If your MATLAB command window output is getting cut off when using the `publish` function, you can adjust the `OutputPath` and specifically set the `MaxColumns` property to ensure that your text is fully captured.
Here’s an example of how to use the `publish` function in MATLAB:
publish('your_script.m', 'OutputPath', 'your_output_directory', 'MaxColumns', 80);
Understanding the Publish Feature
What is the Publish Feature?
MATLAB’s publish feature allows users to automatically convert scripts into well-formatted documents in various formats, such as HTML or PDF. This functionality is particularly important for sharing work, documentation, and reproducibility of research. By utilizing the publish feature, you can enhance the clarity of your output and share comprehensive reports that incorporate both code and output in a structured manner.
Common Functions and Settings
To effectively use the publish feature, familiarity with key commands is essential:
publish('myScript.m'); % Basic syntax for publishing
This command takes your script, processes the comments and output, and formats them into a document. You can customize the publishing behavior through various options, making it a versatile tool for MATLAB users.
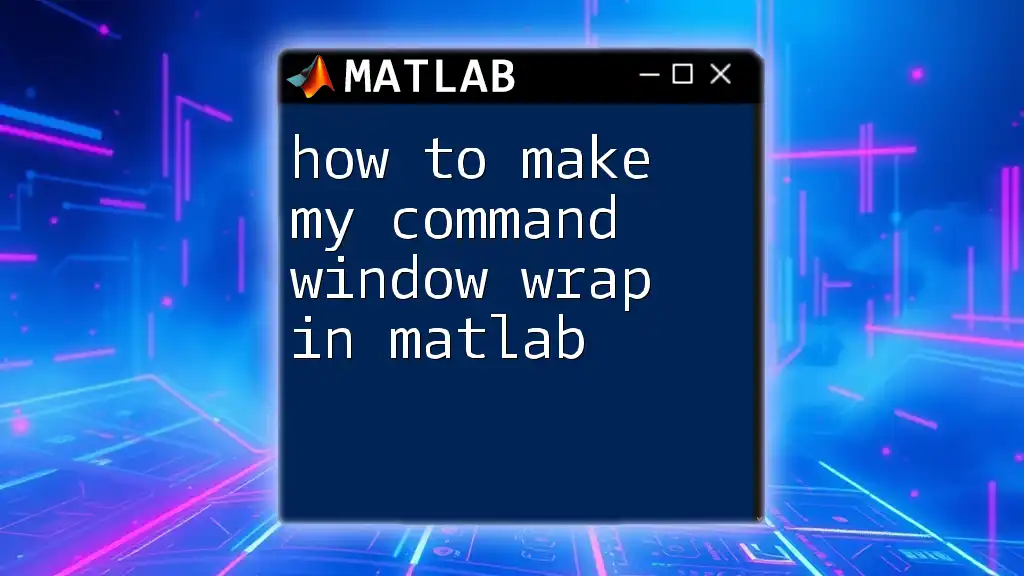
Diagnosing the Command Window Cutoff Issue
What Causes Cuts in the Command Window Output?
The most common cause for the output being cut off in the command window arises from its size limitations. When a script generates a significant amount of output, MATLAB may truncate the display, preventing users from viewing all results. Additionally, if your script does not handle lengthy outputs effectively, MATLAB may skip printing some results altogether.
Identifying the Problem
To identify if your outputs are being cut off, inspect the command window after running a script with extensive output. A classic example of potential truncation is using a loop that displays a large number of rows:
for i = 1:1000
disp(i); % This can lead to truncation
end
If you run this, you might notice that not all numbers are displayed, indicating the command window is unable to keep up with the volume of output being generated.
