A cubic spline in MATLAB is a piecewise polynomial function that provides a smooth interpolation between data points, ensuring that the first and second derivatives are continuous at each point.
Here’s a simple example of how to use cubic splines in MATLAB:
% Sample data points
x = [1, 2, 3, 4, 5];
y = [1, 4, 9, 16, 25];
% Create a cubic spline interpolation
spline_fit = spline(x, y);
% Evaluate the spline at finer points for a smooth curve
xx = linspace(1, 5, 100);
yy = ppval(spline_fit, xx);
% Plotting the results
plot(x, y, 'o', xx, yy, '-');
title('Cubic Spline Interpolation');
xlabel('x');
ylabel('y');
legend('Data Points', 'Cubic Spline');
What is a Cubic Spline?
A cubic spline is a piecewise polynomial function that ensures smoothness within a set of given data points. It consists of multiple cubic polynomial segments joined together, with each segment defined between two successive data points. One of the most notable features of cubic splines is their ability to maintain continuity not just in the function itself, but also in its first and second derivatives. This characteristic makes cubic splines a powerful tool in data interpolation and smoothing.
Using cubic splines is particularly advantageous when you need to model data that shows variability without losing the natural curves present in the dataset. Compared to other interpolation methods, such as linear interpolation, cubic splines yield smoother and more visually appealing results, which can effectively represent the underlying trends within the data.
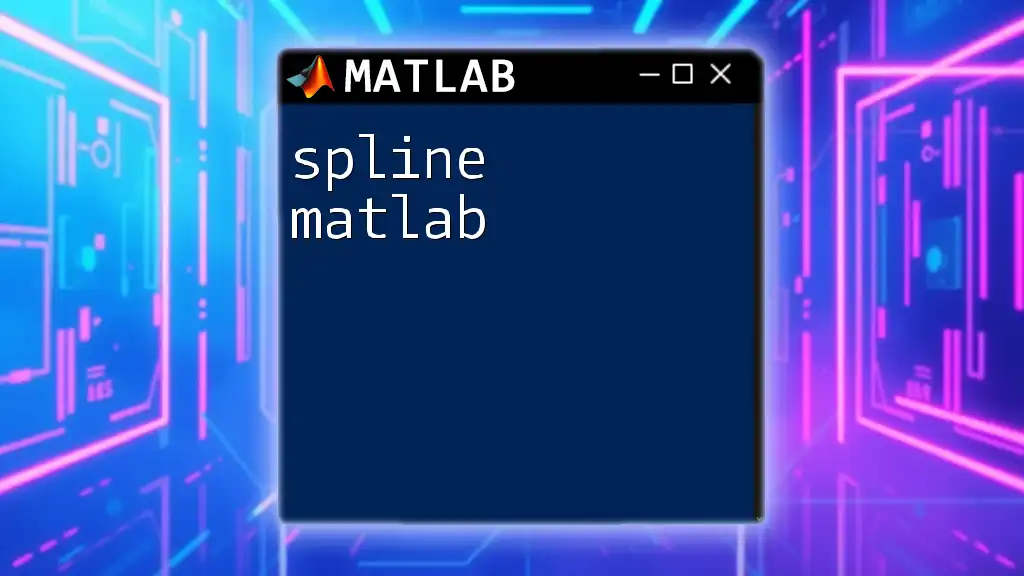
Why Use MATLAB for Cubic Splines?
MATLAB is an excellent platform for implementing cubic spline interpolation due to its powerful built-in functions, intuitive syntax, and ease of visualization. Specifically, for the cubic spline MATLAB implementation, the language provides functions that allow users to easily define, compute, and visualize splines without delving into complex mathematical coding.
Moreover, MATLAB’s array operations and plotting capabilities facilitate quick analyses in fields such as engineering, science, and finance. Its ability to handle large datasets efficiently makes it an invaluable tool for professionals needing to analyze or model data trends.
