In MATLAB, the "not equal" operator is denoted by `~=` and is used to compare two values, returning `true` if they are not equal and `false` otherwise.
Here's a code snippet demonstrating its usage:
a = 5;
b = 3;
result = (a ~= b); % result will be true (1) because 5 is not equal to 3
What is the "Not Equal" Operator in MATLAB?
The not equal operator in MATLAB is represented by `~=`. This operator is fundamental in programming, allowing you to check if two values or expressions are not the same. Understanding how to use this operator is essential for any data manipulation, conditional logic, or matrix operations in MATLAB.
In contrast to the equality operator (`==`), where the result is true if the operands are equal, the not equal operator will yield a true value if the operands differ.
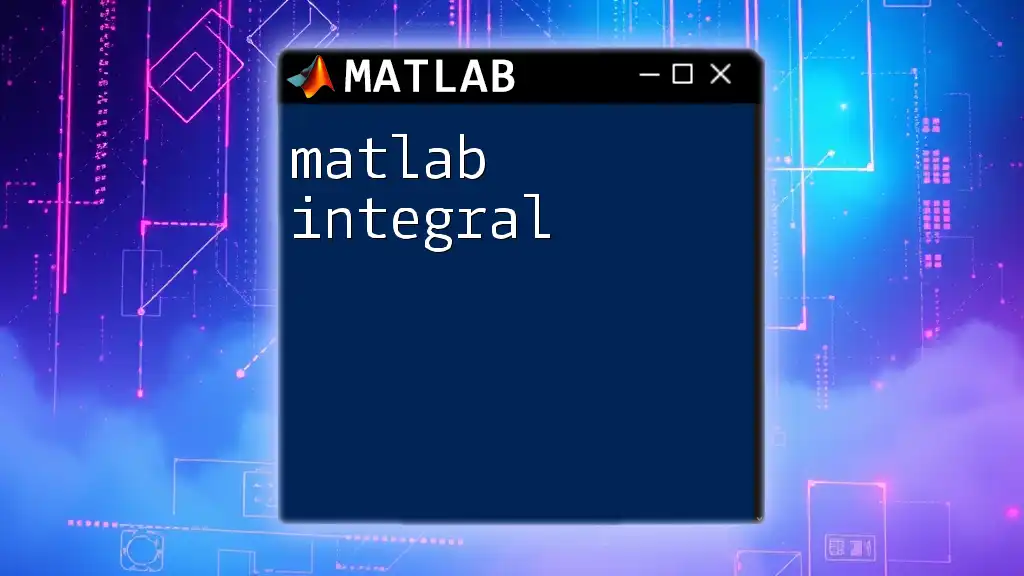
Syntax of the Not Equal Operator
The basic syntax of the not equal operator is straightforward:
result = (a ~= b);
In this example, `result` will be a logical value (`true` or `false`) based on whether `a` and `b` are not equal. It is important to note that the not equal operator can be applied to different data types, including scalars, vectors, matrices, and even strings.
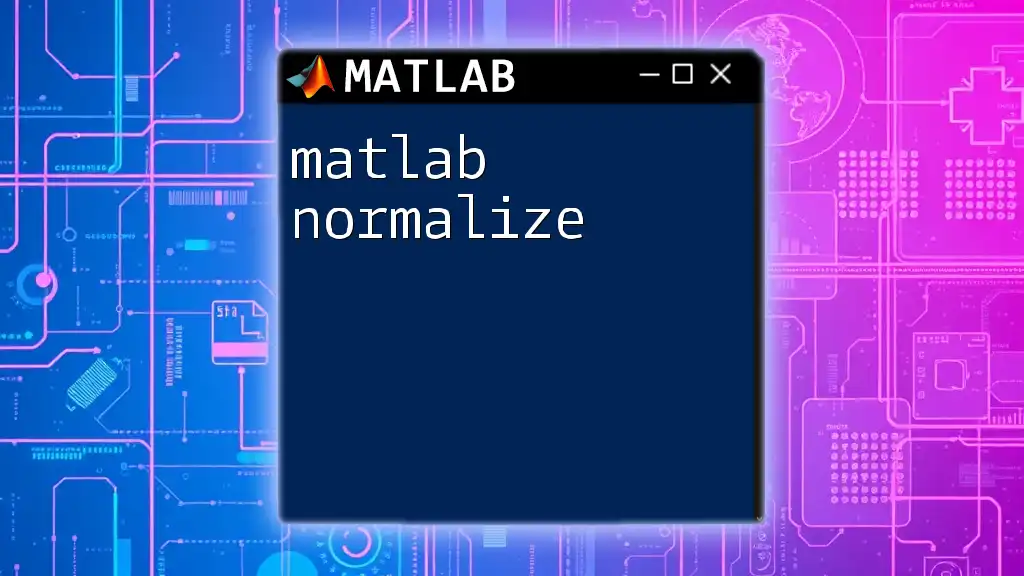
Understanding Logical Arrays
In MATLAB, logical arrays are arrays that contain only `true` (1) or `false` (0) values. When you use the not equal operator on matrices or arrays, MATLAB generates a logical array with the same dimensions, indicating the results of each individual comparison.
