A low frequency filter in MATLAB can be created using a Butterworth filter design to effectively remove high-frequency noise from a signal.
[b, a] = butter(3, 0.1, 'low'); % Design a 3rd-order low pass Butterworth filter with 0.1 normalized frequency
filtered_signal = filter(b, a, original_signal); % Apply the filter to the original signal
What Are Low Frequency Filters?
Low frequency filters are vital tools in signal processing that allow signals with a frequency lower than a certain cutoff frequency to pass through while attenuating frequencies higher than the cutoff. These filters are crucial in various applications, from audio signal processing to communications and biomedical engineering. By using low frequency filters, engineers and researchers can remove unwanted high-frequency noise and focus on the relevant low-frequency components of a signal.
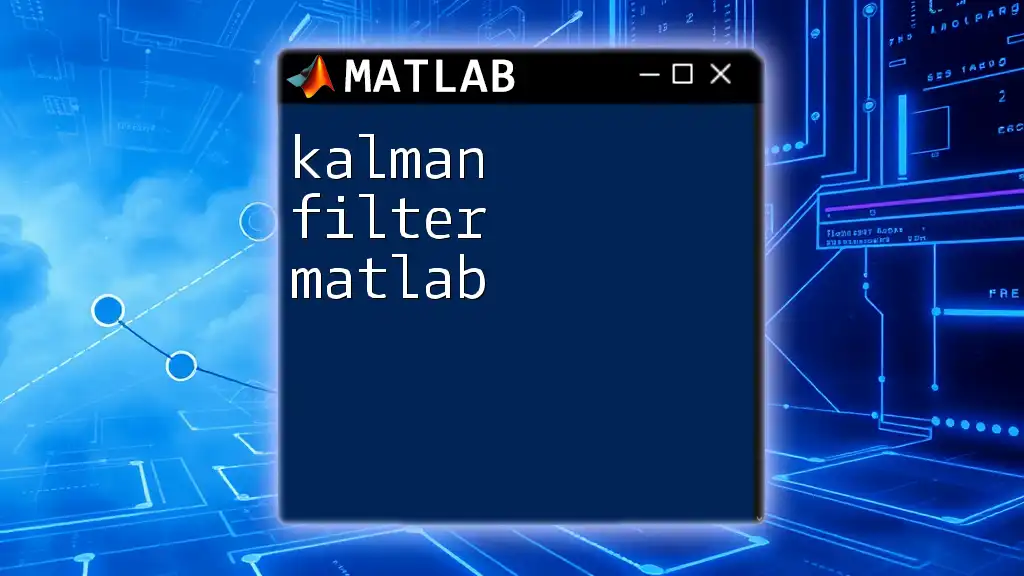
Types of Low Frequency Filters
Active vs. Passive Filters
Filters can be classified as either active or passive.
-
Active filters use external power sources, allowing for improved performance and greater design flexibility. They often employ operational amplifiers for gain and can achieve more complex filtering characteristics.
-
Passive filters, on the other hand, consist of passive components such as resistors, capacitors, and inductors. While simpler and less costly, passive filters cannot provide gain and have certain limitations in terms of performance.
Analog vs. Digital Filters
Another categorization is based on the nature of signal processing:
-
Analog filters operate on continuous-time signals. While effective, their implementation can be challenging in modern applications.
-
Digital filters, implemented in software such as MATLAB, process discretized signals. Digital filter design offers versatility and precise control over filter characteristics, making them a preferred choice in many applications.
