MATLAB UI refers to the user interface functionalities in MATLAB that enable users to create interactive applications and graphical components for better data visualization and user interaction.
Here’s a simple example to create a basic UI with a push button:
f = figure('Position',[100 100 300 200]);
uicontrol('Style', 'pushbutton', 'String', 'Click Me', ...
'Position', [100 80 100 40], 'Callback', @buttonCallback);
function buttonCallback(~, ~)
disp('Button was clicked!');
end
Introduction to MATLAB UI
MATLAB provides powerful tools for creating user interfaces (UIs) that enhance user interaction and data visualization. A well-designed MATLAB UI allows users to manipulate data and view results seamlessly, making it an essential skill for anyone looking to perform data analysis or visualization in an interactive manner.
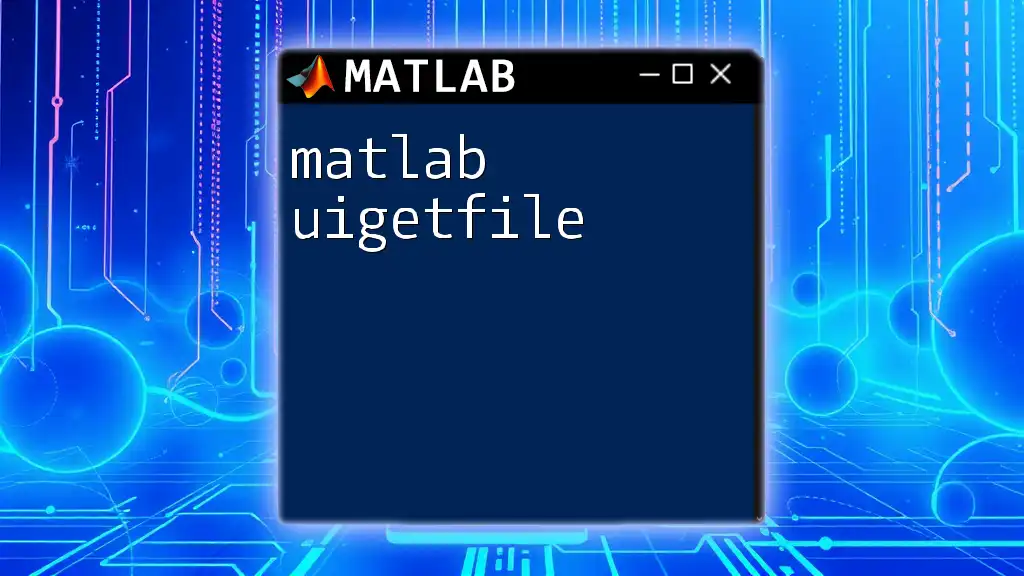
Choosing the Right UI Components
Understanding UI Components
In MATLAB, UI components are the building blocks of user interfaces. These can include buttons, text fields, panels, and more. Selecting the right components based on user needs is crucial for creating intuitive interfaces.
Common UI Elements
Buttons
Buttons are integral in MATLAB UIs for triggering actions. They can be customized in various ways, such as changing colors, sizes, and text labels. To create a simple button, you can use the following code:
uicontrol('Style', 'pushbutton', ...
'String', 'Click Me', ...
'Position', [100, 100, 100, 50], ...
'Callback', @myCallback);
function myCallback(~, ~)
disp('Button was clicked!');
end
Text Fields
Text fields are excellent for both input and output display. They allow users to enter text or display results. To add a text field to your GUI:
uicontrol('Style', 'edit', ...
'Position', [100, 50, 200, 30]);
Pop-up Menus and Dropdowns
Dropdowns or pop-up menus provide a way for users to select an item from a predefined list. This can reduce errors and streamline user interactions. Here is an example of creating a dropdown menu:
uicontrol('Style', 'popup', ...
'String', {'Option 1', 'Option 2', 'Option 3'}, ...
