In MATLAB, "row oriented" refers to operations and data structures that focus on manipulating data along the rows of a matrix, making it easier to perform calculations and access subset elements by treating each row as a distinct entity.
% Creating a 3x3 matrix and accessing the second row
A = [1, 2, 3; 4, 5, 6; 7, 8, 9];
second_row = A(2, :); % Extracts the second row of the matrix A
disp(second_row); % Displays: 4 5 6
What is Row-Oriented Programming?
Row-oriented programming in MATLAB focuses on how data is organized and manipulated in a horizontal manner within matrices and arrays. Unlike column-oriented structures, where data operations predominantly take place along columns, row-oriented structures emphasize the significance of rows. This method aligns closely with MATLAB’s inherent design, which thrives on matrix manipulation.
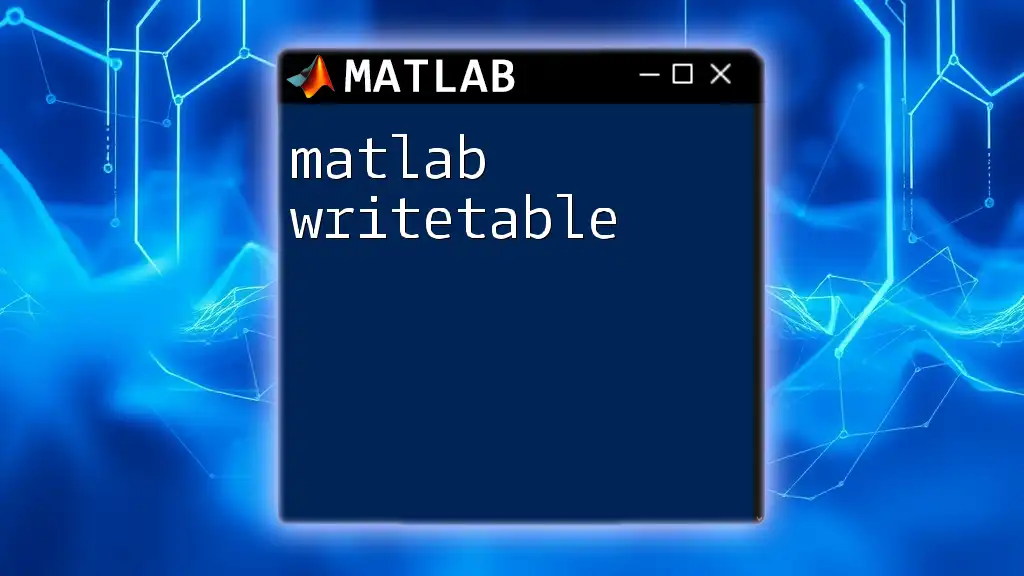
Benefits of Row-Oriented Programming
Engaging with row-oriented programming provides numerous advantages:
- Memory Efficiency: Processing smaller chunks of data aligns better with memory management, leading to improved performance.
- Simplicity in Data Manipulation: Operations can be intuitively performed on contiguous data sets.
- Enhancement of Computational Speed: Certain operations benefit from optimized memory access patterns, resulting in faster execution times.
