The MATLAB command for copying a file from one location to another is `copyfile`, which allows you to specify both the source and destination paths.
copyfile('sourceFilename.txt', 'destinationFilename.txt');
What is `copyfile`?
The `copyfile` function in MATLAB is a powerful built-in command that allows users to duplicate files and folders easily. It is incredibly useful not only for basic file management but also when automating data processing tasks. In essence, `copyfile` helps you efficiently manage your files and maintain structured directories, particularly essential in data analysis and engineering workflows.
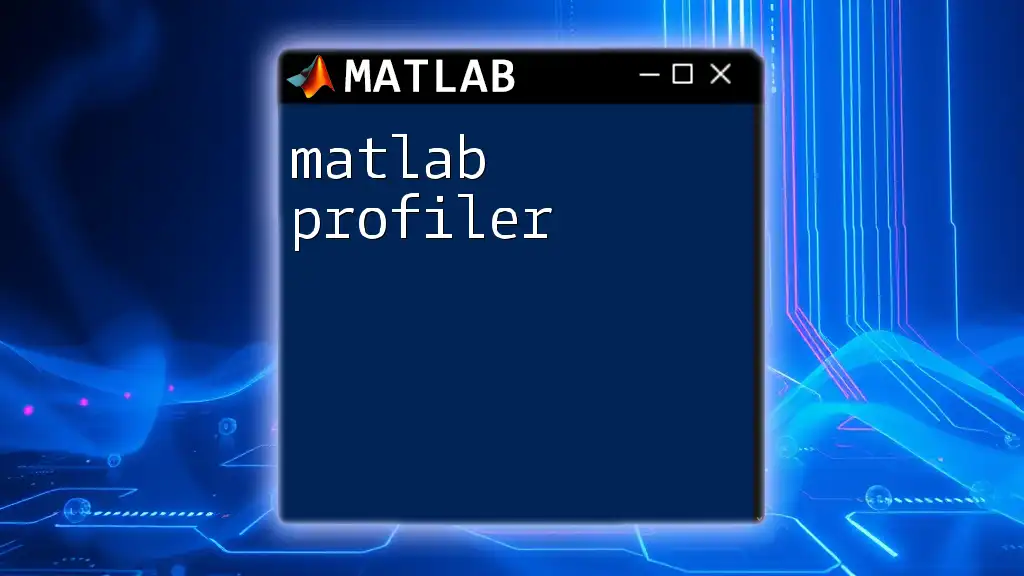
Why Use `copyfile`?
Using the `copyfile` command comes with several benefits:
- Ease of Duplication: Whether you need a single file or an entire folder, `copyfile` simplifies the copying process, removing much of the manual effort involved.
- Backup and Preservation: Ensuring that you have backups of critical files helps to prevent data loss and makes it easy to retrieve previous versions.
- Automation Potential: By integrating `copyfile` into scripts, you can automate file operations, saving time and minimizing the chance of human error.
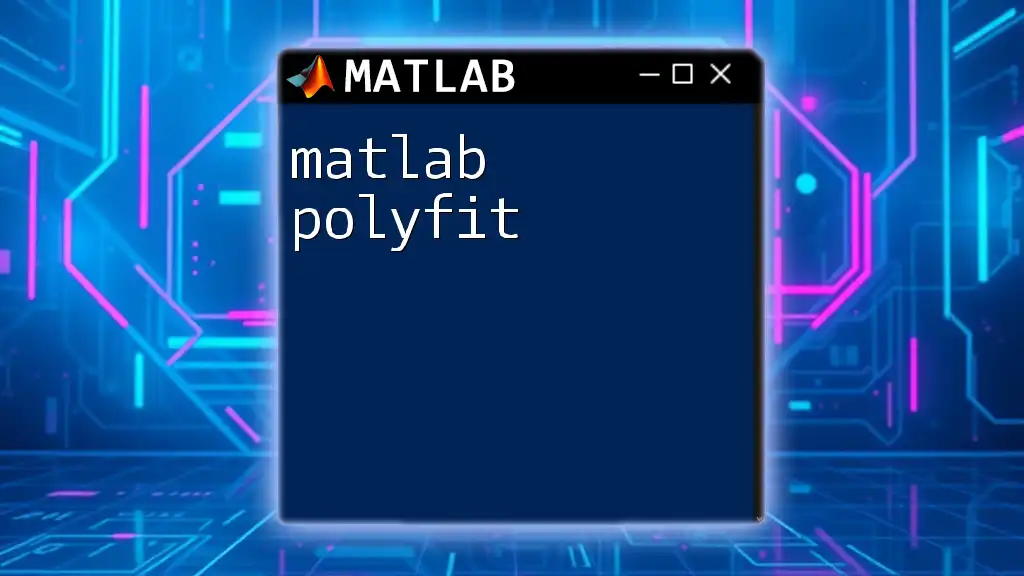
Basic Syntax of `copyfile`
Understanding the Function Syntax
The basic syntax for using the `copyfile` function is:
copyfile(Source, Destination)
- Source: Refers to the file or folder that you want to copy.
