Vehicle platooning in MATLAB refers to the use of MATLAB commands to manage and simulate the coordinated movement of multiple vehicles traveling in a closely spaced formation to enhance traffic efficiency and safety.
Here’s a simple MATLAB code snippet that initializes a platoon of vehicles:
% Define vehicle parameters
numVehicles = 5; % Number of vehicles in the platoon
desiredSpacing = 5; % Desired spacing between vehicles in meters
% Initialize positions and velocities
positions = zeros(numVehicles, 1);
velocities = 20 * ones(numVehicles, 1); % All vehicles start at 20 m/s
% Set initial positions based on desired spacing
for i = 1:numVehicles
positions(i) = (i-1) * desiredSpacing;
end
% Display initial positions and velocities
disp('Initial Positions:');
disp(positions);
disp('Velocities:');
disp(velocities);
Understanding Vehicle Platoon
What is Vehicle Platooning?
Vehicle platooning is an innovative transportation technique that involves a group of vehicles traveling closely together at high speeds, relying on automated systems to control distance, speed, and coordination. This method not only reduces air resistance and fuel consumption but also enhances road safety. The concept aids in minimizing traffic congestion and provides an efficient means of transport.
Platooning enables vehicles to communicate with one another, thereby making split-second decisions to maintain a safe distance. Some of the significant advantages of vehicle platooning include:
- Improved fuel efficiency due to reduced aerodynamic drag.
- Increased road capacity, enabling more vehicles to travel in the same space.
- Enhanced safety measures as vehicles can respond to each other’s movements quickly.
Applications in transportation systems are diverse; they range from freight transportation to passenger vehicles. The technology is vital for the development of autonomous driving, where vehicles can navigate and make decisions based on real-time communication.
Importance of MATLAB in Vehicle Platooning
MATLAB plays a crucial role in vehicle platooning scenarios by providing robust tools for modeling, simulation, and algorithm testing. Its user-friendly interface and powerful computational capabilities allow researchers and practitioners to simulate complex interactions among vehicles efficiently.
By utilizing MATLAB for vehicle platooning simulations, users can:
- Visualize vehicle behavior through real-time graphics that illustrate the dynamic movements within a platoon.
- Test various control algorithms and evaluate their performance under different conditions.
- Analyze and optimize designs with ease, paving the way for safer and more efficient platooning solutions.
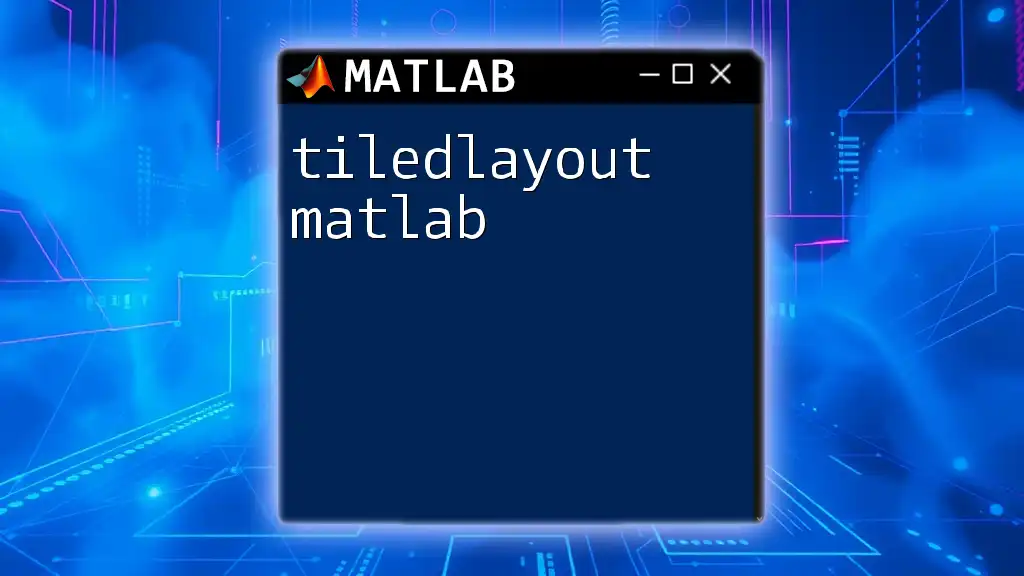
Setting Up Your MATLAB Environment
Downloading and Installing MATLAB
To get started with MATLAB, you’ll need to download and install it on your computer. Head over to the MathWorks website, and follow the instructions for your operating system. Ensure that your system meets the required specifications before initiating the installation process.