'Position', [100, 150, 100, 50]);
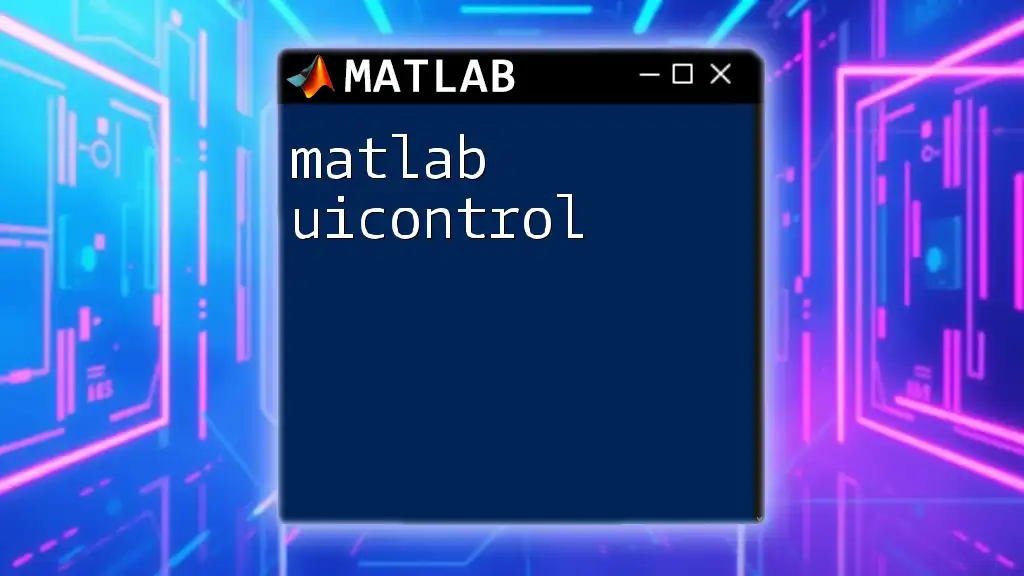
Building a Basic GUI in MATLAB
Using the App Designer
The App Designer is a modern environment for building MATLAB applications. It provides a drag-and-drop interface that streamlines the app creation process. The advantages of using App Designer include:
- An intuitive interface for designing UIs
- Integrated coding environment to manage callbacks
- Built-in support for deploying applications
Creating Your First App
To create a simple UI, follow these steps:
- Open App Designer from the MATLAB toolstrip.
- Choose a layout (e.g., a blank app).
- Drag components (like buttons and text fields) from the component library onto the design canvas.
- Set properties of each component using the right-side property inspector.
An example of creating a basic "Hello World" app:
uicontrol('Style', 'pushbutton', ...
'String', 'Say Hello', ...
'Position', [100, 100, 100, 50], ...
'Callback', @(~,~) disp('Hello, World!'));
Saving and Sharing Your App
Once you've created your app, saving it is straightforward through the File menu in App Designer. You can also share your app by exporting it as a standalone executable or packaging it in a .mlapp file for others to open in their MATLAB instances.
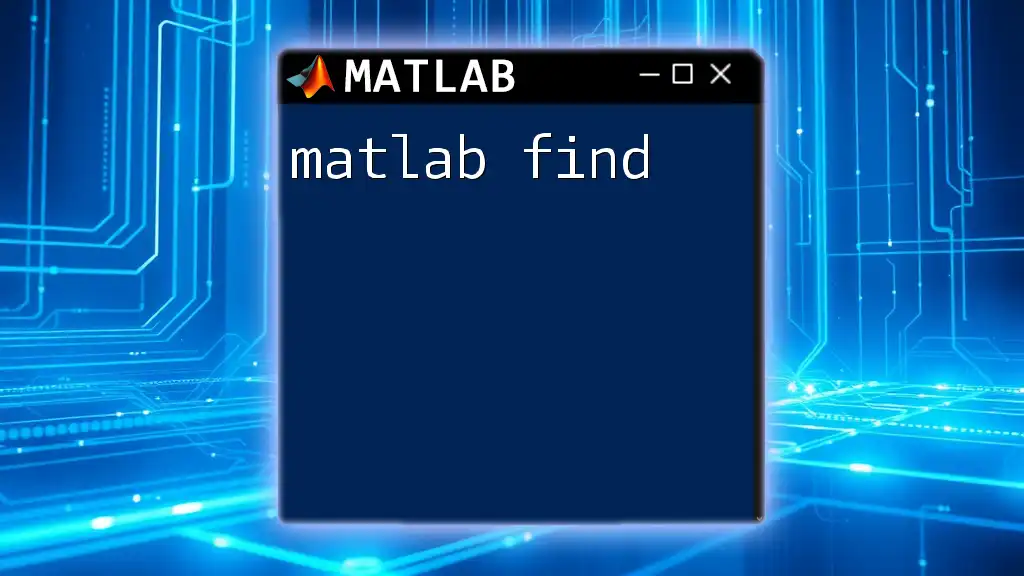
Advanced UI Techniques
Creating Complex Interfaces
Complex UIs often require nested panels and advanced layouts. By grouping related controls, you can create a more organized and understandable interface. Using grid and flow layouts can help maintain order. Here is an example of a more complex UI:
f = figure;
panel1 = uipanel('Position', [0.1, 0.1, 0.8, 0.8]);
uicontrol(panel1, 'Style', 'pushbutton', ...