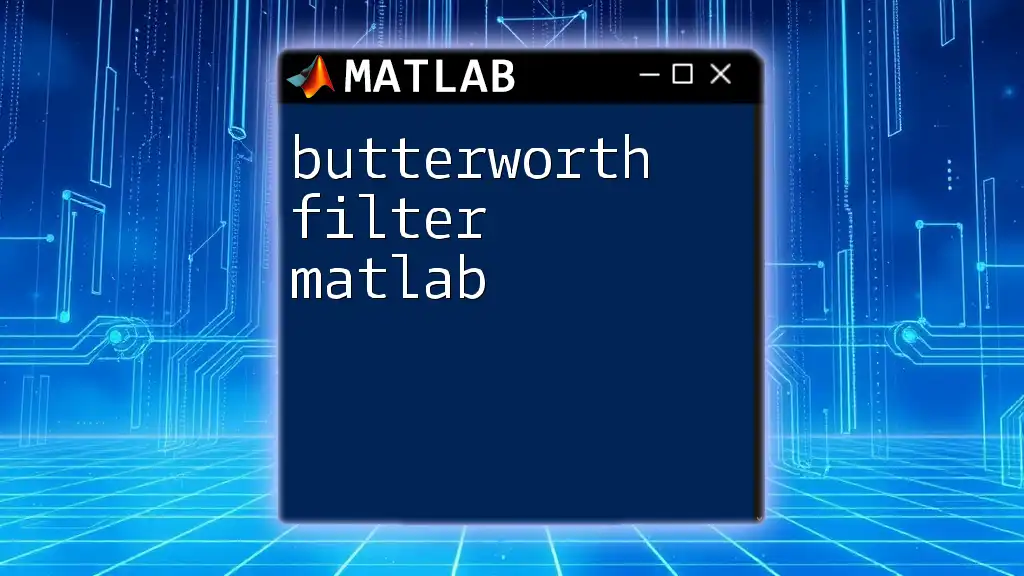
Understanding the Basics of Filtering in MATLAB
Signal Processing Concepts
Before diving into filter design in MATLAB, it's essential to grasp some fundamental signal processing concepts. Understanding the difference between the time domain and frequency domain is key, as it allows you to analyze how a signal behaves both in its original state and after being modified by a filter.
Additionally, concepts like sampling rate play a critical role in filter design. The sampling rate determines how often a signal is sampled and affects the filter's performance.
MATLAB's Signal Processing Toolbox
MATLAB provides a powerful Signal Processing Toolbox that includes functions and tools specifically designed for filtering and analyzing signals. Utilizing this toolbox will enable you to create, visualize, and apply low frequency filters efficiently.
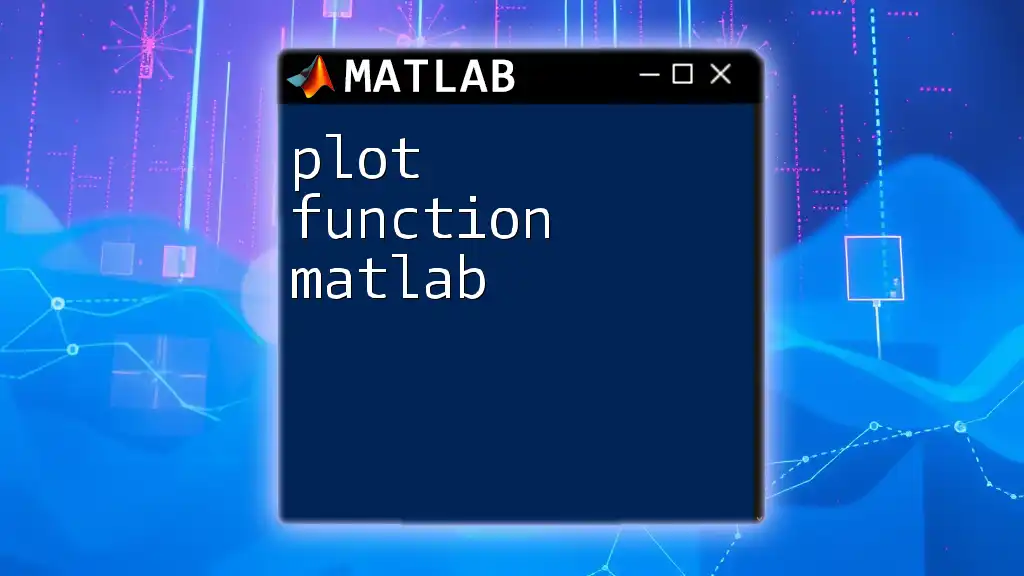
Designing a Low Frequency Filter in MATLAB
Filter Specifications
When designing a low frequency filter, it's important to define certain specifications:
- Cutoff frequency: This is the frequency at which the filter begins to attenuate frequencies beyond this point.
- Order of the filter: Higher-order filters have sharper roll-offs and better performance but may be more complex to implement.
- Filter type: Depending on the application, you may choose from various filter types such as Butterworth, Chebyshev, or elliptic filters.
Creating a Low Pass Butterworth Filter
What is a Butterworth Filter?
A Butterworth filter is known for its smooth frequency response in the passband, making it ideal for applications where minimal signal distortion is desired. It is characterized by a maximally flat frequency response and provides a gentle roll-off around the cutoff frequency.