Key MATLAB Toolboxes for Vehicle Platooning
Several toolboxes enhance MATLAB's capabilities for vehicle platooning applications:
- Simulink: This is an essential toolbox for simulating dynamic systems. It offers a graphical block diagram environment conducive for vehicle modeling.
- Vehicle Network Toolbox: It enables communication simulation between vehicles, critical for testing platooning strategies in controlled scenarios.
Familiarizing yourself with these toolboxes is essential for leveraging MATLAB’s full potential in vehicle platooning.
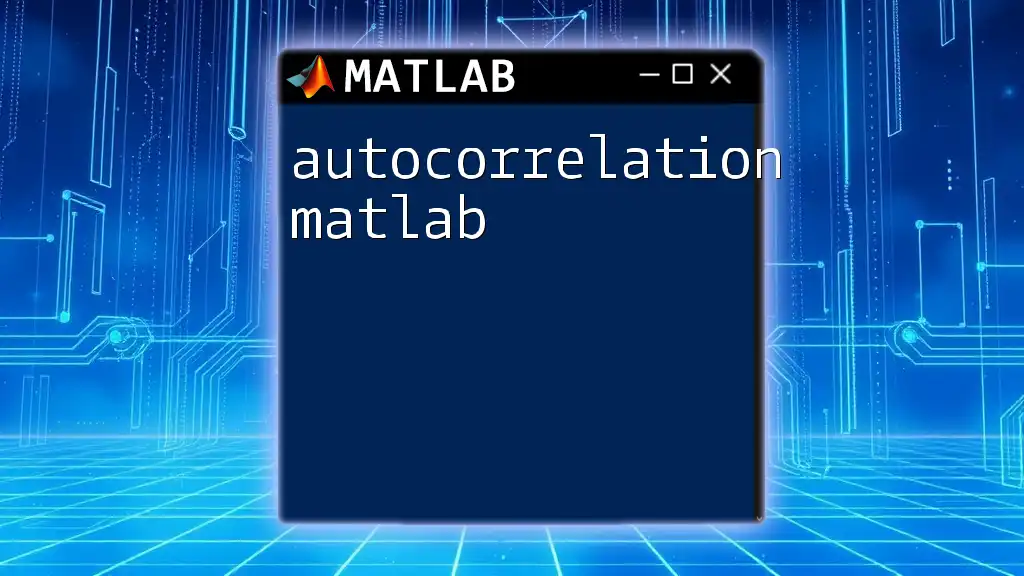
Basic Concepts of Vehicle Dynamics in MATLAB
Introduction to Vehicle Dynamics
Understanding vehicle dynamics is fundamental to modeling how vehicles behave under various conditions. Key factors influencing vehicle motion include acceleration, braking, road conditions, and inertia.
Modeling Vehicle Dynamics in MATLAB
Creating a basic vehicle model involves implementing the equations of motion, which describe the relationship between forces and the motion of a vehicle. A simple MATLAB script can illustrate this concept:
m = 1500; % mass of the vehicle in kg
a = 2; % acceleration in m/s^2
v = 0; % initial velocity in m/s
t = 0:0.1:10; % time from 0 to 10 seconds
% Calculate the velocity over time
v = v + a * t;
plot(t, v);
title('Vehicle Velocity over Time');
xlabel('Time (s)');
ylabel('Velocity (m/s)');
In this script, the mass and acceleration are defined, and the resulting velocity is calculated over a defined time frame. The graphical representation further aids in visualizing how velocity evolves.