'String', 'Panel Button', ...
'Position', [20, 30, 100, 40]);
Using MATLAB Components in Custom UIs
Integrating MATLAB figures into your UI is also possible. For example, displaying a plot can enhance data presentation:
axes('Position', [0.2, 0.2, 0.5, 0.5]);
plot(rand(1, 10));
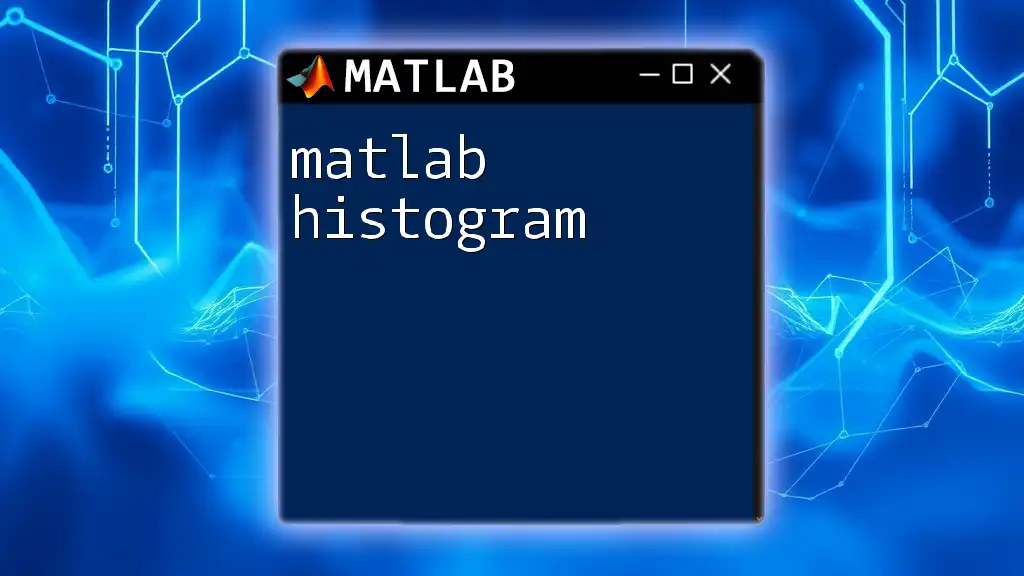
Enhancing User Experience
Customizing the Look and Feel
Customizing your UI's aesthetics enhances user experience. Changing colors, fonts, and component sizes can create a visually appealing interface. Consider using balanced color schemes that are pleasing to the eye and improve readability.
Adding Tooltips and Help Sections
User guidance is essential for effective interaction. Tooltips can provide instant help or hints regarding a component's function. You can add a tooltip using the `TooltipString` property:
uicontrol('Style', 'pushbutton', ...
'String', 'Help', ...