- Destination: Represents where you want the copy to be placed, which can also include a new file name.
Arguments Explained
When using the `copyfile` command, it is essential to understand the source and destination arguments:
- Source Argument: This can be specified as a full path, relative path, or a wildcard expression (like `*.txt` for all text files).
- Destination Argument: Can be a specified folder path or a new name for the file being copied.
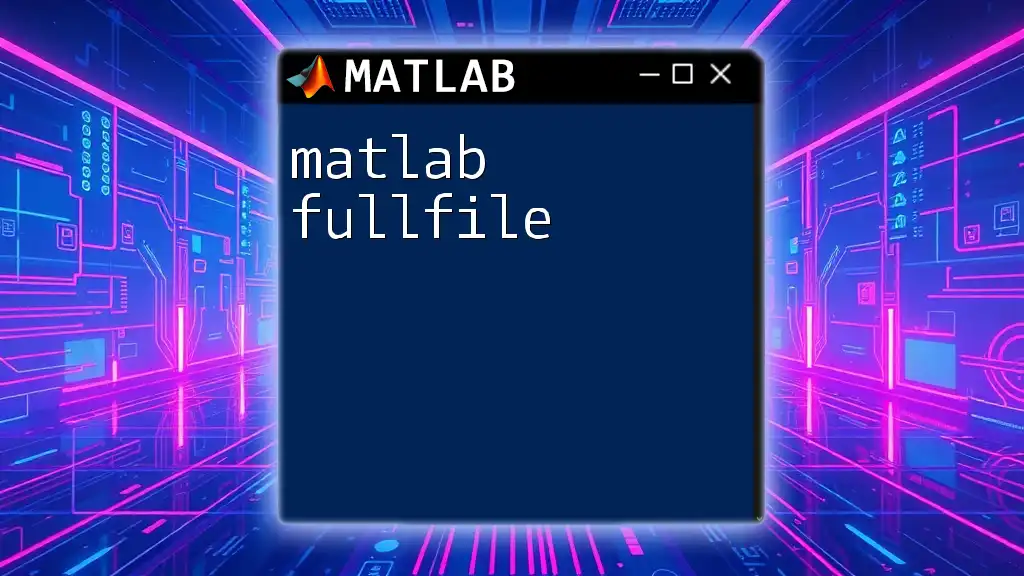
How to Use `copyfile` in MATLAB
Copying a Single File
Copying a single file is straightforward. For instance, when you want to create a backup of a file named `sourceFile.txt`, you would execute:
copyfile('sourceFile.txt', 'destinationFile.txt');
This copies `sourceFile.txt` to a new file named `destinationFile.txt` in the current directory. It’s important to ensure the source file exists; otherwise, MATLAB will throw an error.
Copying Multiple Files
You can also copy multiple files simultaneously using a wildcard. For example, if you want to copy all text files from the current directory to a backup folder:
copyfile('*.txt', 'backupFolder/');
In this command, all `.txt` files are copied to the specified folder, allowing for efficient file management without needing to name each file.
Copying Folders
If you need to copy entire directories, `copyfile` handles this as well. For instance, to copy a folder named `sourceFolder` to a new location:
copyfile('sourceFolder/', 'destinationFolder/');
This command maintains the entire directory structure and all its contents, effectively cloning `sourceFolder` into `destinationFolder`.