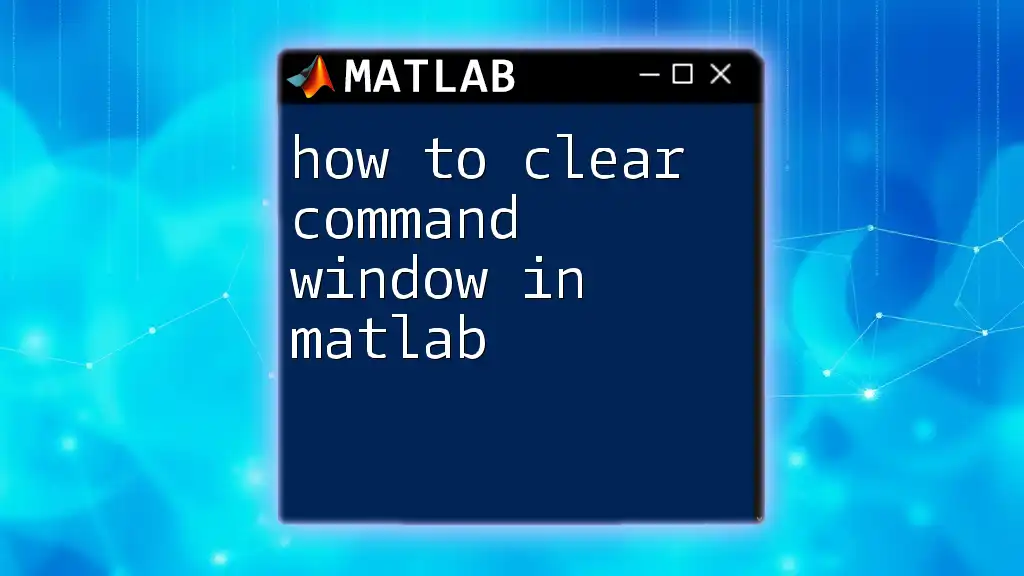
Solutions to Fix the Cutoff Issue
Adjusting the Output Length
Limiting your output to only show essential information can help prevent cutoff issues. Instead of displaying every single iteration, you might use the `disp` and `fprintf` functions effectively for controlled outputs, allowing you to manage what gets displayed:
for i = 1:100
fprintf('Value: %d\n', i);
end
This approach is more concise and maintains readability without overwhelming the command window.
Modifying Publish Options
To further customize the output when using the publish command, you can define specific options that enhance how results are displayed:
options.format = 'html';
options.outputDir = 'myOutputDirectory';
options.evalCode = true;
publish('myScript.m', options);
These options allow you to control the output format and directory, ensuring that your document appears as you intend and that no information is lost or cut off. Adjusting the `evalCode` option can also help you manage how code executes and reports results.
Splitting Outputs into Multiple Sections
If your calculation generates extensive output, consider breaking the output into manageable sections to improve clarity. MATLAB structures or loops can effectively do this:
chunks = 1:100;
for i = 1:length(chunks)
if mod(i,10) == 0
fprintf('Output chunk %d: %d\n', i, chunks(i));
end
end
This method not only minimizes truncation but also allows users to comprehend large data comprehensively.
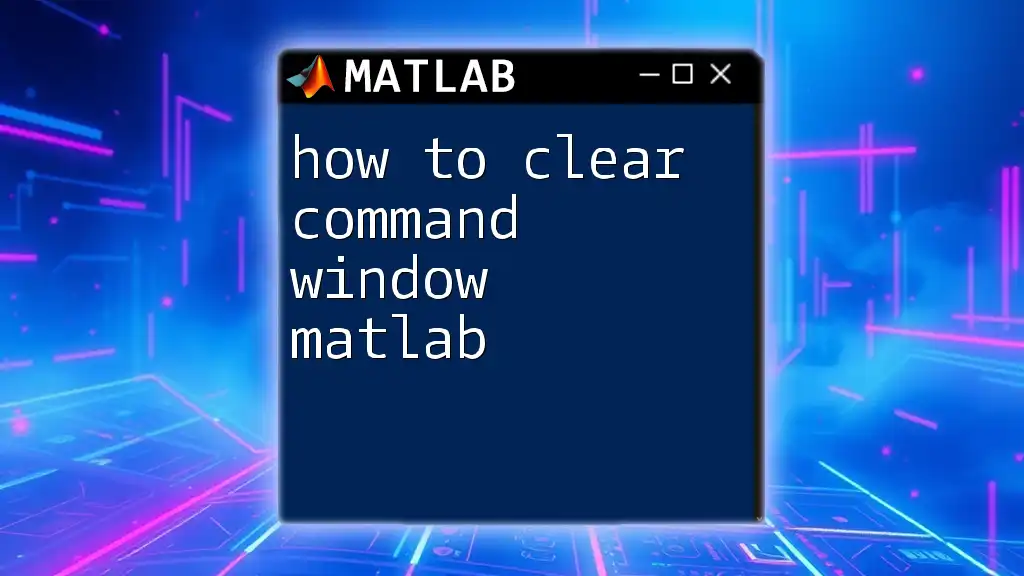
Enhancing the Command Window Output
Using Cell Arrays for Better Format
Using cell arrays can enhance the visual presentation of your output. For example, instead of printing each output individually, you can store them in a cell array and display them all at once:
results = cell(10, 1);
for i = 1:10
results{i} = sprintf('Result %d', i);
end
disp(results);
This transforms extensive outputs into a more compact and organized format, making it easier to read.