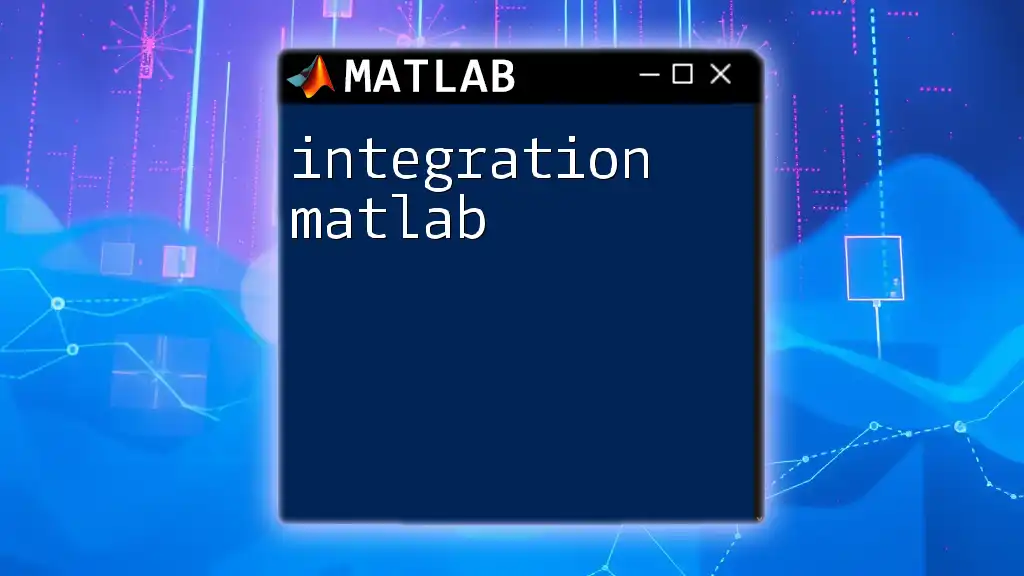
Implementing Vehicle Platooning in MATLAB
Basic Platooning Strategies
Several platooning strategies can be explored, such as maintaining a constant distance or adjusting velocity based on traffic conditions. It is essential to select a strategy that aligns with your objectives, whether ensuring safety or enhancing fuel efficiency.
Simulation Setup for Platooning
Setting up a basic simulation in MATLAB involves defining the initial conditions for each vehicle in a platoon. Here is a demonstration of how to initialize the positions of multiple vehicles:
% Initialize platoon vehicles
numVehicles = 5;
distanceBetween = 10; % meters
vehiclePositions = zeros(numVehicles, 1);
for i = 1:numVehicles
vehiclePositions(i) = (i-1) * distanceBetween;
end
This code snippet establishes the positions of five vehicles spaced 10 meters apart, setting the stage for simulating their interactions.
Controlling Vehicle Interactions
Controlling interactions among vehicles is vital for safe platooning. Implementing control algorithms, such as PID controllers, can help maintain desired distances.
For instance, a function for controlling a follower vehicle in relation to its leader might look like this:
function [force] = platoonControl(leaderPosition, followerPosition, desiredDistance)
error = (leaderPosition + desiredDistance) - followerPosition;
Kp = 1.5; % proportional gain
force = Kp * error;
end
The function computes the force needed for the follower based on the distance to the leader, enabling appropriate adjustments to be made in real time.
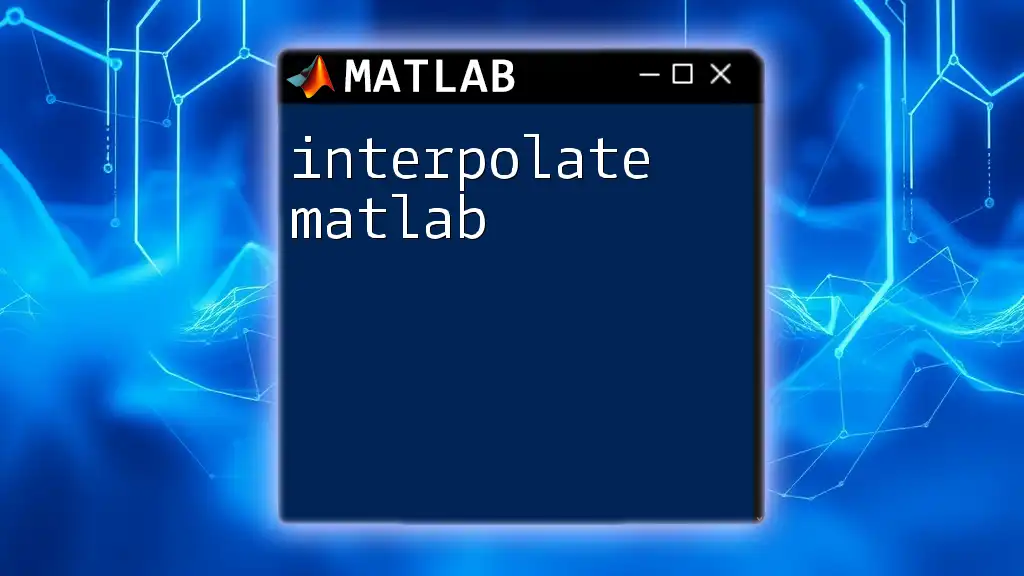
Visualization of Vehicle Dynamics and Platooning
Plotting Vehicle Movement in MATLAB
Visualization is key to understanding vehicle dynamics within a platoon. MATLAB provides powerful plotting functions to illustrate vehicle trajectories. An example of how to graph vehicle positions could be as follows:
plot(vehiclePositions, 'o-');
title('Vehicle Positions in Platoon');
xlabel('Vehicle Index');
ylabel('Position (m)');
This plot will offer a clear overview of the spatial arrangement of vehicles in the platoon.