MATLAB Code Example
To design a low pass Butterworth filter in MATLAB, you can use the `butter` function. Here’s a simple example:
% Design a Butterworth low-pass filter
fs = 1000; % Sampling frequency
fc = 50; % Cutoff frequency
[b, a] = butter(4, fc/(fs/2)); % Fourth-order Butterworth
Visualizing the Filter Response
Visualizing the frequency response of your filter is critical. It helps you understand how the filter behaves across different frequencies.
MATLAB Code Example
You can utilize the `fvtool` function to visualize your filter’s response:
fvtool(b, a); % Visualize the filter's frequency response
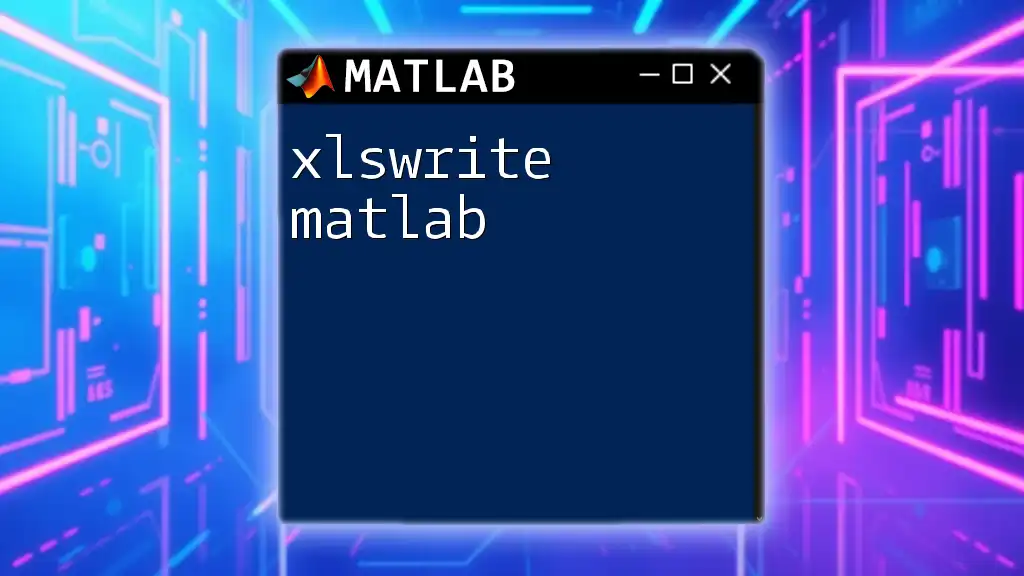
Applying the Low Frequency Filter to a Signal
Generating a Sample Signal
Before applying the filter, it's helpful to create a sample signal. For demonstration purposes, let’s generate a noisy sine wave:
t = 0:1/fs:1; % Time vector
x = sin(2*pi*10*t) + 0.5*randn(size(t)); % Noisy sine wave
Filtering the Sample Signal
To smooth the signal and reduce high-frequency noise, apply the designed Butterworth filter:
y = filter(b, a, x); % Apply the filter
Visual Comparison of Signals
It's essential to visualize both the original and filtered signals to analyze the effectiveness of your low frequency filter.
figure;
subplot(2,1,1), plot(t, x), title('Original Signal');
subplot(2,1,2), plot(t, y), title('Filtered Signal');
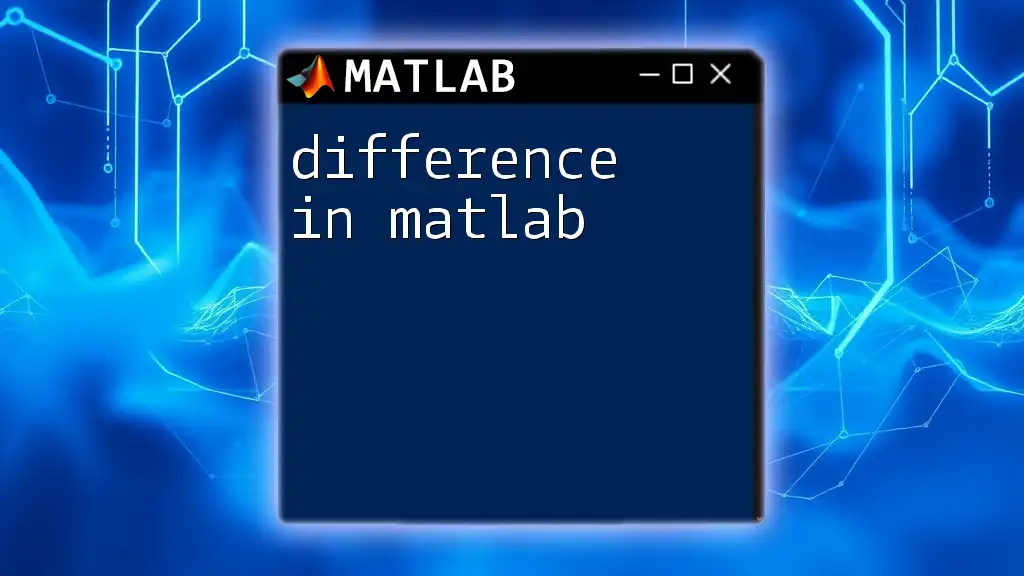
Advanced Filtering Techniques
Designing Other Types of Low Frequency Filters
While Butterworth filters are popular, other types in low frequency applications include:
-
Chebyshev Filters: These filters allow for ripple in the passband and provide a steeper roll-off, making them suitable for applications requiring sharper filtering.