Improving Readability
Improving the readability of your output is crucial, especially when dealing with numerous results. Consider incorporating new lines or extra spacing between blocks of text to facilitate easy reading:
fprintf('First Line%sSecond Line\n', newline);
This function allows you to introduce whitespace that can separate distinct pieces of output and enhance the overall structure of your published documents.
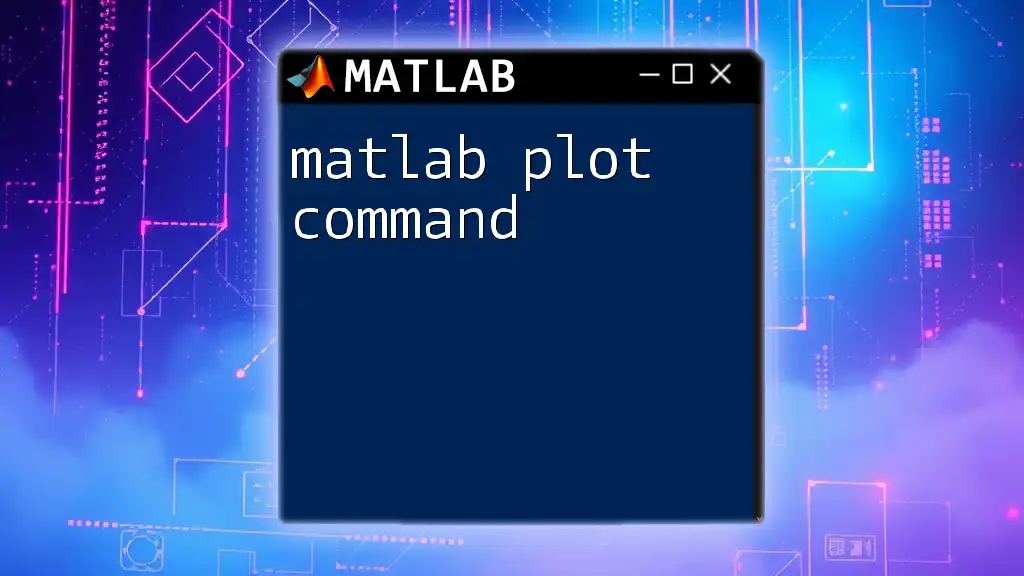
Best Practices for Publishing in MATLAB
Keeping Your Code Clean and Organized
Clean and organized code is fundamental for effective publishing. Practicing modular coding by breaking your scripts into functions improves reusability and maintainability. For instance, you might create a function to reset your command window before you run extensive outputs:
function clearDisplay()
clc; % Clear command window
end
Keeping your functions succinct and on-topic helps ensure a smoother publishing process.
Regular Updates and Testing
Maintaining and updating your publishing scripts is vital, especially as MATLAB updates its features and capabilities. Regular testing will help you identify issues before they affect your output. Always test your scripts in various environments to ensure consistency, especially if you work in teams or different machines.
Documentation Best Practices
Effective commenting and documentation improve the code's clarity and help others (or future you) understand your thought process. Always include comments explaining what your sections of code do:
% This loop displays numbers from 1 to 10
for i = 1:10
disp(i);
end
Clear comments can significantly enhance the understanding of your published output.