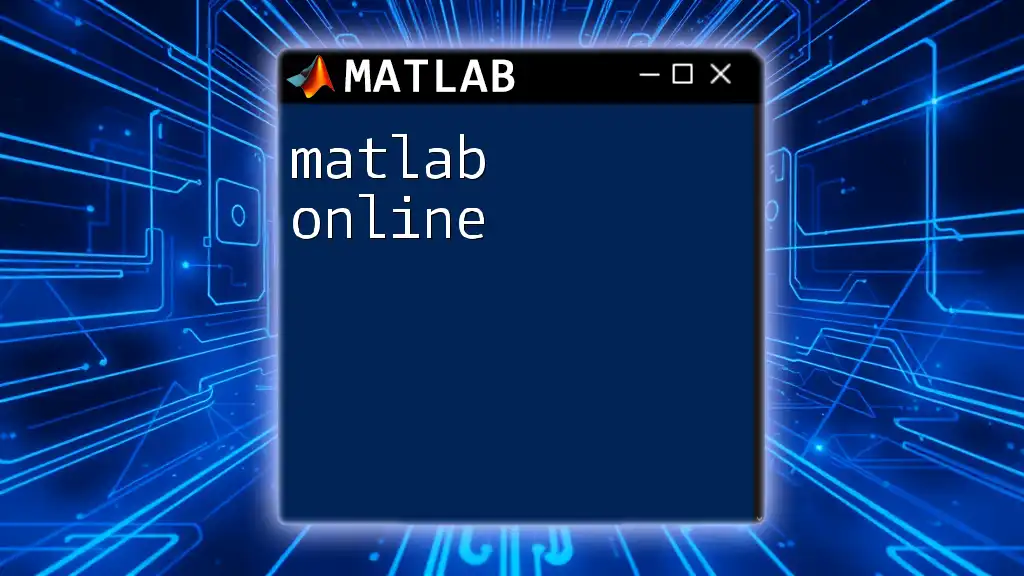
Understanding MATLAB Arrays and Rows
Basics of MATLAB Arrays
In MATLAB, arrays are foundational elements representing collections of data. They can store more than just numeric values; they can contain strings, structures, and even cells.
Types of Arrays
- Row Vectors: A one-dimensional array with a single row.
- Column Vectors: A one-dimensional array with a single column.
- Matrices: Two-dimensional arrays containing multiple rows and columns.
Creating Row Vectors
Row vectors can be created using various methods in MATLAB. The most common syntax involves enclosing elements in square brackets, separated by spaces or commas.
Syntax for Creating Row Vectors
For example, creating a simple row vector can be done as follows:
rowVec = [1, 2, 3, 4];
Using `linspace` and `colon` Operators
You can also generate row vectors using the `linspace` function, which creates evenly spaced values, or through the colon operator for defining ranges. Here are examples of both:
rowVec1 = linspace(1, 10, 5); % Creates a row vector with 5 evenly spaced values from 1 to 10
rowVec2 = 1:5; % Creates a row vector from 1 to 5
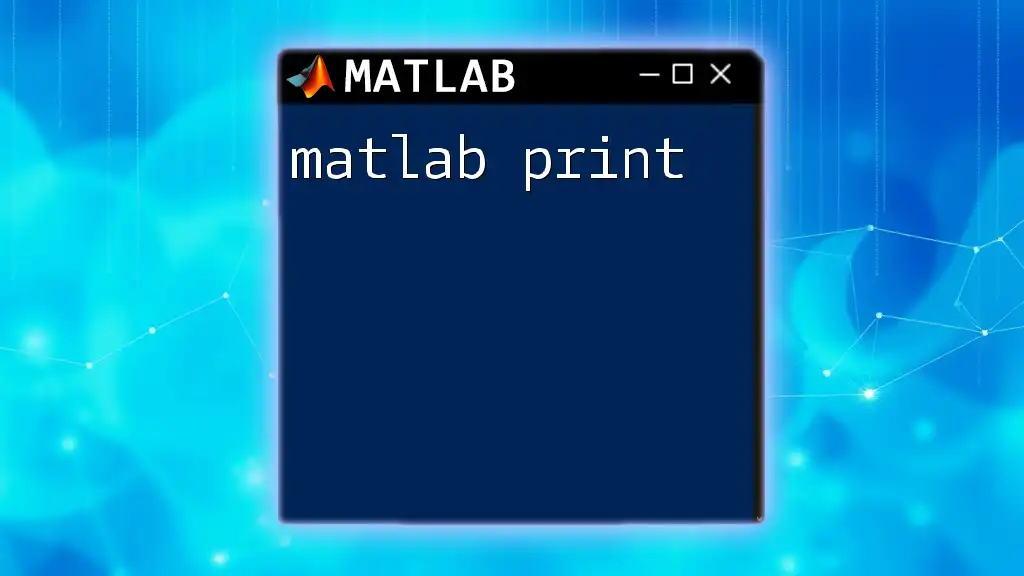
Fundamental Row Operations
Accessing Row Elements
Accessing specific elements in a row is straightforward using MATLAB’s indexing system, which supports both linear and logical indexing. This allows for efficient selection of data.