-
Elliptic Filters: Combining features of both Chebyshev and Butterworth filters, elliptic filters also allow rippling but achieve rapid transition between the passband and stopband.
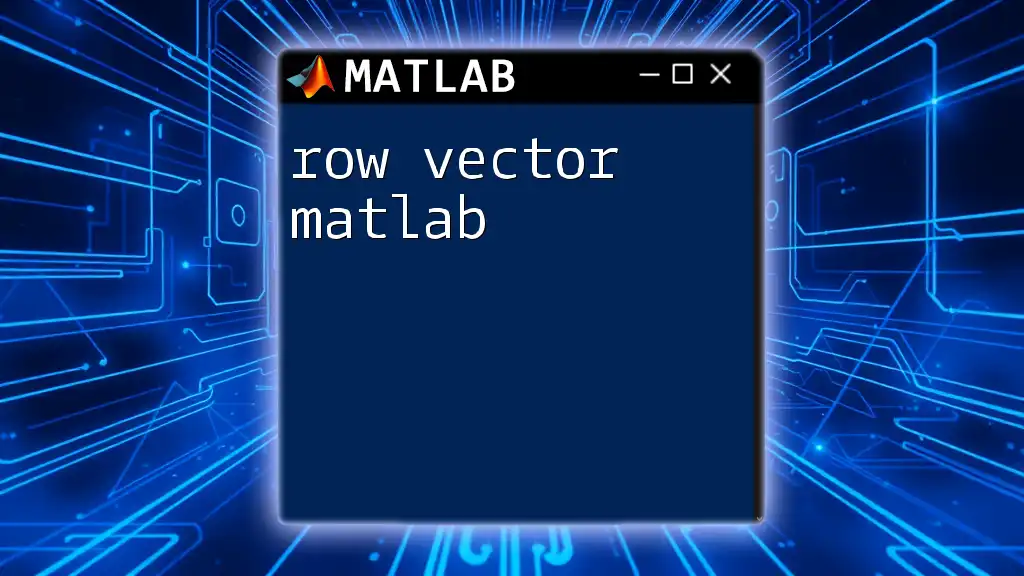
Evaluating Filter Performance
Measuring Filter Quality
Evaluating the performance of your low frequency filter involves measuring metrics such as ripple and attenuation. You'll also want to examine the stability of the filter and how it affects the phase response of signals.