Analyzing Results from the Simulation
After conducting simulations, analyzing the results is critical in assessing performance. Consider examining vehicle speed, the distance maintained, and response times to leader movements. This enables you to identify areas for improvement within your platooning strategy.
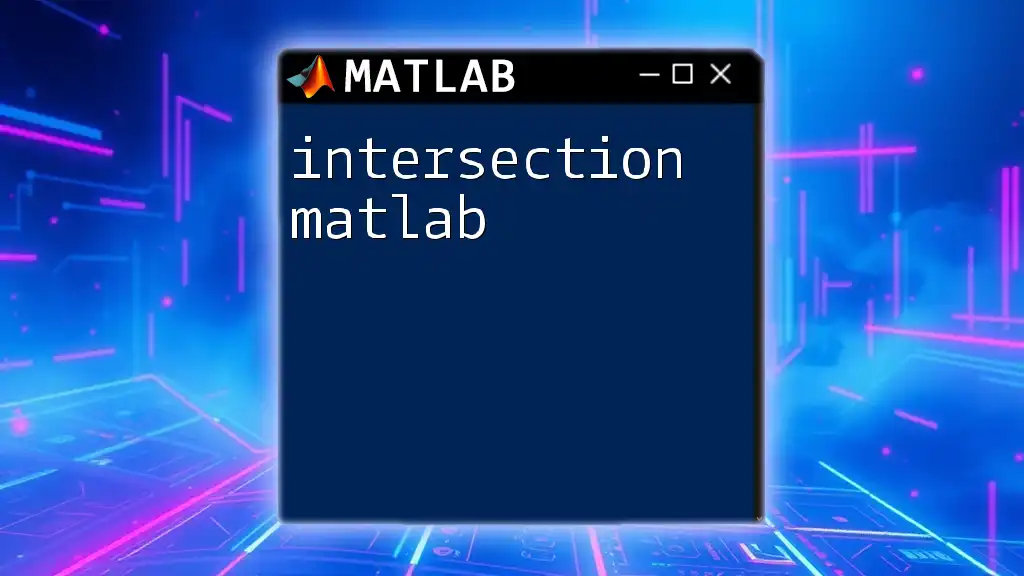
Advanced Topics in Vehicle Platooning
Cooperative Control Algorithms
Cooperative control strategies play a significant role in multi-vehicle platooning. These strategies allow vehicles to work together cohesively, responding to changes in their environment collaboratively. For example, consensus algorithms can be employed, allowing vehicles to achieve agreement on course adjustments based on shared information.
Challenges and Solutions in Platooning
Platooning does come with its challenges, including communication latency and potential errors in vehicle-to-vehicle communication. Addressing these challenges often involves developing robust error-handling protocols in simulations. Using MATLAB, you can model these scenarios to test the resilience of your platooning strategies under adverse conditions.