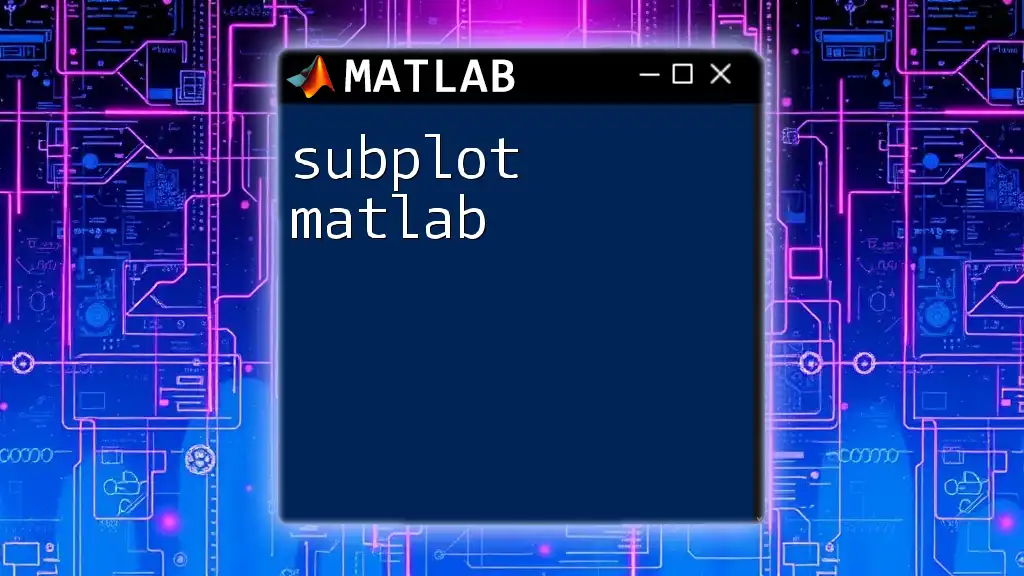
Setting Up Your MATLAB Environment
Installing MATLAB
Before diving into cubic spline implementation, ensure MATLAB is installed on your device. Check the system requirements on the official MathWorks website and follow the installation steps meticulously. Once installed, you can start by creating a new project designed for spline analysis.
Essential MATLAB Commands for Beginners
Familiarize yourself with some fundamental MATLAB commands that will be useful before implementing cubic splines. Understanding the MATLAB interface will help ease the learning curve. Key areas to focus on include data manipulation commands, plotting functions, and the differences between scripting and interactive commands.
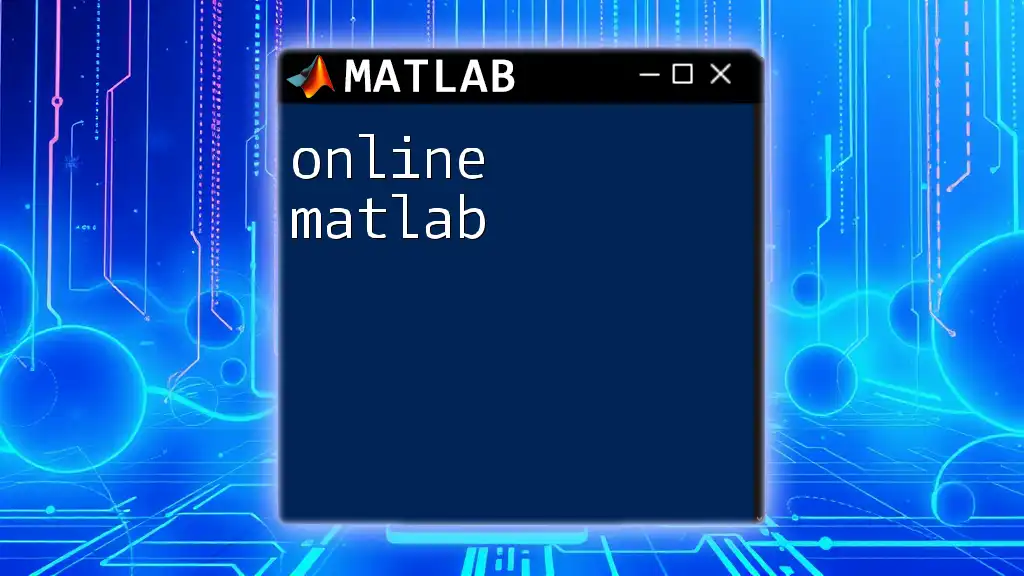
Understanding the Basics of Spline Interpolation
Types of Splines
Splines can be categorized into different types based on the degree of their polynomial segments. While there are linear, quadratic, and cubic splines, the cubic spline stands out due to its balance between flexibility and smoothness. Cubic splines minimize oscillations and deviations from the original data points, making them ideal for smooth interpolations.