'TooltipString', 'Click for assistance');
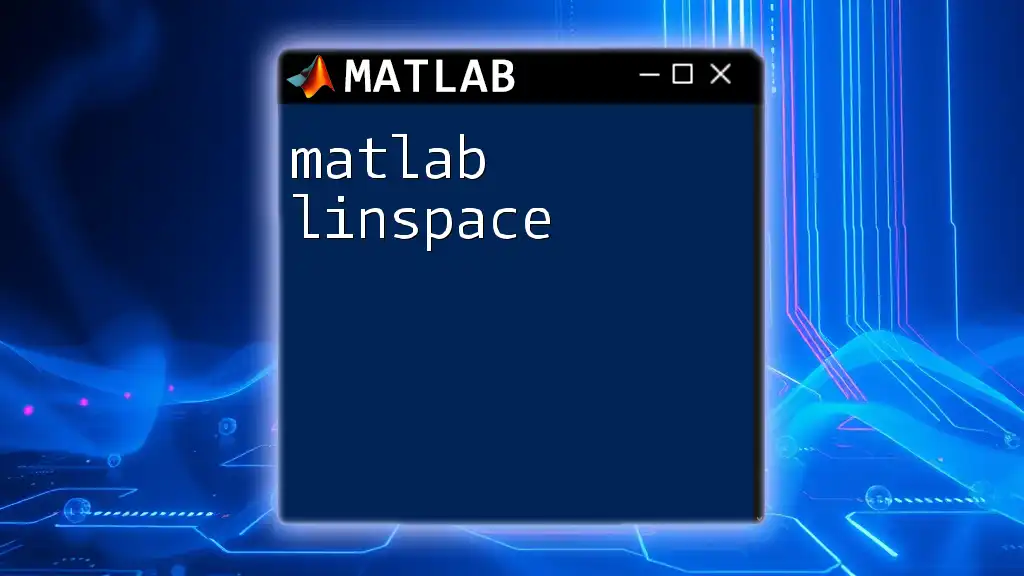
Debugging and Testing Your MATLAB UI
Common Issues When Developing UIs
Developing UIs can lead to various challenges, such as misaligned components or callback functions not executing as intended. Awareness of these common issues helps in troubleshooting effectively.
Debugging Tips and Best Practices
Using MATLAB's built-in debugging features is crucial for resolving errors. Setting breakpoints within callback functions allows you to step through code execution, revealing logical or runtime issues.
function myCallback(~, ~)
disp('Button clicked!'); % Set a breakpoint here
end
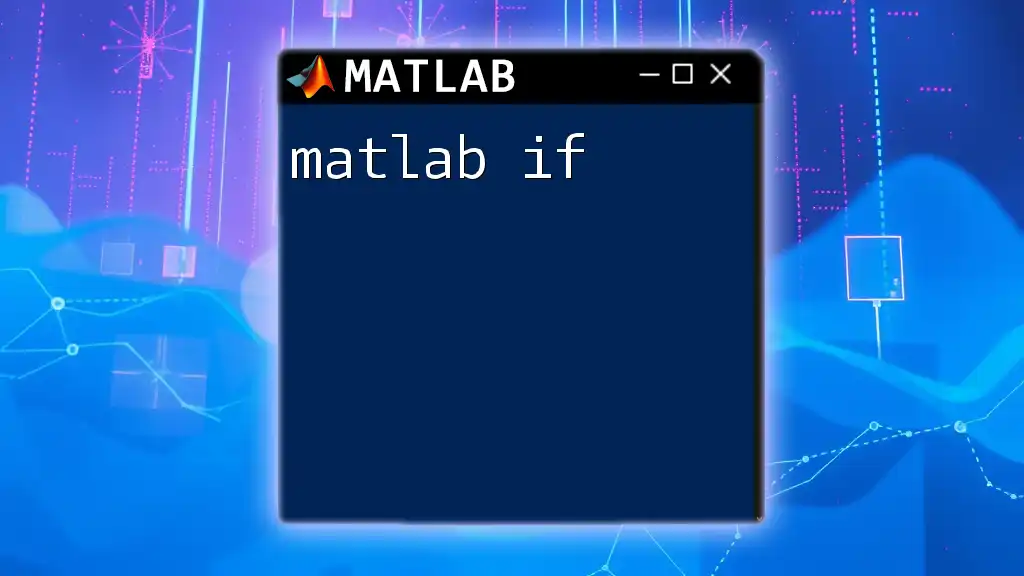
Real-World Applications of MATLAB UI
Using UI for Data Analysis and Visualization
The practical applications of MATLAB UI are extensive, especially in data analysis and visualization. An interactive data visualization tool can significantly enhance the analytical workflow, allowing users to explore data dynamically.
Developing Applications for Specific Fields
MATLAB UIs can cater to various domains, including engineering simulations, financial modeling, and educational tools. For instance, creating a UI for an engineering survey application allows users to input parameters and visualize results instantly.
