In MATLAB, the "greater than or equal to" operator (`>=`) is used to compare two values, returning a logical true (1) if the left operand is greater than or equal to the right operand, and false (0) otherwise.
Here’s a code snippet demonstrating its use:
a = 5;
b = 3;
result = a >= b; % This will return true (1) since 5 is greater than 3
Understanding Relational Operators in MATLAB
What are Relational Operators?
Relational operators are fundamental components of programming languages, including MATLAB. They enable programmers to compare values, which is crucial for decision-making processes within the code. In MATLAB, the common relational operators include:
- `>` (greater than)
- `<` (less than)
- `>=` (greater than or equal to)
- `<=` (less than or equal to)
- `==` (equal to)
- `~=` (not equal to)
The "Greater Than or Equal" Operator
The greater than or equal to operator in MATLAB is denoted as `>=`. This operator is used to compare two values, returning logical `true` (1) if the left operand is greater than or equal to the right operand. Otherwise, it returns `false` (0). The syntax for using this operator is straightforward:
A >= B
where `A` and `B` can both be scalars, arrays, or matrices. Understanding how this operator functions is pivotal for effective data analysis and manipulation in MATLAB.
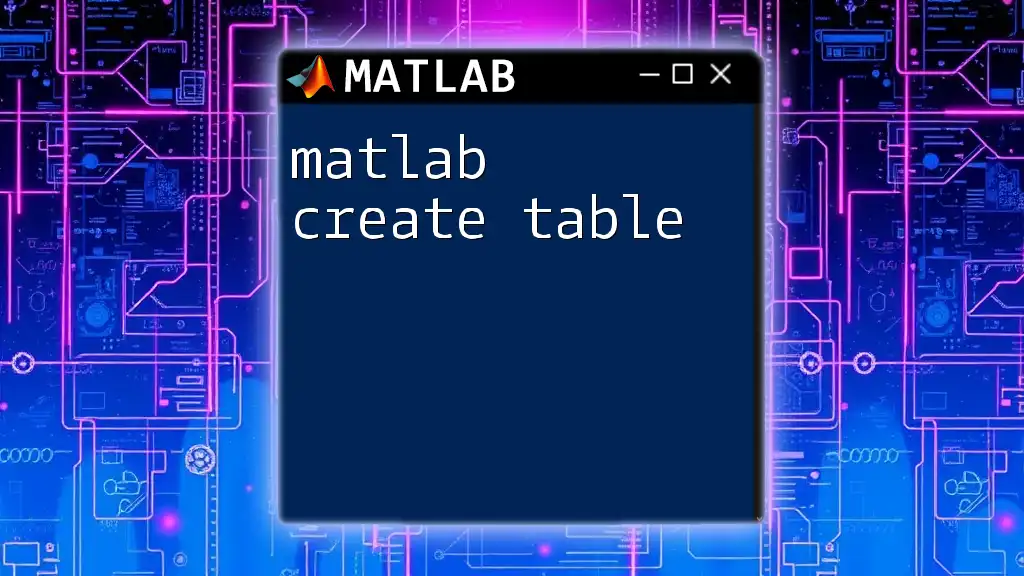
Using the "Greater Than or Equal" Operator in MATLAB
Basic Syntax and Usage
The basic syntax for the greater than or equal operator revolves around two values, as shown below.
To illustrate its use, consider the following example:
A = 5;
B = 3;
result = A >= B; % result will be true (1)
In this case, since `5` is indeed greater than `3`, the result will be `true`.
Comparing Arrays with the Operator
Element-wise Comparison
When working with arrays, the greater than or equal operator performs an element-wise comparison. This means that each element in the first array is compared with the corresponding element in the second array.
Here’s an example demonstrating this:
A = [1, 2, 3, 4];
B = [2, 2, 3, 1];
result = A >= B; % result will be [0 1 1 1]
In this scenario, the output is an array where each position indicates whether the corresponding element of `A` is greater than or equal to `B`.
Logical Array Outputs
The result of the comparison is a logical array where values are either `true` (1) or `false` (0). This output can be particularly useful for logical indexing. For instance, you can use the logical output to filter or select elements from an array.
Consider the following example:
A = [10, 20, 30, 40];
threshold = 25;
result = A >= threshold; % result will be [0 0 1 1]
filtered = A(result); % filtered will be [30 40]
Here, `filtered` contains only the elements of `A` that are greater than or equal to `25`.