Performance Evaluation Code Snippet
One way to assess the filter’s stability and performance is to visualize the frequency response:
% Assess filter stability
[h,f] = freqz(b, a, 1024, 'half');
plot(f, 20*log10(abs(h))); % Plot response in dB
title('Frequency Response of the Low Pass Filter');
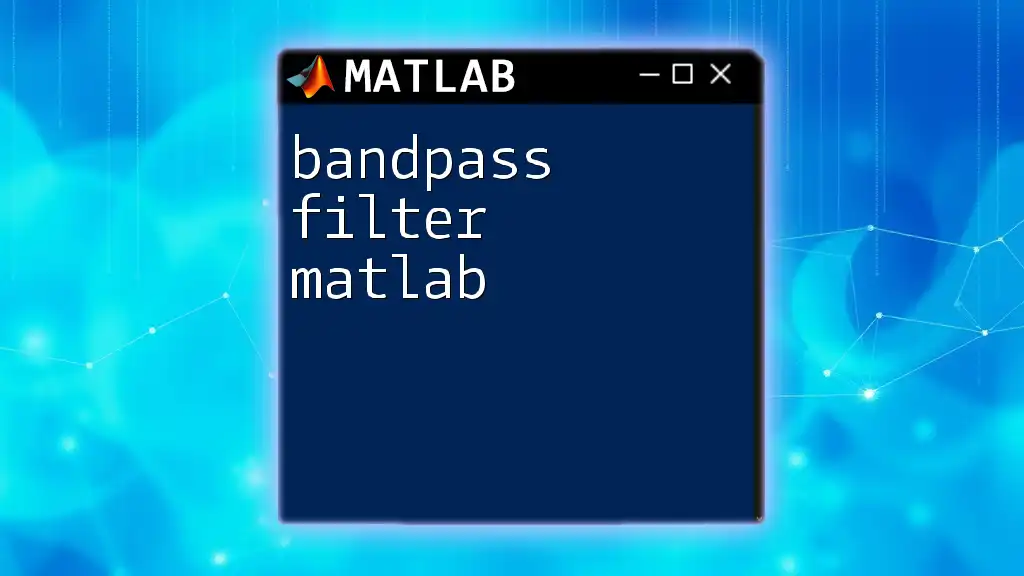
Common Pitfalls and Best Practices
Avoiding Signal Distortion
While implementing filters, one must be cautious about potential distortions in the signal due to filter characteristics. Proper design choices, such as ensuring that the sampling frequency is sufficiently higher than twice the cutoff frequency, must be considered.
Choosing the Right Filter for Your Needs
Selecting the correct filter type is crucial based on your specific application. Evaluate the requirements of your project carefully to ensure optimal performance.
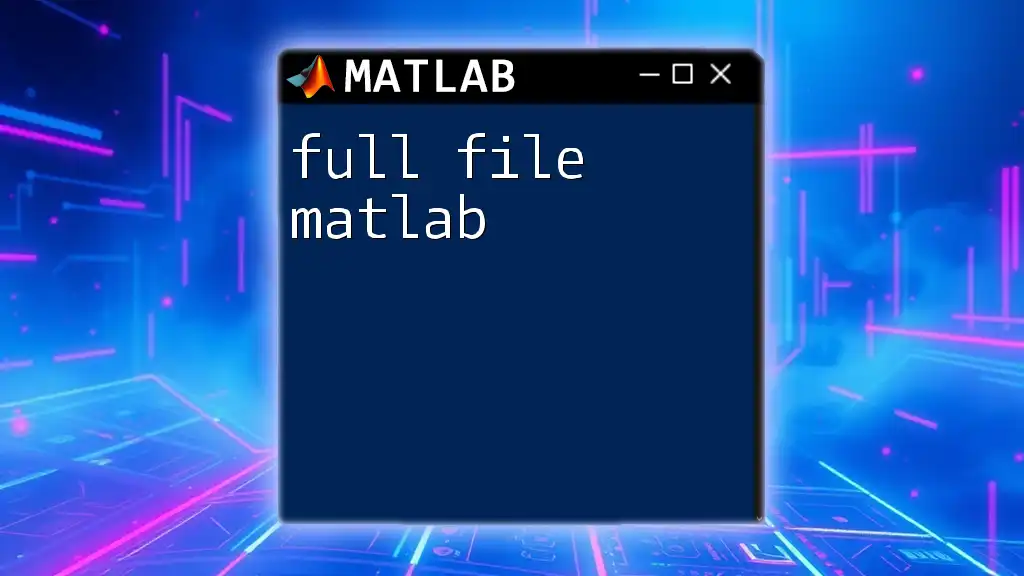
Conclusion
Engaging with low frequency filter design in MATLAB opens numerous possibilities for signal enhancement and analysis. By grasping the basic principles and implementing practical examples, you can effectively leverage low frequency filters in your projects.