How Cubic Splines Work
Cubic splines consist of polynomial functions that are defined piecewise over the intervals between data points. The key features of cubic splines include the following:
- Each segment is a cubic polynomial.
- The resulting spline is continuous at each data point, ensuring a smooth transition from one segment to another.
- The first and second derivatives are also continuous, which prevents sharp turns or breaks in the curve.
Understanding the mathematical foundation of cubic splines will enhance your ability to implement and troubleshoot them effectively.
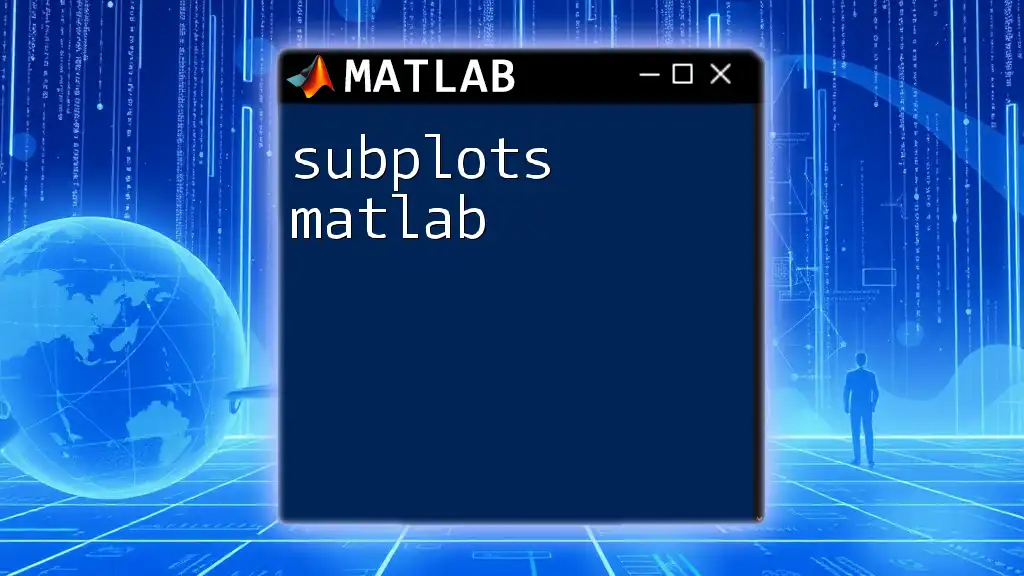
Implementing Cubic Splines in MATLAB
Preparing Your Data
Before implementing cubic splines, you must prepare your data in MATLAB. Here’s an example dataset:
x = [1, 2, 3, 4];
y = [2.2, 2.8, 3.6, 4.5]; % Example data points
Ensure that your data is formatted in vectors — one for the x-values and one for the y-values.