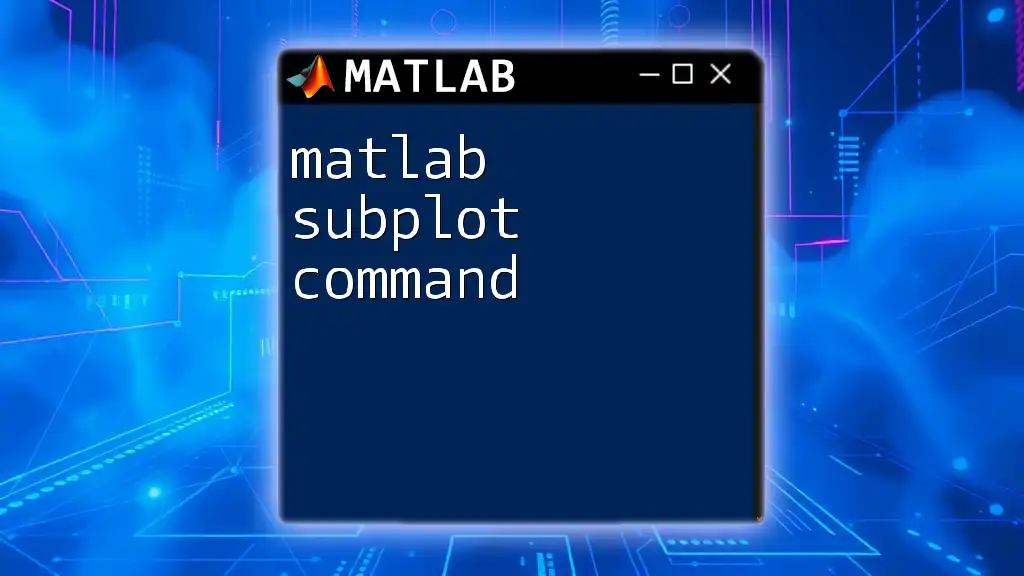
Conclusion
Addressing the issue of MATLAB truncating command window outputs through proper use of the publish feature is essential for anyone looking to present their work effectively. Experimenting with the various options available and employing best practices will ensure that your final output is not only functional but also visually appealing. Always remember to take the time to troubleshoot and adapt your workflows to minimize issues related to command window output truncation.
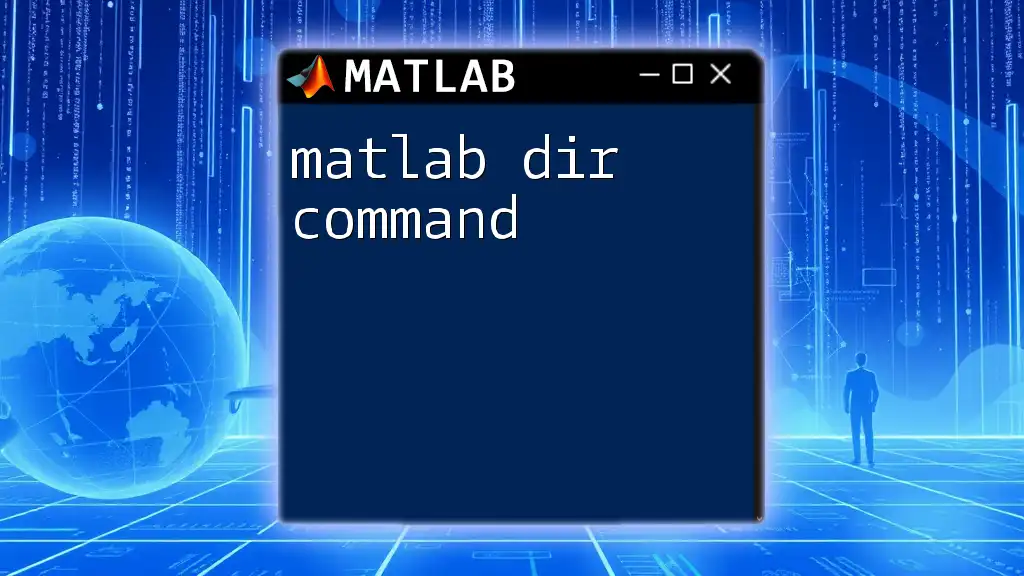
Additional Resources
Useful Links and References
- Official MATLAB documentation on publishing functionalities provides in-depth insights on how to maximize the potential of this feature.
- Online forums and MATLAB communities are excellent resources for troubleshooting and gathering tips from peers.
By keeping these suggestions in mind, you can create concise, clear, and effective documents that communicate your results without the hassle of cutoff areas in command window outputs.