Working with Logical Arrays
Let’s consider the following example using arrays:
A = [1, 2, 3; 4, 5, 6];
B = [1, 0, 3; 4, 7, 6];
result = (A ~= B);
In this case, the `result` will produce a logical array:
0 1 0
0 1 0
Explanation: Here, the output indicates where elements in matrices `A` and `B` differ. A `0` represents equality, while a `1` indicates the values are not equal.
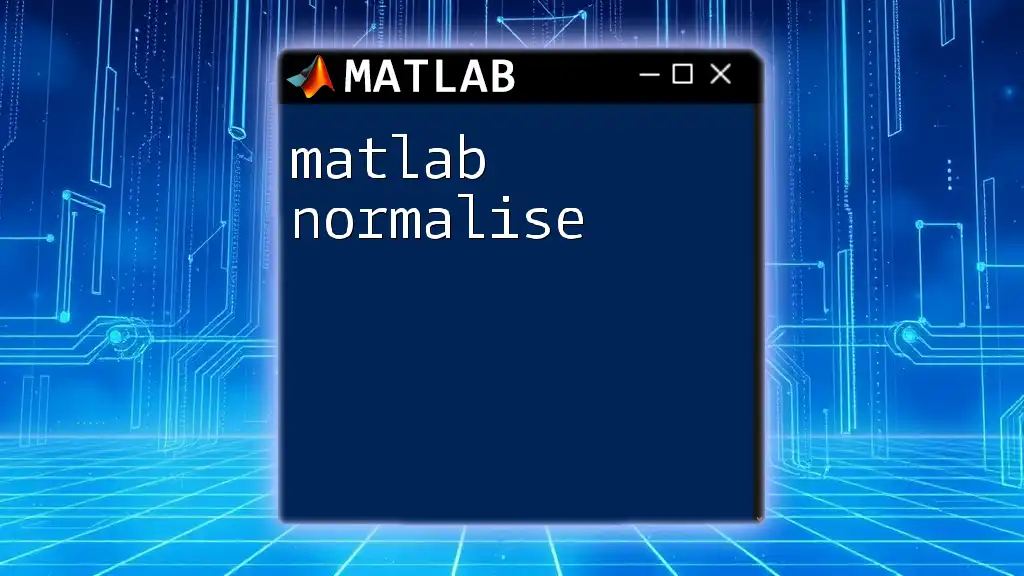
Common Use Cases of the Not Equal Operator
Filtering Data
One of the most practical applications of the not equal operator is in data analysis and filtering datasets. You can easily remove unwanted values from an array using this operator. For example:
data = [1, 2, 3, 4, 5];
filtered_data = data(data ~= 3);
In this scenario, `filtered_data` will output:
1 2 4 5
Explanation: The output successfully filters out the unwanted value (3), demonstrating how the not equal operator can simplify data management.
Conditional Statements
The not equal operator is also frequently used in control flow with conditional statements. Below is an example of how it can be applied:
if a ~= b
disp('a and b are not equal.');
end
Explanation: This simple condition checks if `a` is not equal to `b`. If true, it executes the code inside the `if` block. This logic is crucial for building dynamic applications that respond to varying conditions.