Using the `spline` Function
MATLAB provides a built-in function called `spline`, which simplifies cubic spline interpolation. The basic syntax follows a straightforward approach. Here’s how you can use it:
pp = spline(x, y);
In this code snippet, `pp` represents the piecewise polynomial form of the cubic spline. This polynomial can further be evaluated at any point using the `ppval` function, which makes it easy to conduct real-time evaluations.
Visualizing the Cubic Spline
Visualization is crucial for understanding how well your spline fits the data. Here’s how to plot your original data alongside the cubic spline:
xx = linspace(1, 4, 100);
yy = ppval(pp, xx);
plot(x, y, 'o', xx, yy, '-');
title('Cubic Spline Interpolation');
xlabel('X-axis');
ylabel('Y-axis');
In this plot, the circles represent the original data points, while the line reflects the cubic spline interpolation. This visual representation aids in diagnosing how well the spline models the data.
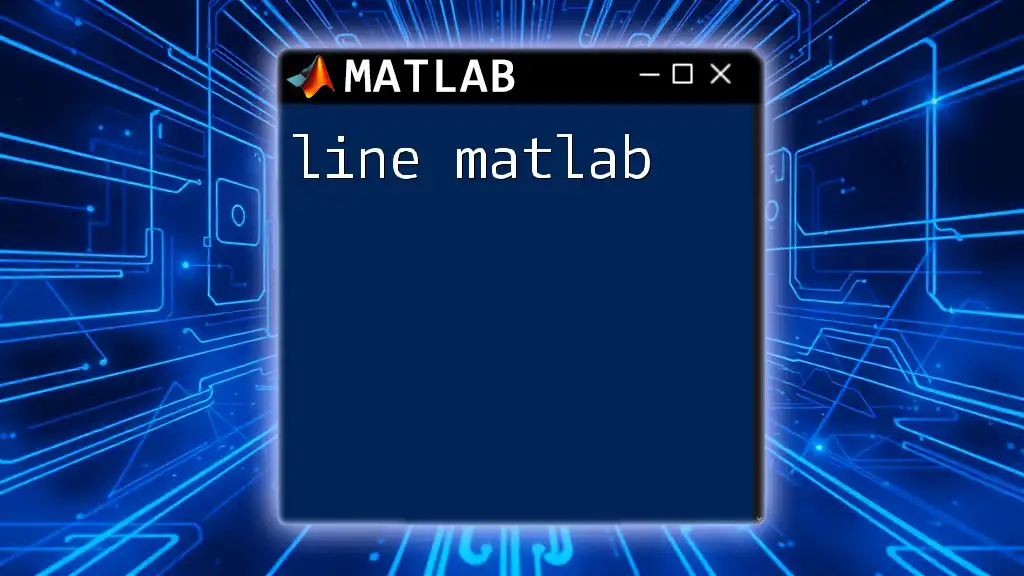
Advanced Techniques in Cubic Spline Interpolation
Natural and Clamped Cubic Splines
Natural cubic splines assume that the second derivative at the endpoints is zero, which leads to a more relaxed fit. In contrast, clamped cubic splines specify the first derivative at the endpoints for stricter control over the spline’s behavior.
Here’s how to implement both types in MATLAB:
% Natural Cubic Spline
pp_natural = spline(x, y);
% Clamped Cubic Spline Example
% Define first derivative at endpoints
dydx = [1, 1]; % Derivative values
pp_clamped = spline(x, [y(1), dydx(1), y, dydx(end), y(end)]);
Understanding these types of splines allows you to select the approach that best suits your data’s characteristics.
Error Analysis in Spline Interpolation
When implementing cubic splines, it’s essential to perform an error analysis to assess the interpolation’s accuracy. By comparing the spline’s output against known values or other interpolation methods, you can quantify the error. Visualization of the error can also provide insights into where the spline might be underperforming or overfitting.