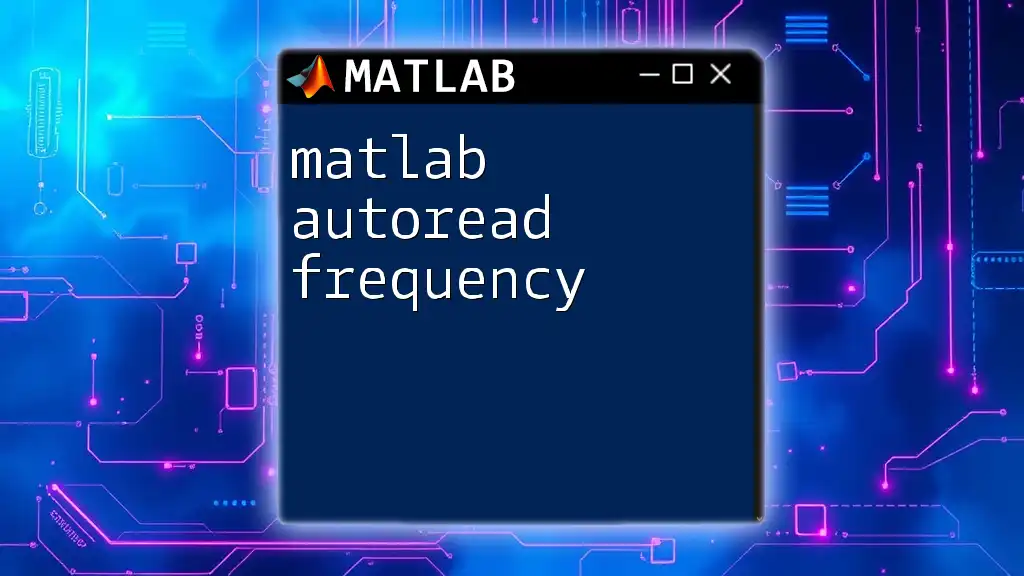
Practical Applications of the Operator
Conditional Statements
The greater than or equal operator is often used within conditional statements. By employing this operator, you can create logical branching in your code based on dynamic conditions.
Here is an example of using the operator in an `if-else` structure:
value = 15;
if value >= 10
disp('Value is greater than or equal to 10');
else
disp('Value is less than 10');
end
In this script, if `value` is `15`, the output will be “Value is greater than or equal to 10.”
Loop Structures
The operator is equally useful within loop structures, enabling iterative checks and statements depending on the condition.
For instance, consider using the greater than or equal operator in a `for` loop:
A = [5, 7, 10, 3, 8];
for i = 1:length(A)
if A(i) >= 7
disp(['Element ', num2str(A(i)), ' is greater than or equal to 7']);
end
end
This code snippet will output elements of the array `A` that are greater than or equal to `7`.
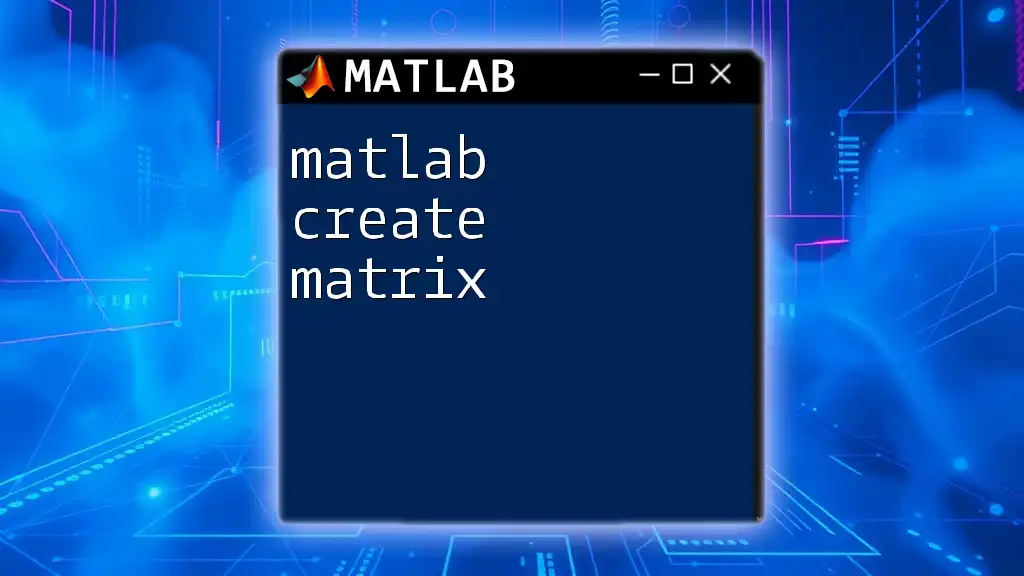
Troubleshooting and Common Errors
Common Mistakes
When utilizing the matlab greater than or equal operator, it’s crucial to ensure the data types are compatible. A frequent mistake is attempting to compare types that do not equate meaningfully, such as numeric arrays with strings or cell arrays. It’s vital to cast data types or convert them appropriately to avoid unexpected results.
Debugging Tips
Debugging issues related to the operator can be straightforward with a few techniques. Using the `class()` function allows you to check the data types of your variables:
disp(class(A)); % Check the type of variable A
Ensuring both operands of the comparison are of compatible types will mitigate many common issues.