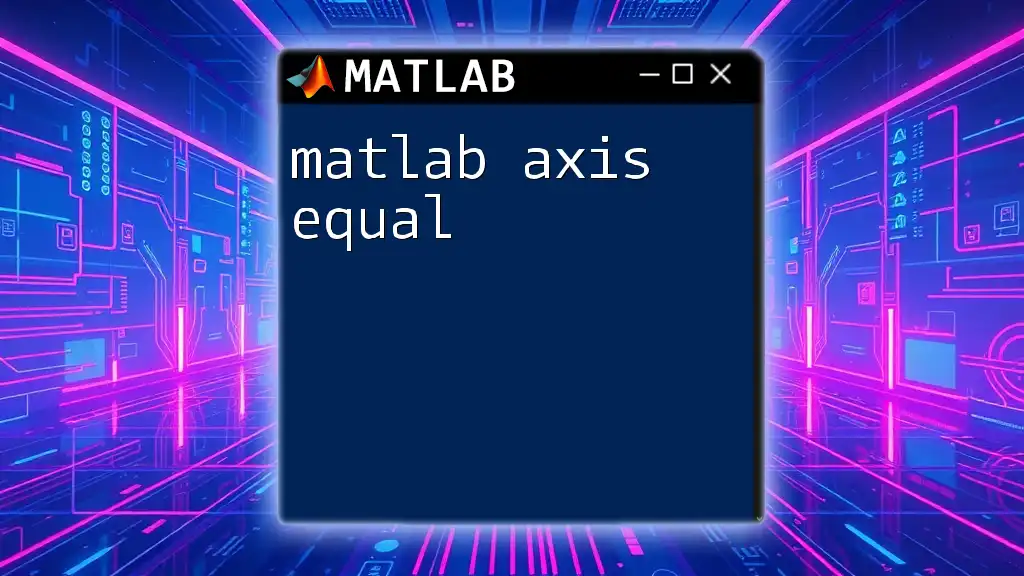
Combining Not Equal with Other Relational Operators
The not equal operator can also be effectively combined with other relational operators to create more complex conditional logic. For instance, consider the following code:
if (x ~= 0) && (y > 5)
disp('Conditions met!');
end
Explanation: In this case, two conditions are checked: whether `x` is not equal to zero and whether `y` is greater than five. Both must be true for the message "Conditions met!" to display. This type of logic is essential in many programming scenarios where you need multiple validations before executing code.
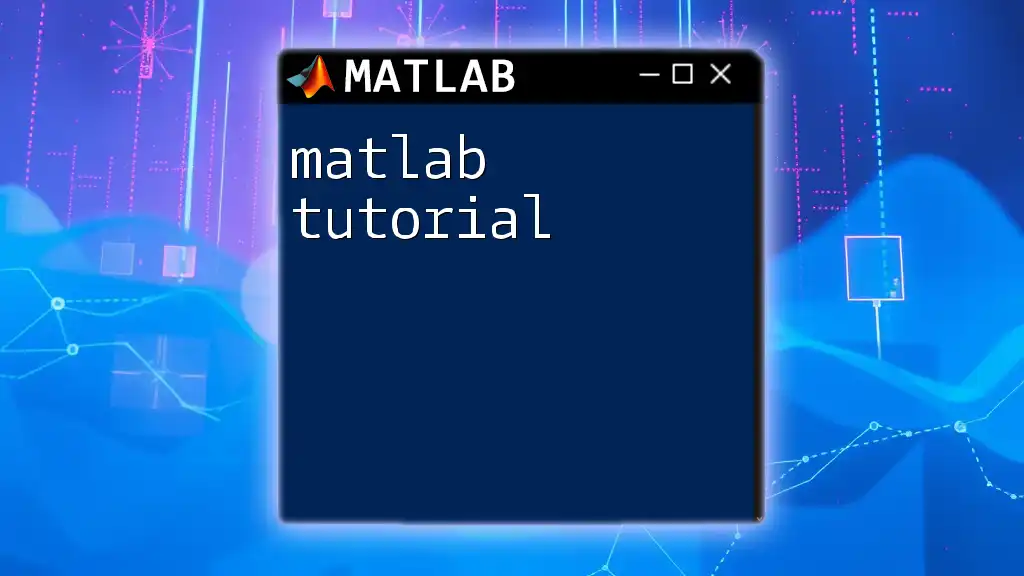
Handling Special Cases
NaN Comparisons
When dealing with numerical computations, you might encounter NaN (Not a Number). This special value can produce unexpected results when using comparison operators. For example:
result = (NaN ~= NaN);
Explanation: Surprisingly, this expression returns `true`. In MATLAB, NaN is not equal to anything, even itself, which is crucial to understand when cleaning datasets or managing computations.
String Comparisons
String comparisons in MATLAB can also utilize the not equal operator. For example, here’s how you can implement it:
str1 = 'hello';
str2 = 'world';
result = ~strcmp(str1, str2);
Explanation: In this example, `strcmp` checks whether the two strings are equal. The tilde (`~`) negates this result, so if the strings are not equal, `result` will be `true`. This allows for a variety of logical checks and filters when working with text data.
