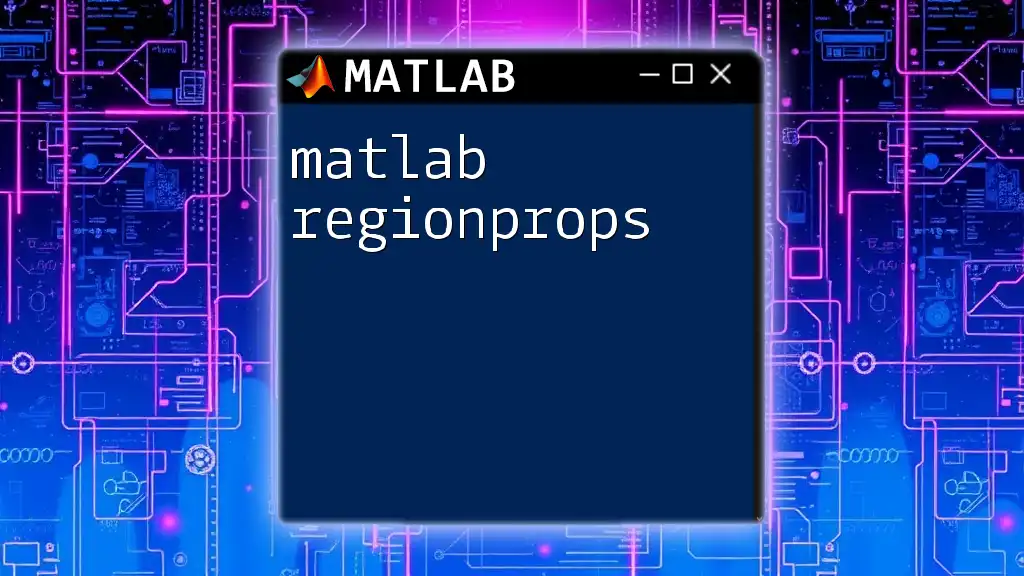
Practical Applications of While Loops
Data Processing Tasks
While loops are particularly beneficial for data processing tasks. For example, reading through a file until EOF (End of File) can be efficiently handled using a while loop. This usage illustrates the adaptability and power of while loops in handling real-world data.
Simulations and Modelling
In simulations where the outcome depends on dynamically changing conditions, while loops can provide the necessary iteration until specific parameters are met. This is critical in fields such as engineering and physics where models may require iterative computations to converge on a solution.
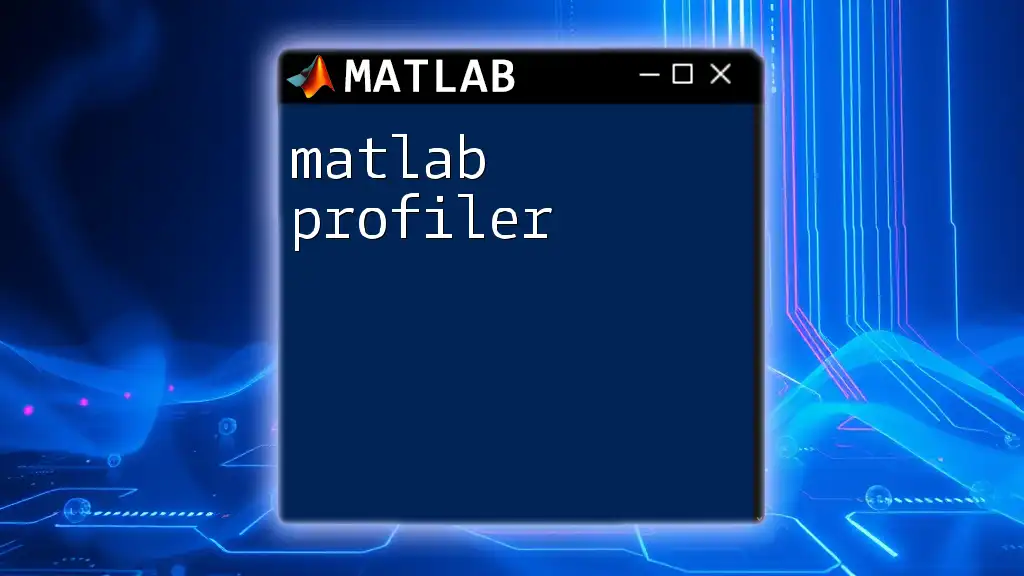
Conclusion
In summary, understanding how to effectively utilize MATLAB for while loops is essential for anyone looking to deepen their programming skills. From basic syntax to advanced techniques, while loops offer a powerful tool for iterative processes. As you practice, take the time to experiment with various scenarios, and don’t hesitate to explore the nuances of this vital control structure. Engage with the community, ask questions, and share your experiences to continue developing your skills!
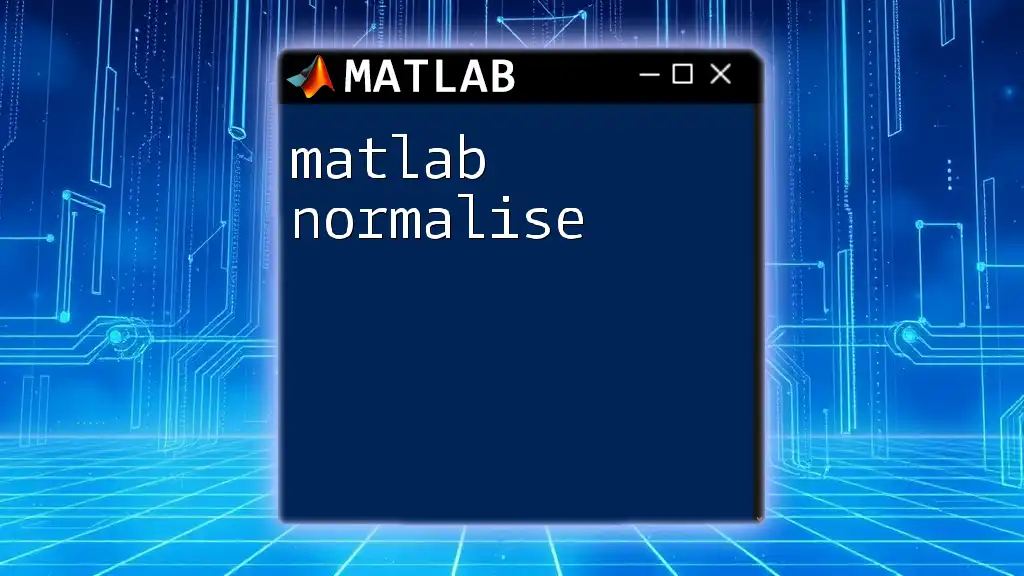
Additional Resources
To further enhance your understanding of while loops, consider diving into MATLAB documentation and the wealth of online resources available. Engaging in practical exercises will also help solidify your knowledge.