Indexing Rows
Consider a simple matrix:
A = [1, 2, 3; 4, 5, 6];
To access the first row, you can use:
row1 = A(1, :); % Accesses the entirety of the first row
Modifying Row Elements
Once you’ve retrieved a row, you might want to modify it. Changing values within a row is just as intuitive. For instance:
A(1, :) = [10, 20, 30]; % Modifies the first row to contain the new values
Adding and Deleting Rows
Appending Rows to a Matrix
To append a new row to an existing matrix, the `cat` function can be beneficial. For example:
B = [7, 8, 9];
A = [A; B]; % Appends the vector B as a new row
Removing a Row
Conversely, if you want to remove a row, use:
A(1, :) = []; % Removes the first row from the matrix
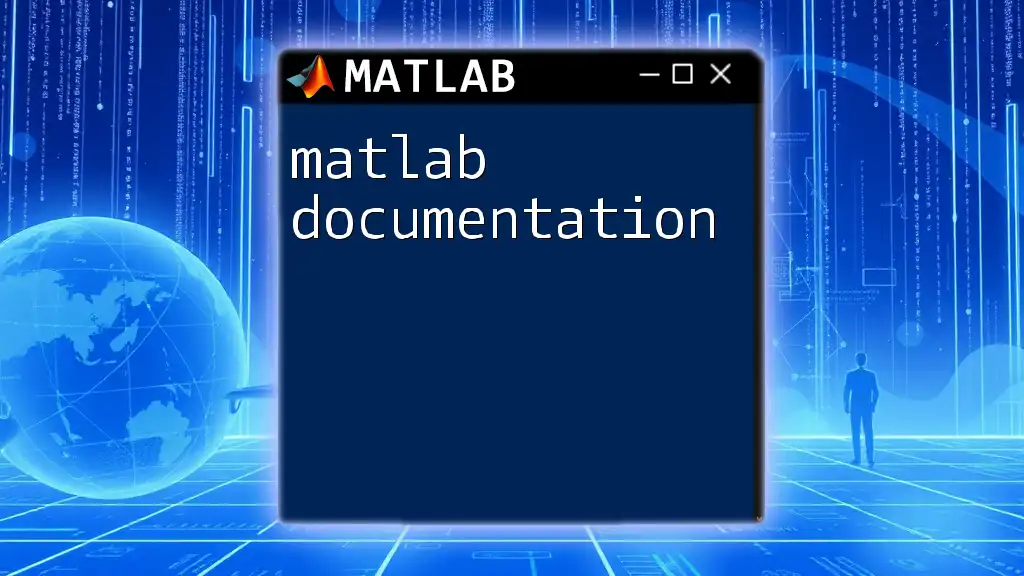
Advanced Row Operations
Row-wise Operations and Functions
MATLAB’s built-in functions can be applied row-wise, providing powerful tools for data manipulation and analysis.
Using Built-in Functions on Rows
To compute the sum or average of each row, you might use:
rowSum = sum(A, 2); % Returns the sum of each row
rowMean = mean(A, 2); % Returns the mean of each row
Logical Operations and Filtering