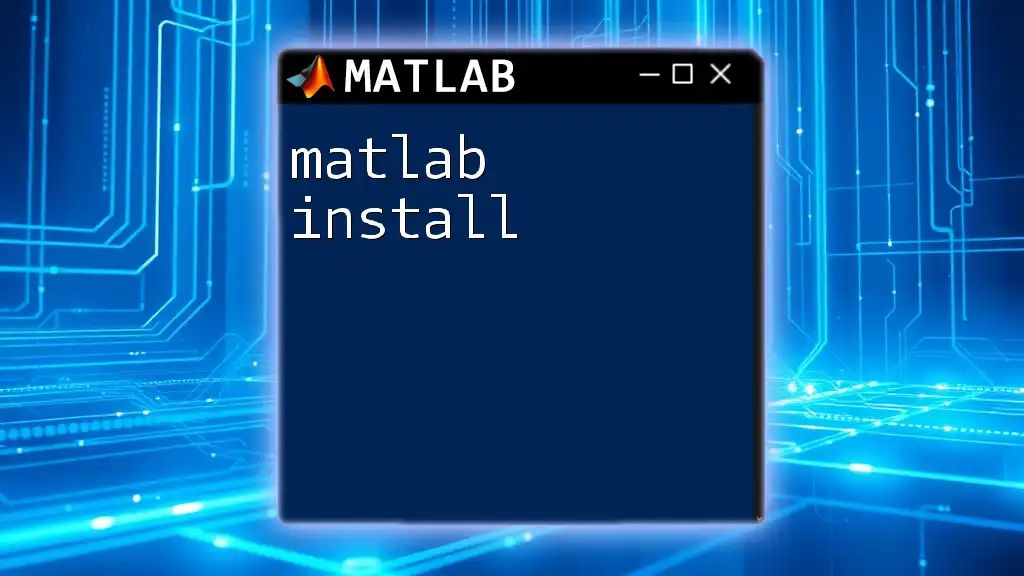
Best Practices
When using the MATLAB not equal operator, consider the following best practices:
- Understand Data Types: Ensure you know how different data types behave when compared using `~=`. For example, comparing cell arrays with numeric arrays produces errors.
- Avoid Common Pitfalls: Always use `~=` for not equal comparisons to prevent bugs. Using `==` mistakenly can lead to logical errors in your programs.
- Test Your Logic: Small test cases can help verify that your comparisons and filtering operations behave as expected.
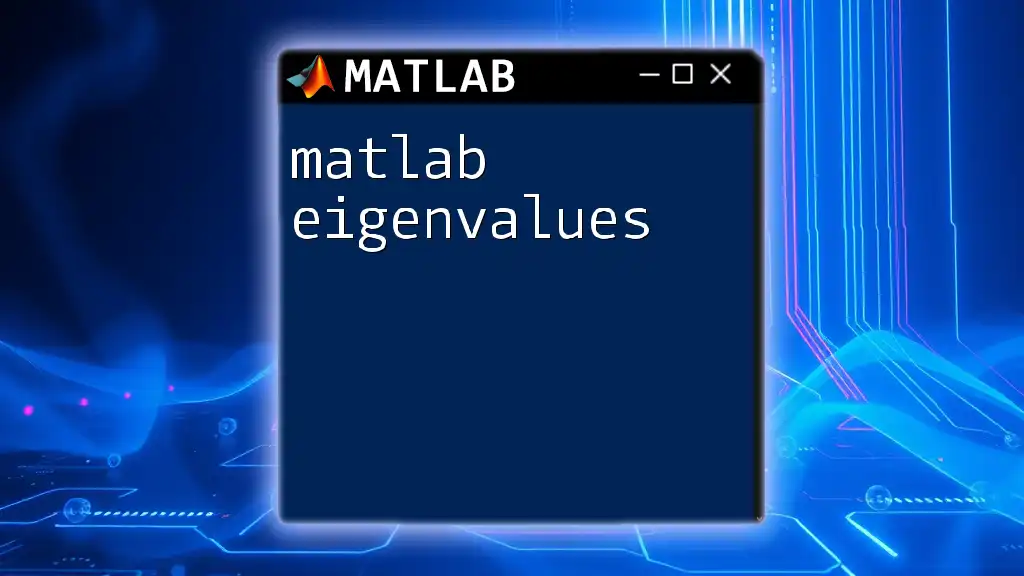
Conclusion
Understanding how the MATLAB not equal operator works is essential for effective programming in MATLAB. Whether you are filtering arrays, making conditional decisions, or dealing with special cases like NaN or strings, mastering the not equal operator will enhance your coding skills and improve your data analysis capabilities.