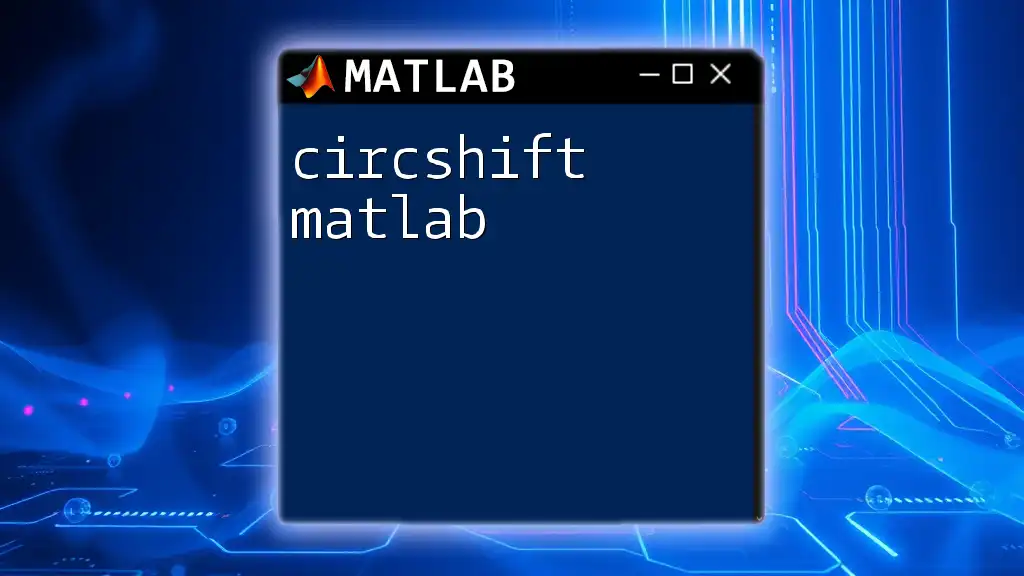
Applications of Cubic Splines
Engineering and Physics
Cubic splines are widely used in engineering and physics for modeling data that follows a non-linear trend. For instance, when fitting curves to road profiles or bridge designs, cubic splines offer a smooth representation that can be pivotal in analysis and simulations.
Finance and Economics
In the finance sector, cubic splines find applications in modeling yield curves or pricing options by providing a smooth estimation over various maturity dates. This helps traders and analysts visualize market trends more effectively.
Data Science and Machine Learning
In the realm of data science, cubic splines assist in regression problems, helping analysts interpolate missing values or model non-linear relationships between variables. Numerous case studies demonstrate how cubic splines elegantly handle diverse datasets.
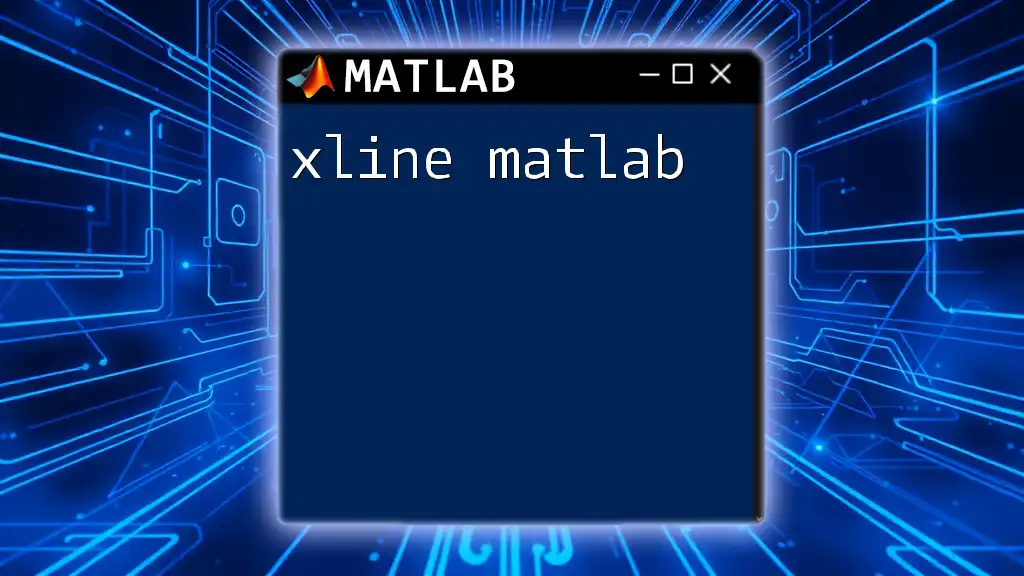
Troubleshooting Common Issues
Common Errors and How to Fix Them
During the implementation of cubic splines in MATLAB, you may encounter common errors, such as dimension mismatches or out-of-range evaluations. To troubleshoot:
- Ensure that your input vectors are of equal length.