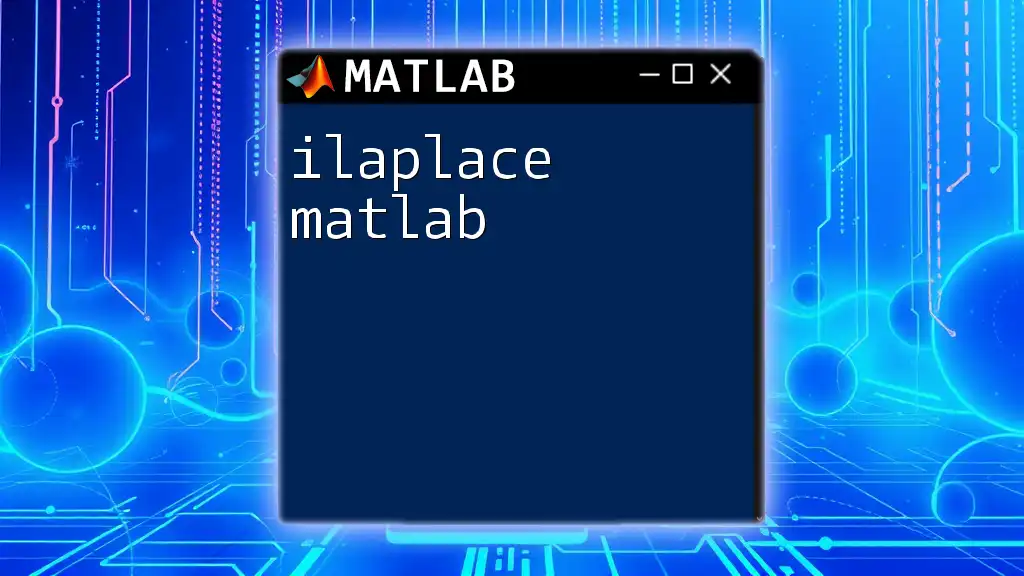
Conclusion
Summary of Key Takeaways
In this guide, we explored the concept of vehicle platooning and its advantages. We established the importance of MATLAB for simulating and modeling these systems. We delved into basic vehicle dynamics, simulation setups, control strategies, and visualization techniques.
Next Steps
To further your knowledge, consider exploring books and online courses focused on vehicle dynamics, control theory, and MATLAB programming. Applying what you've learned in practical projects will deepen your understanding and keep you engaged with this exciting field.
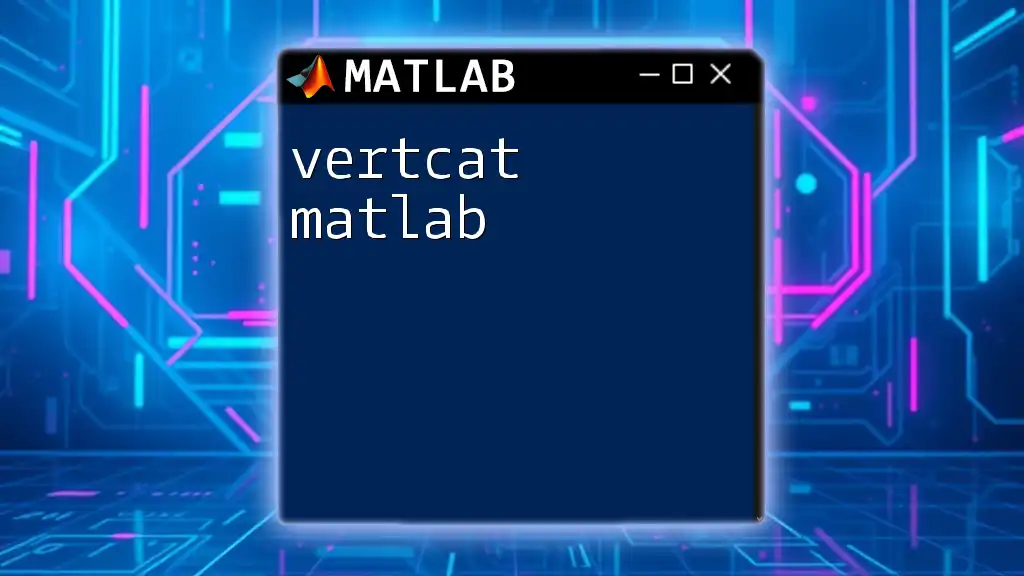
References
Recommended Reading and Resources
Broadening your knowledge base is essential; seek out literature and online resources related to vehicle platooning and MATLAB, which will provide more in-depth insights and advanced methodologies.
MATLAB Community and Support
Engaging with communities such as MATLAB Central or Stack Overflow can provide additional support and foster collaboration with other enthusiasts in the field of vehicle platooning.