MATLAB Object-Oriented Programming (OOP) allows you to define custom classes that encapsulate data and methods, enabling more structured and reusable code.
Here’s a simple example of defining a class in MATLAB:
classdef Dog
properties
Name
Age
end
methods
function obj = Dog(name, age)
obj.Name = name;
obj.Age = age;
end
function bark(obj)
fprintf('%s says: Woof!\n', obj.Name);
end
end
end
Introduction to Object-Oriented Programming in MATLAB
Object-Oriented Programming (OOP) is a programming paradigm that utilizes "objects" to design software programs. In MATLAB, OOP enables developers to create reusable, modular, and organized code structures, making it easier to manage and extend applications.
What is Object-Oriented Programming (OOP)?
OOP is fundamentally a way to approach programming where functionalities are encapsulated within specific data structures, known as objects. Each object is an instance of a class, which can have properties (characteristics) and methods (behaviors). OOP helps streamline complex code by promoting a design that mirrors real-world entities, making it intuitive to understand and maintain.
Key Advantages of OOP in MATLAB:
- Code Reusability: Classes can be reused across different projects.
- Modularity: Code can be broken down into discrete components.
- Encapsulation: Data and methods are kept private, reducing complexity.
- Ease of Maintenance: Changes can be made to classes independently, minimizing the risk of breaking other parts of the code.
Overview of OOP Concepts
Understanding OOP hinges on several core concepts:
- Classes: Blueprints for creating objects.
- Objects: Instances of classes with specific states.
- Inheritance: Ability to create new classes based on existing ones.
- Polymorphism: Ability to redefine methods in derived classes.
- Encapsulation: Restricting access to certain components of a class.

Understanding Classes and Objects in MATLAB
Defining a Class
In MATLAB, a class is defined using the `classdef` keyword, marking the beginning of a class definition. The properties and methods associated with a class follow the class definition.
Here’s an example of creating a simple class called `Dog`:
classdef Dog
properties
Name
Age
end
methods
function obj = Dog(name, age)
obj.Name = name;
obj.Age = age;
end
end
end
In this code snippet, the `Dog` class has two properties: `Name` and `Age`. The constructor method initializes these attributes when a new `Dog` object is created. Understanding the structure and purpose of each component is crucial for effective OOP in MATLAB.
Creating Objects from Classes
After defining a class, creating an instance (or object) is straightforward. For instance, you can instantiate the `Dog` class as follows:
myDog = Dog('Buddy', 3);
This line creates an object named `myDog` with the name Buddy and the age 3. With OOP, this object now holds specific data, making it easier to manage and manipulate.