Leveraging logical conditions for filtering can significantly enhance data analysis. For example, if you want to select certain rows based on a condition, you can employ logical indexing:
filteredRows = A(A(:, 1) > 2, :); % Selects rows where the first column’s value is greater than 2
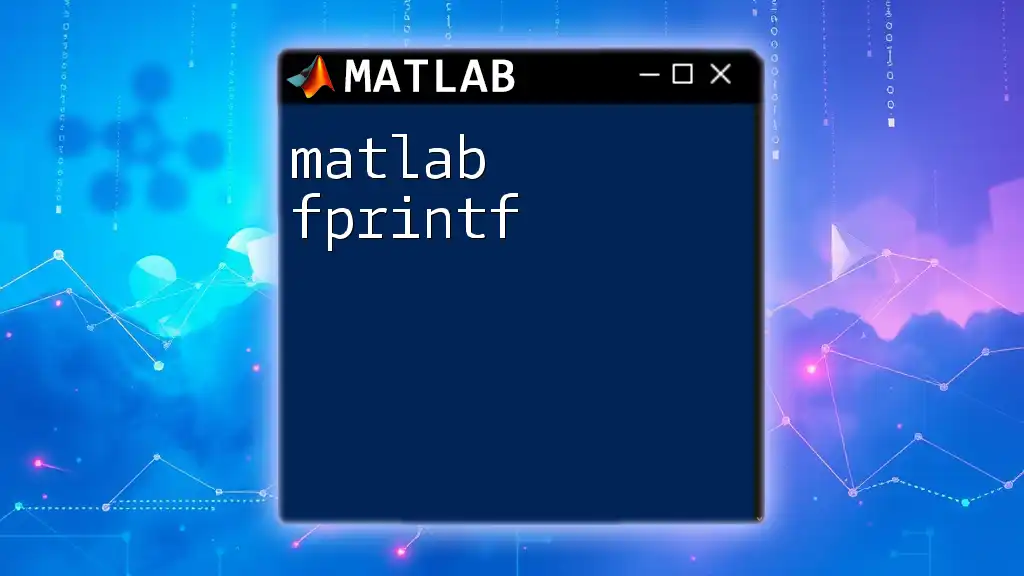
Practical Applications of Row-Oriented Programming
Data Manipulation with Row-Oriented Methods
Row-oriented programming is advantageous in real-life applications, particularly in areas such as data cleaning and preprocessing. Efficient manipulation of rows allows for effective handling of missing data, duplicates, and outliers.
Visualizing Row Data
Visual representation of row data can provide insights that are not readily apparent from raw numbers. For instance, using MATLAB’s plotting capabilities:
x = 1:10;
y = [sin(x); cos(x)];
plot(x, y); % Plots both sine and cosine functions on the same axes
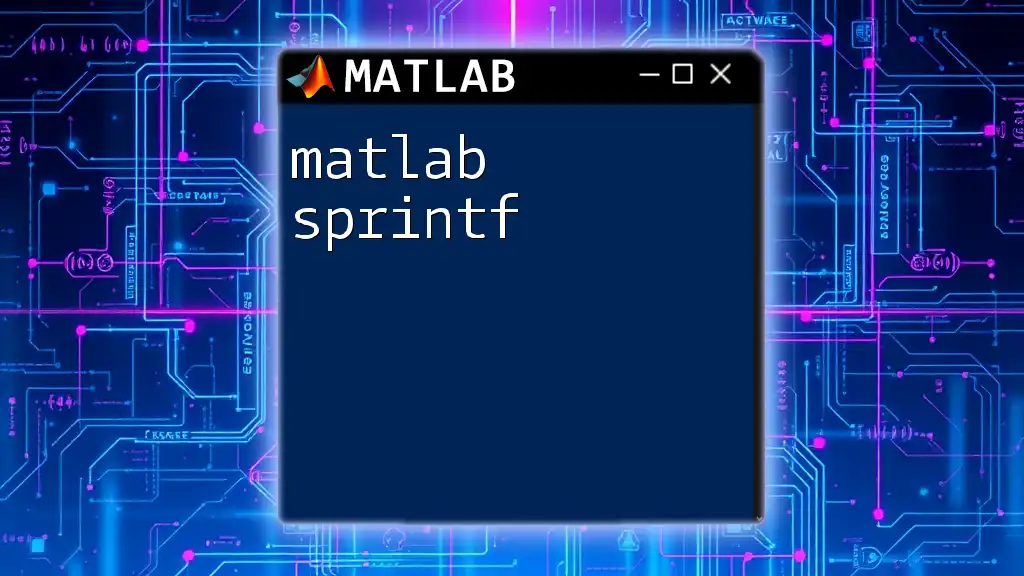
Recap of Key Takeaways
Understanding MATLAB's row-oriented capabilities allows users to leverage its powerful data manipulation features. From creating and modifying row vectors to implementing advanced operations, proficiency in these methods significantly enhances your programming toolbox.