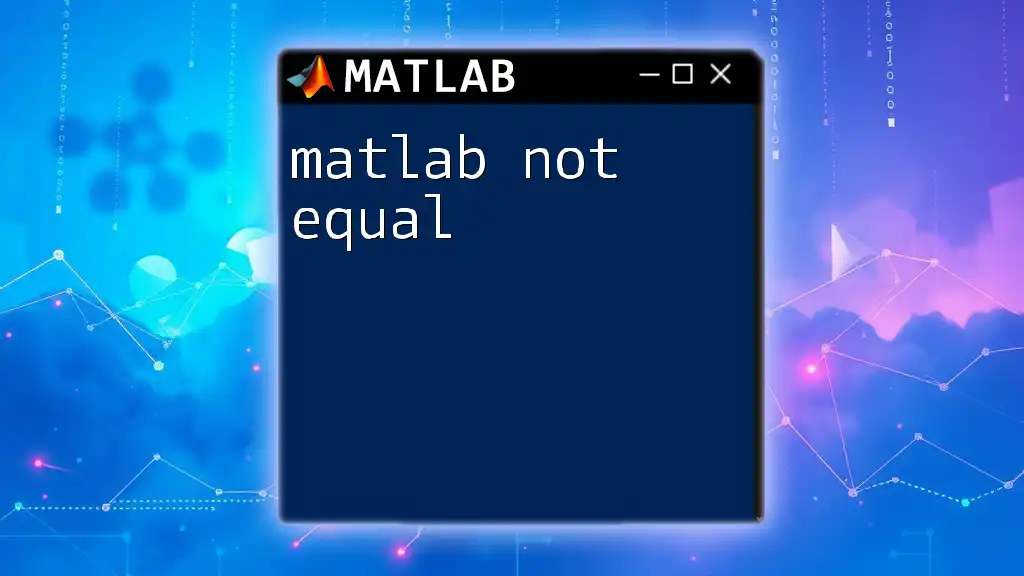
Advanced Topics
Logical Operations and Combining Conditions
The capability of MATLAB to combine multiple logical conditions can enhance the power of decision-making in scripts. You can combine the greater than or equal operator with other logical operators, such as `&` (AND) and `|` (OR).
For example, the following code snippet demonstrates how to check multiple conditions:
A = 15;
B = 10;
C = 5;
if A >= B && A >= C
disp('A is greater than or equal to both B and C');
end
Here, it checks if `A` is greater than or equal to both `B` and `C`.
Vectorized Operations
MATLAB is optimally designed for vectorized operations, allowing for efficient computations. When using the greater than or equal operator across entire matrices, MATLAB performs the operation element-wise, enhancing performance.
For example:
M = [1, 2; 3, 4];
N = [2, 3; 1, 5];
result = M >= N; % Logical matrix output
In this illustration, `result` will contain logical values indicating whether each element of `M` is greater than or equal to the corresponding element of `N`.
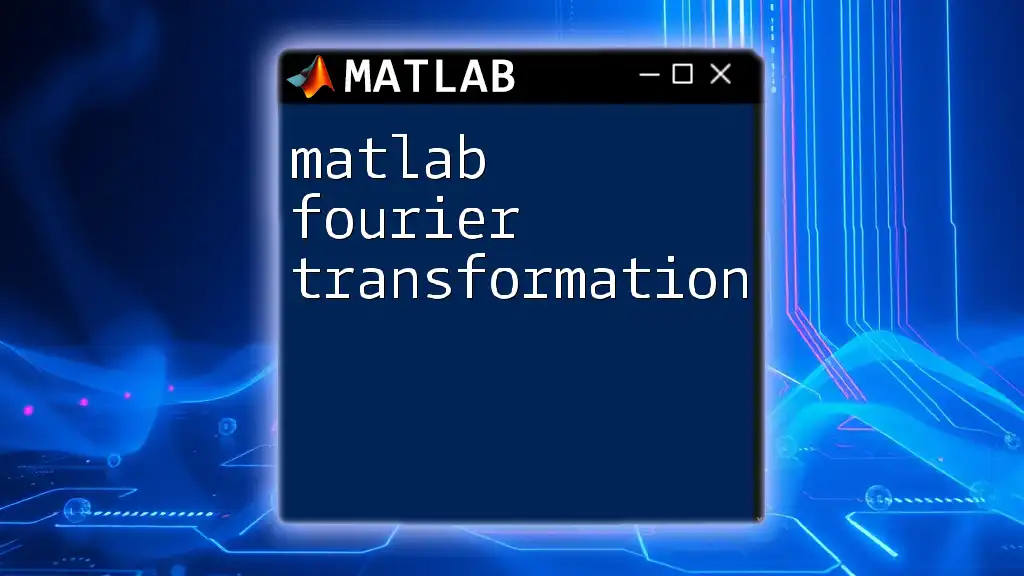
Conclusion
In conclusion, the matlab greater than or equal operator provides fundamental functionality for performing comparisons and conditional logic within MATLAB scripts. By mastering its use, programmers can effectively manipulate and analyze data with precision, leading to more robust MATLAB applications. Practice utilizing this operator across various contexts will further solidify understanding and implementation in your coding practices.