Properties and Methods in Classes
Understanding Properties
Properties are variables that belong to a class, representing the state of an object. They can be accessed or modified directly:
myDog.Name % Returns 'Buddy'
myDog.Name = 'Max'; % Changes the dog's name to 'Max'
This flexibility allows you to work with the object’s data easily and can be essential when building interactive applications.
Defining Methods
Methods are functions that define the behaviors of a class and can manipulate object data. Here’s an example of a method that returns the dog's information using the previously defined properties:
methods
function info = getDogInfo(obj)
info = sprintf('Name: %s, Age: %d', obj.Name, obj.Age);
end
end
To use this method, you simply call it on the object:
info = myDog.getDogInfo();
This returns a string with the dog’s information, demonstrating how encapsulated logic can be accessed through refined interfaces.
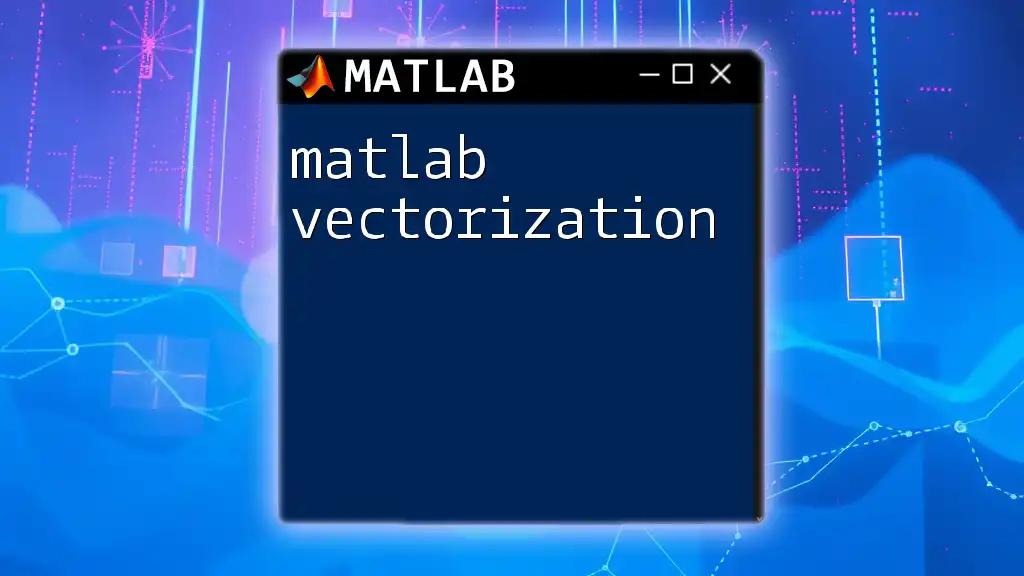
Inheritance in MATLAB Classes
What is Inheritance?
Inheritance allows you to create new classes based on existing ones, inheriting properties and methods, which facilitates code reuse. The class being inherited from is called the superclass, and the newly defined class is called the subclass.
Creating Subclasses in MATLAB
To create a subclass in MATLAB, use the `<` operator to indicate inheritance. For example, let’s create a subclass `ServiceDog` that extends the `Dog` class:
classdef ServiceDog < Dog
properties
ServiceType
end
methods
function obj = ServiceDog(name, age, serviceType)
obj@Dog(name, age); % Call the superclass constructor
obj.ServiceType = serviceType;
end
function info = getServiceDogInfo(obj)
info = sprintf('%s, Service Type: %s', getDogInfo(obj), obj.ServiceType);
end
end
end
Here, the `ServiceDog` class inherits the properties and methods of the `Dog` class while also introducing a new property `ServiceType` and a method `getServiceDogInfo`.
Overriding Methods
You can also override a method from the superclass in the subclass. For instance, if you want `ServiceDog` to provide additional information when calling `getDogInfo`, you can redefine it in `ServiceDog`.
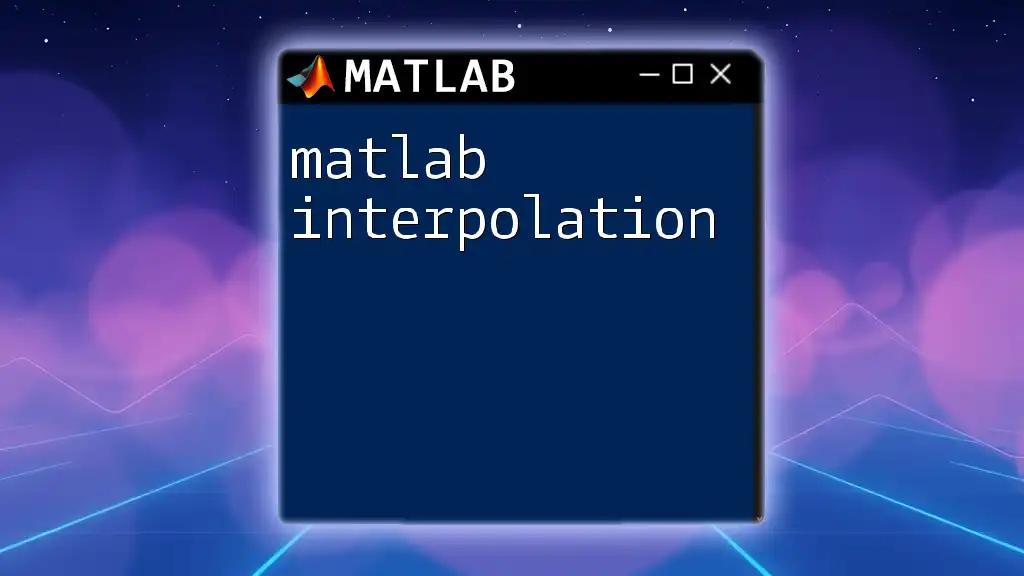
Polymorphism in MATLAB
Understanding Polymorphism
Polymorphism allows different classes to be treated as instances of the same class through a common interface. This means a single method name can behave differently based on the object that calls it.
Using Polymorphism with Method Overloading
Method overloading is an aspect of polymorphism that allows you to create multiple methods with the same name but different signatures. For example, you can have a method that calculates areas for different shapes (a rectangle or a circle) under the same name, adapting to the parameters received.

Encapsulation in MATLAB OOP
What is Encapsulation?
Encapsulation restricts direct access to some of an object's components. This is a fundamental principle that protects the integrity of the object. Accessing properties directly can lead to unforeseen issues if not managed properly.
Access Control: Public, Private, and Protected
MATLAB allows you to control access to properties and methods using public, private, and protected keywords.
Here’s how you might define a class with encapsulated properties:
classdef BankAccount
properties (Access = private)
Balance
end
methods
function obj = BankAccount(initialBalance)
obj.Balance = initialBalance;
end
function withdraw(obj, amount)
obj.Balance = obj.Balance - amount;
end
end
end
In this example, `Balance` is kept private, ensuring that it can only be modified through methods defined within the class, maintaining the integrity of the account balance.

Best Practices for Using MATLAB OOP
Structuring Your Code
Effective OOP design in MATLAB requires a structured approach. Organize your classes logically, grouping related functionalities together to enhance readability and maintainability.
Naming Conventions
Adopting a clear naming convention improves code clarity. Use descriptive names for classes, methods, and properties that convey their purpose. For example, `getDogInfo` is more descriptive than `gDI`.
Documentation and Comments
Writing documentation and comments is crucial. It helps other developers (or future you) understand the thought process behind your code. Use comments judiciously to explain complex logic and provide documentation for public methods to guide users.

Conclusion
In concluding this guide on MATLAB Object-Oriented Programming, we’ve explored the foundational principles that enable you to write effective OOP code. By understanding and using classes, properties, methods, inheritance, polymorphism, and encapsulation, you can create robust applications that are easier to manage and extend. Embrace these concepts and practice regularly, as hands-on experience will solidify your understanding and help you become proficient in MATLAB OOP. Consider joining our MATLAB training sessions for more insights and personalized learning!