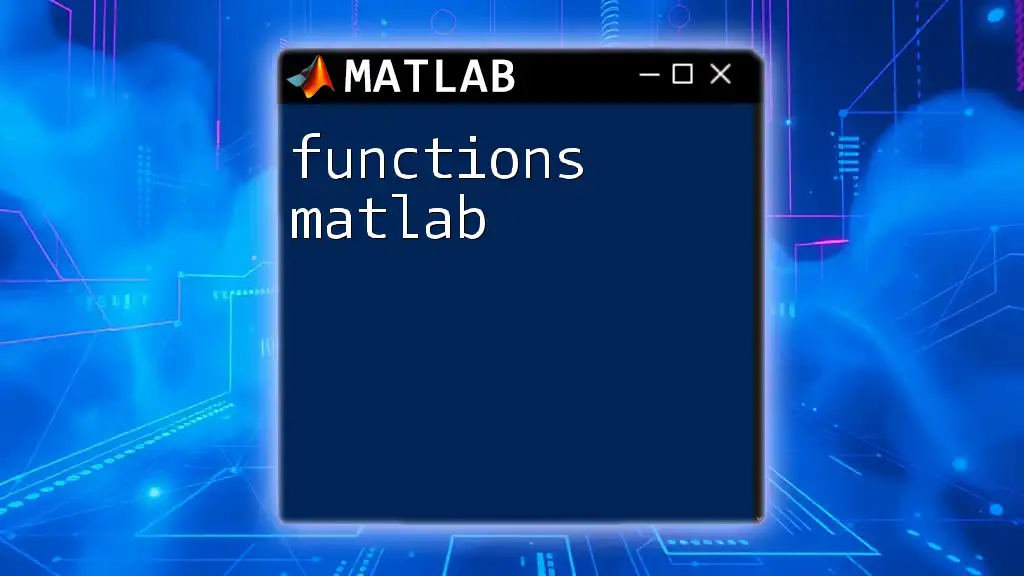
Additional Resources
For deeper exploration of filters and their design, consider visiting official MATLAB documentation and online courses focusing on signal processing techniques. These resources provide invaluable insights and practical knowledge to enhance your understanding and skills in using MATLAB for filter design.
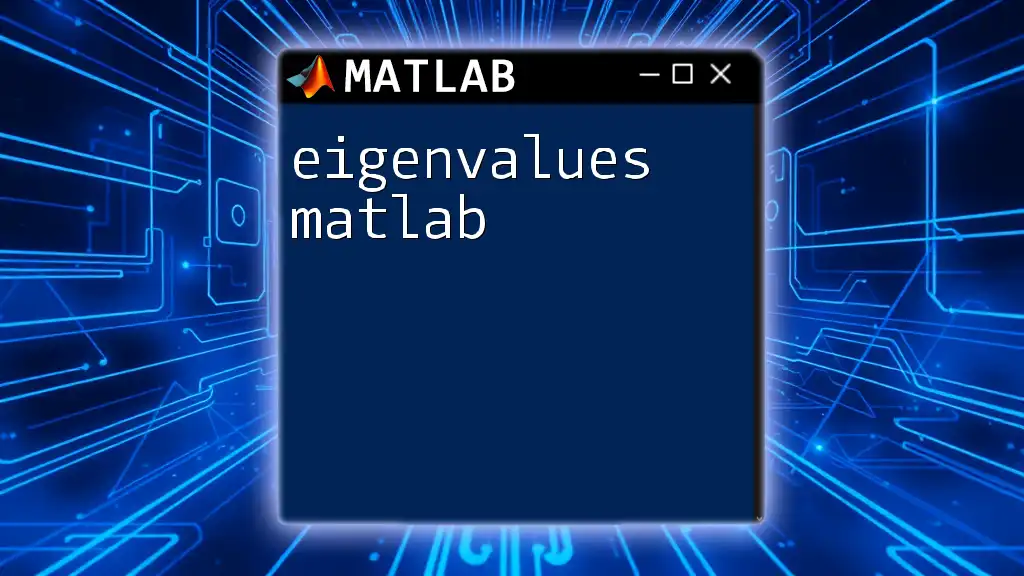
FAQs
What is the difference between a low pass filter and a high pass filter?
A low pass filter allows signals with frequencies below a specified cutoff to pass, while attenuating those above it. In contrast, a high pass filter does the opposite—it permits signals with frequencies above a cutoff to pass.
How do I set the sampling frequency for my filter?
The sampling frequency should be set according to the Nyquist theorem, which states that the sampling rate must be at least twice the highest frequency present in the signal for proper reconstruction.
Why is the order of the filter important?
The order of the filter affects its roll-off rate and performance. Higher-order filters provide sharper transitions between the passband and stopband but may introduce more complexity and potential instability.