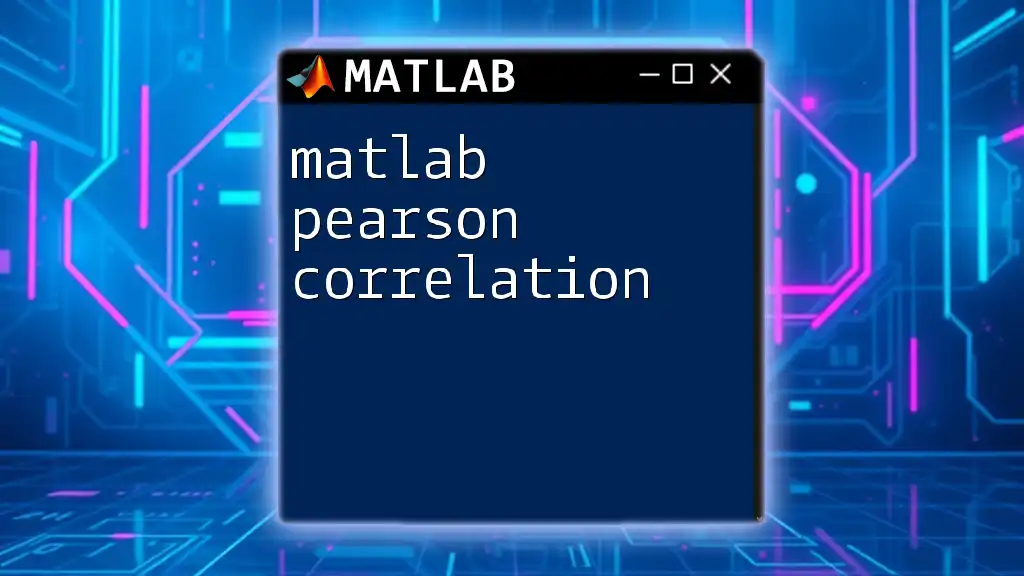
Additional Resources
For further learning, consider exploring the official MATLAB documentation, engaging with online forums, and participating in MATLAB user communities. These resources can provide additional insights and support as you navigate your journey through MATLAB programming.
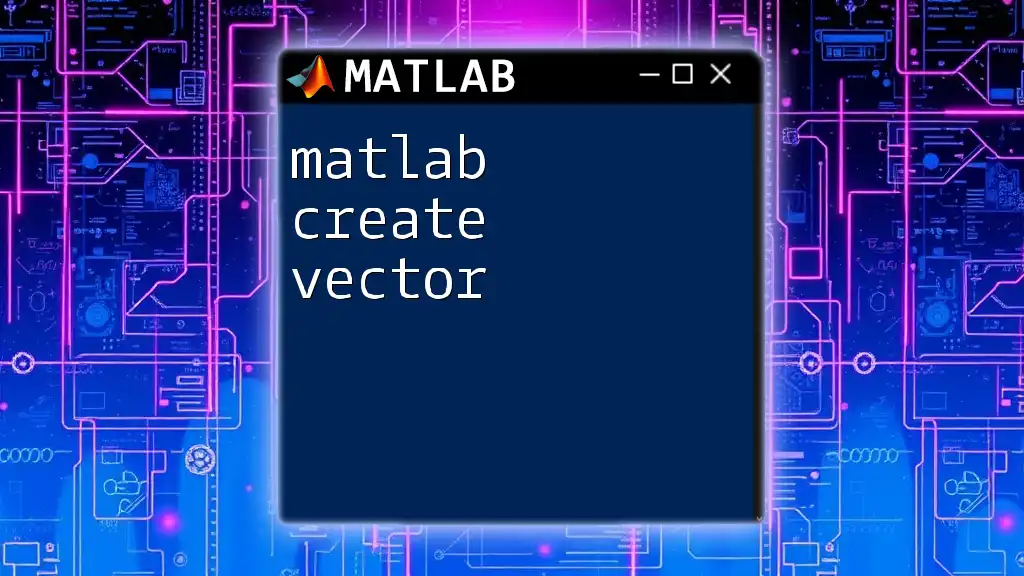
Call to Action
Stay tuned for more articles and tutorials that will enhance your MATLAB skills. Sign up to our newsletter for updates on exciting workshops and classes designed to help you master MATLAB commands efficiently.