- Double-check indices when using evaluation functions like `ppval`.
Tips for Improving Spline Performance
To enhance the performance of your cubic spline interpolation:
- Carefully select your dataset, as overly noisy data may lead to an inaccurate spline.
- Consider refining your spline’s boundary conditions for better fit and accuracy.
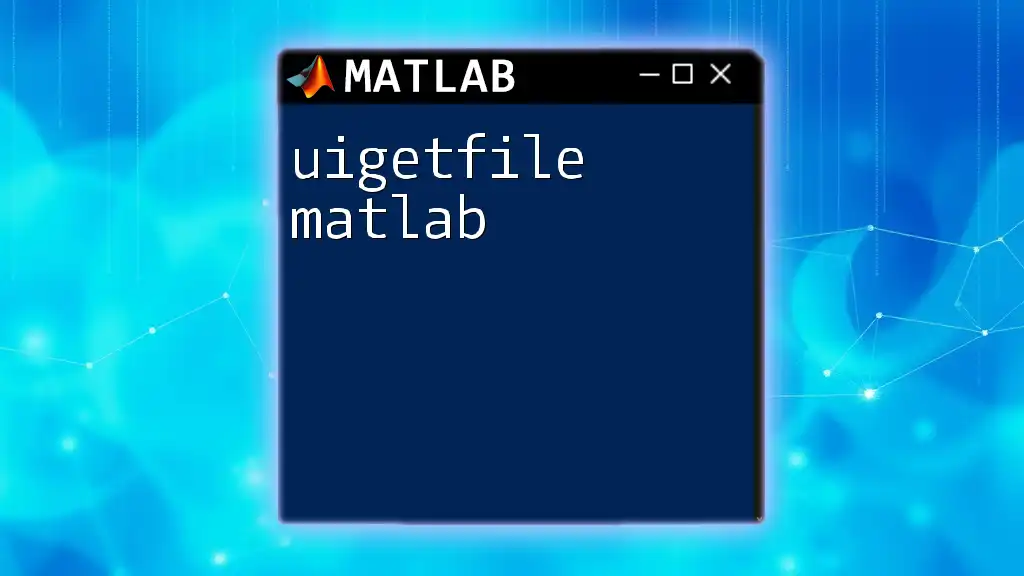
Conclusion
Cubic splines in MATLAB offer a robust approach to interpolation and data modeling. By understanding both the theoretical background and practical implementation, you can leverage the power of cubic splines in your analyses. By utilizing the built-in functions and visualization capabilities of MATLAB, you can transform complex data sets into elegant models that provide insights into underlying trends.