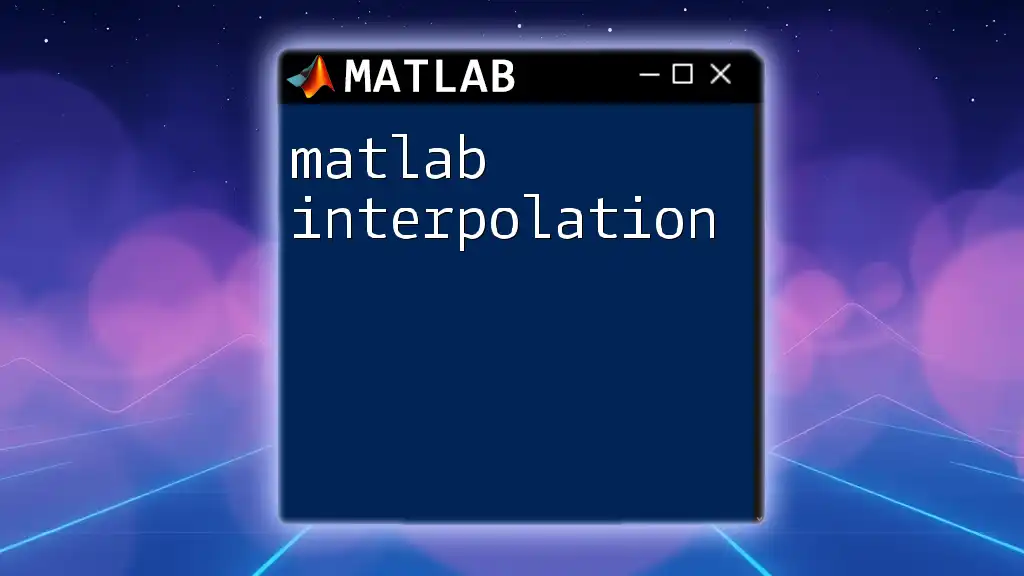
Additional Resources
For further learning, consider exploring the MATLAB documentation, which offers a trove of references and guides on relational operators. Additionally, online courses and tutorials can provide practical hands-on experience with MATLAB programming.
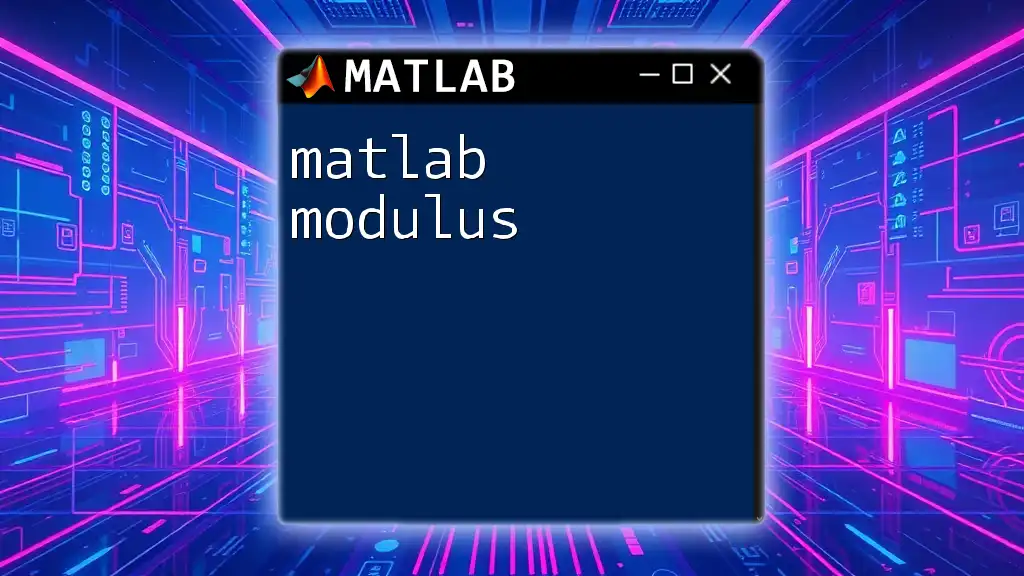
Call to Action
Put your knowledge into practice by using the not equal operator in different scenarios! Experiment with comparisons, conditional logic, and data filtering. If you’re eager to learn more about MATLAB, reach out to us for specialized teaching opportunities that can elevate your skills.