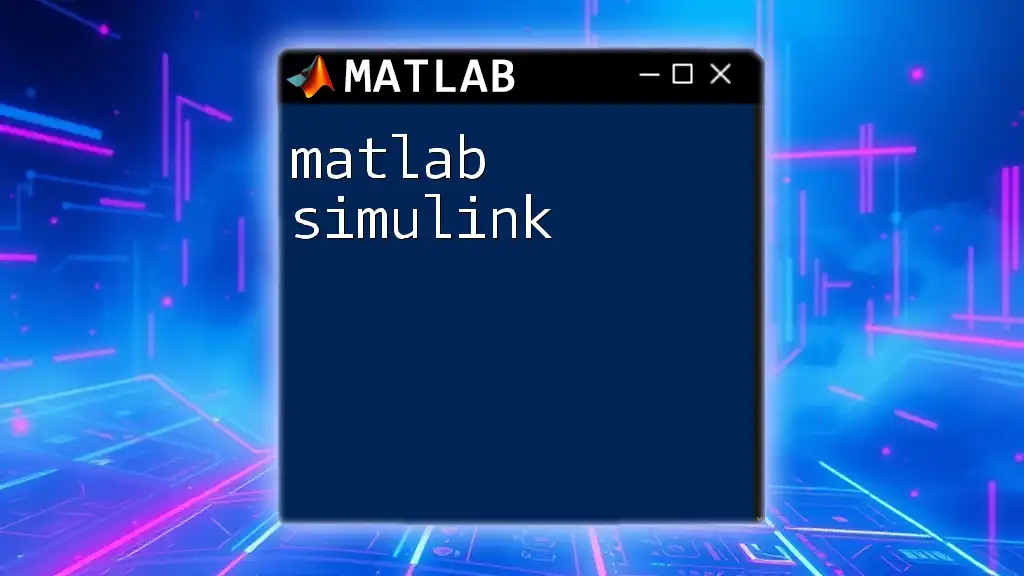
Conclusions and Final Thoughts
In summary, mastering MATLAB UI development can significantly improve user interaction with your applications. A well-designed UI is not just about aesthetics but also about functional usability that caters to user needs. As you dive deeper, experiment with various components, explore the App Designer, and build apps that resonate with users.
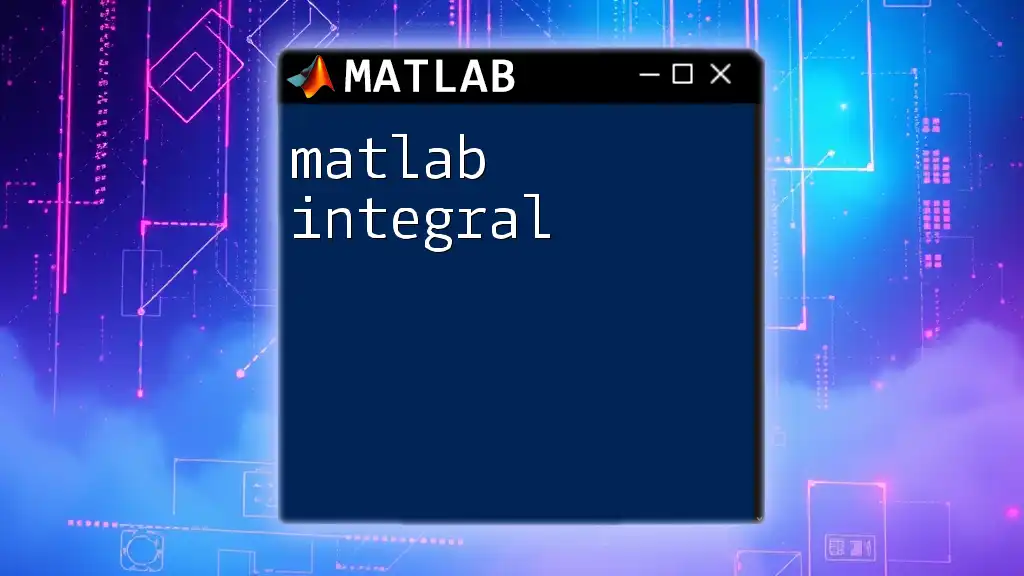
Additional Resources
For more in-depth learning and exploration of MATLAB UIs, utilize the official MATLAB documentation, which is invaluable for understanding the nuances of different components and their functionalities. Engaging with the MATLAB community through forums and discussions can also provide new insights and best practices.