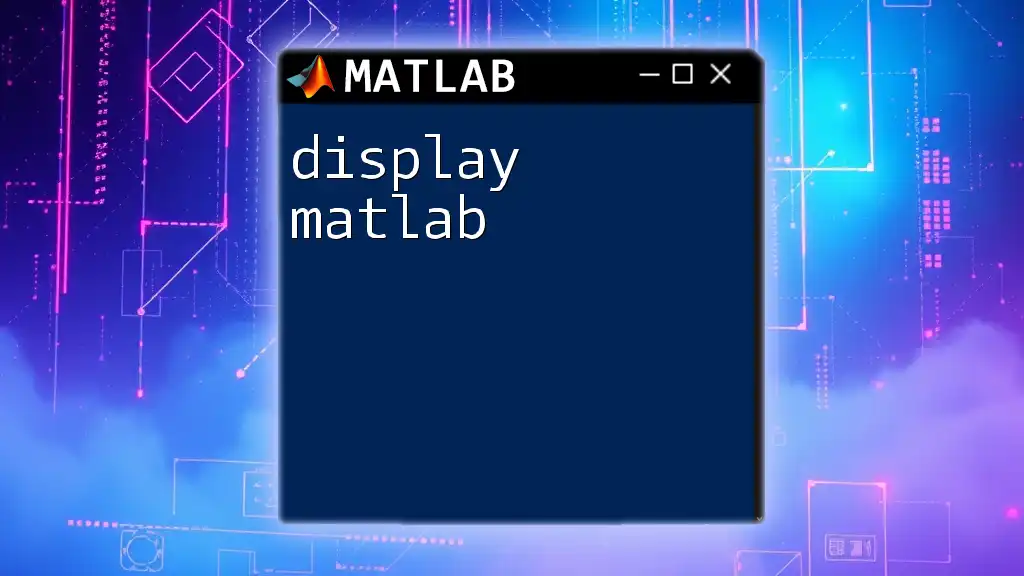
Further Resources
For those eager to learn more about cubic splines and MATLAB, an array of resources awaits. Recommended reading materials, MATLAB's official documentation, and online forums are excellent avenues to deepen your understanding and get support.
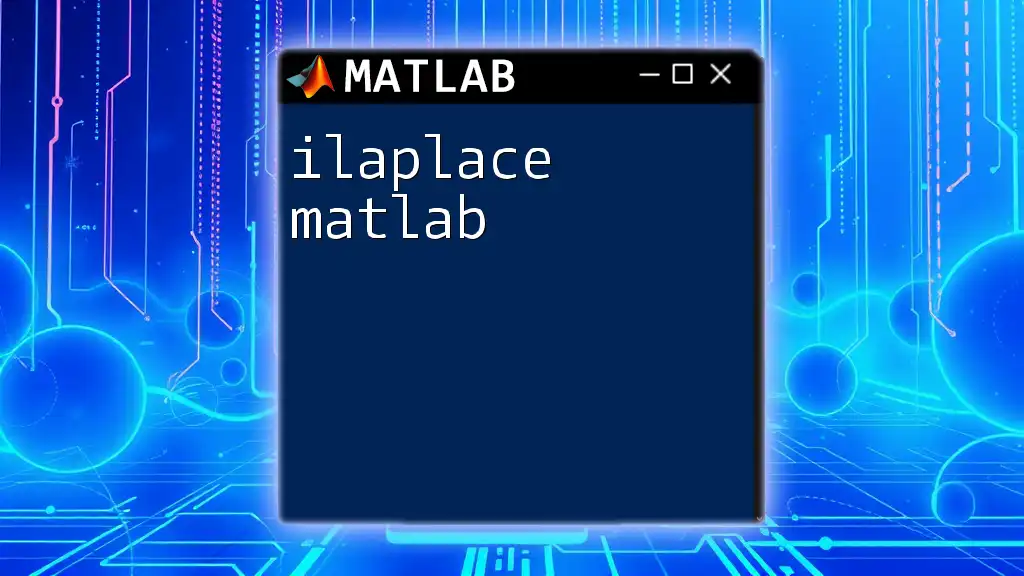
Call to Action
Try implementing cubic spline interpolation in MATLAB today! Whether you are an engineer, data scientist, or financial analyst, mastering this technique will significantly enhance your analytical skills. Additionally, consider signing up for our upcoming workshops to delve deeper into MATLAB commands and techniques that will elevate your programming proficiency.