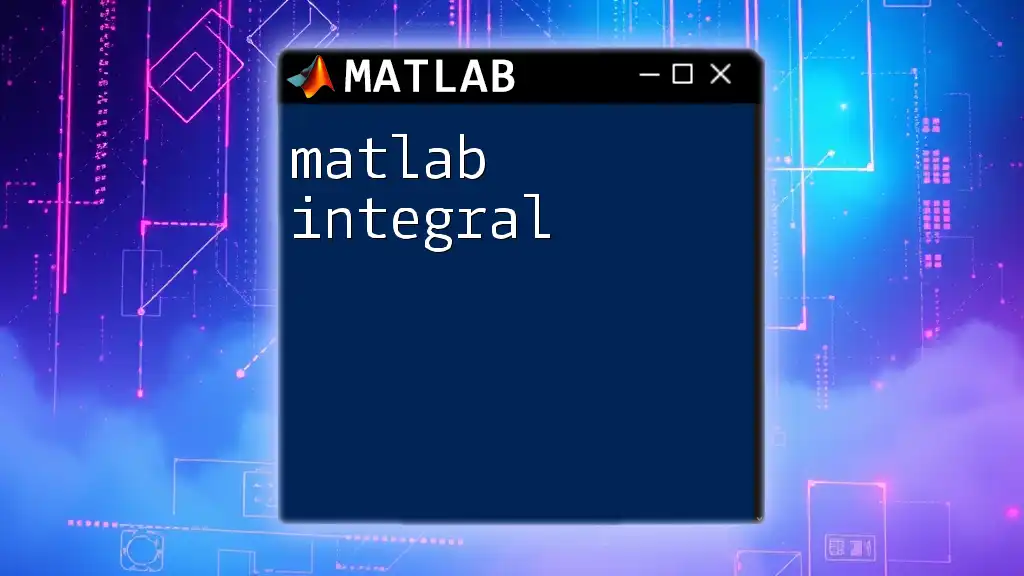
Encouragement for Further Exploration
The landscape of MATLAB is rich with possibilities waiting to be explored. Whether you're a beginner or an experienced programmer, practicing row-oriented programming can deepen your understanding and improve your data analysis skills. Continue experimenting and discovering the full potential of MATLAB!
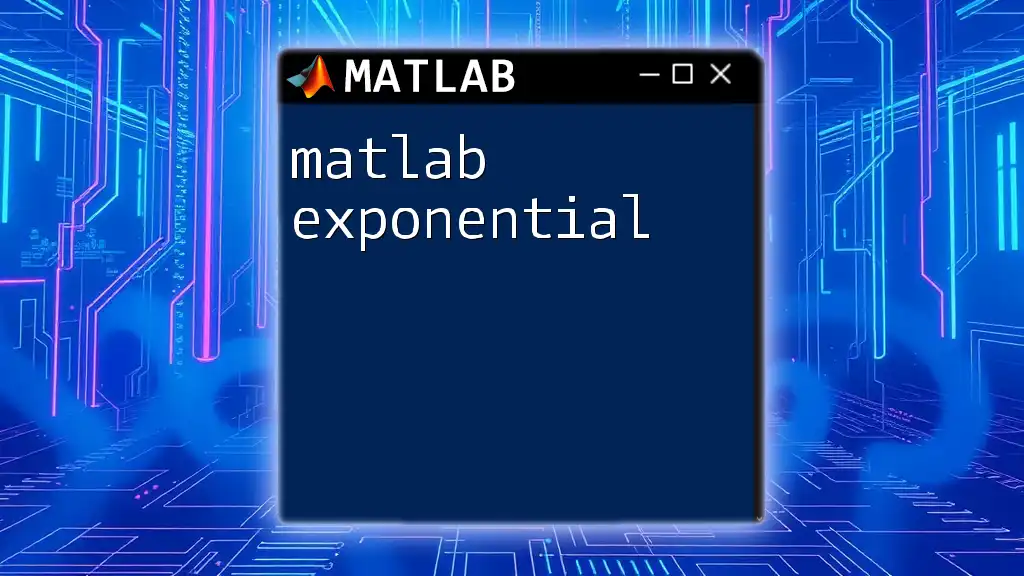
Useful Links and Documentation
For further learning, consult the [official MATLAB documentation](https://www.mathworks.com/help/matlab/) and engage in online forums and communities, where you can connect with other MATLAB users to share knowledge and tips.