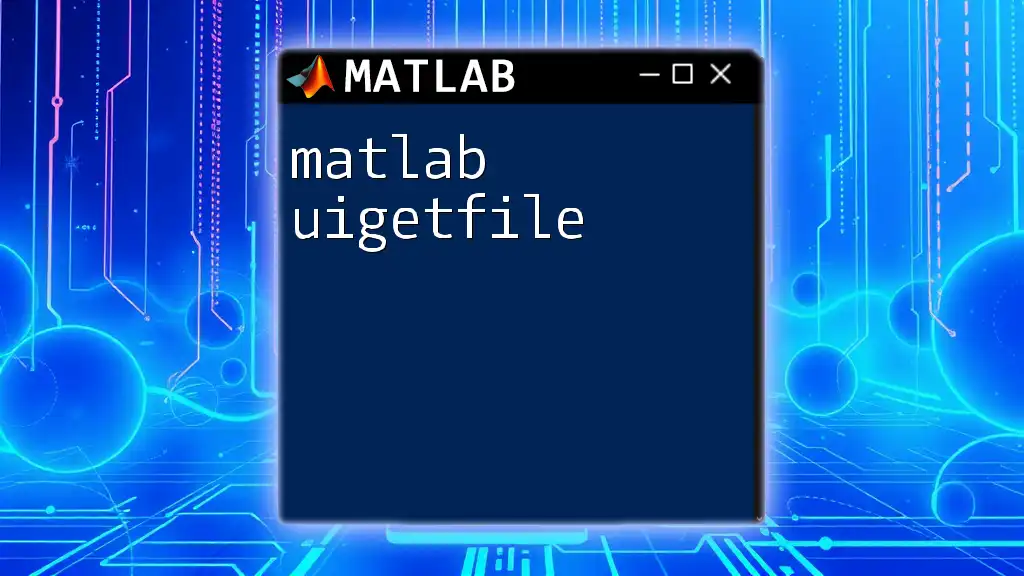
Different Modes of `copyfile`
Overwrite Existing Files
By default, if the destination file already exists, MATLAB will not overwrite it automatically. To force the overwrite of existing files, you can use the 'f' flag:
copyfile('source.txt', 'destination.txt', 'f');
Using this flag helps streamline processes where file overwriting is necessary, such as in automated scripts.
Preserve File Attributes
When copying files, you often want to keep their original attributes like timestamps and permissions. Use the 'p' flag to ensure that the file attributes are preserved:
copyfile('source.txt', 'destination.txt', 'p');
By doing this, the original file's metadata remains unchanged when copied, which is crucial for maintaining data integrity.
Verbose Output
If you want to see detailed output about what `copyfile` is doing, you can activate the verbose mode:
copyfile('source.txt', 'destination.txt', 'f', 'verbose');
This will provide feedback during the copying process, helping you track progress and quickly identify any issues that arise.
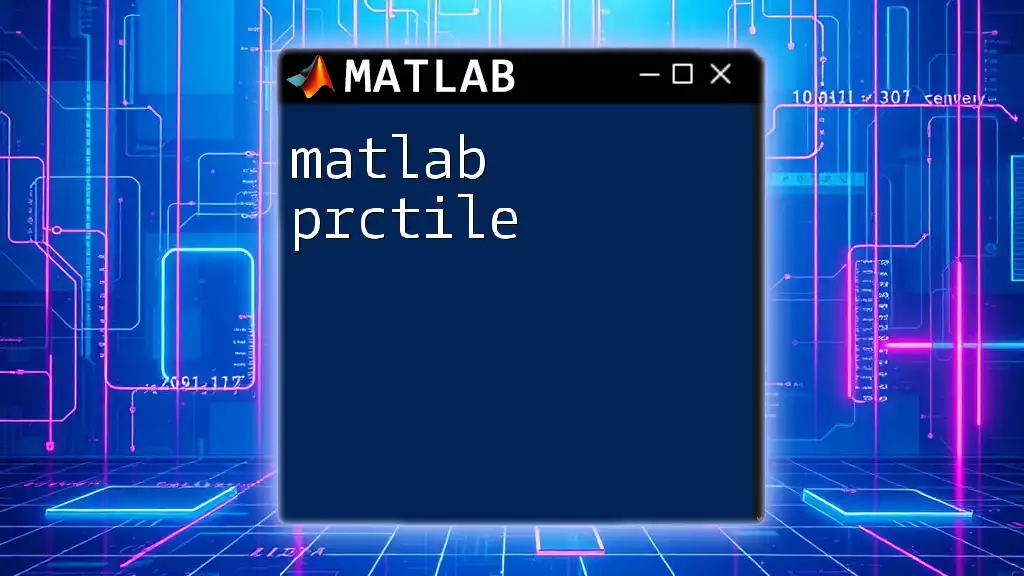
Common Errors and Troubleshooting
Error: File Not Found
A common error with `copyfile` is when the specified source file does not exist. Ensuring that the path is correct is key. Use the `exist` function to check if a file exists before proceeding with the copy:
if exist('sourceFile.txt', 'file')
copyfile('sourceFile.txt', 'backup/');
end
Error: Permission Denied
Sometimes, user permissions can prevent files from being copied. In cases where you encounter a permission denied error, check the file properties and your current directory permissions to ensure you have the necessary access rights.
Error: Destination Exists
If the target destination already contains a file with the same name, MATLAB will throw an error unless specified otherwise. You can either add a check to skip overwriting, or use the 'f' flag to ensure the existing file gets replaced.
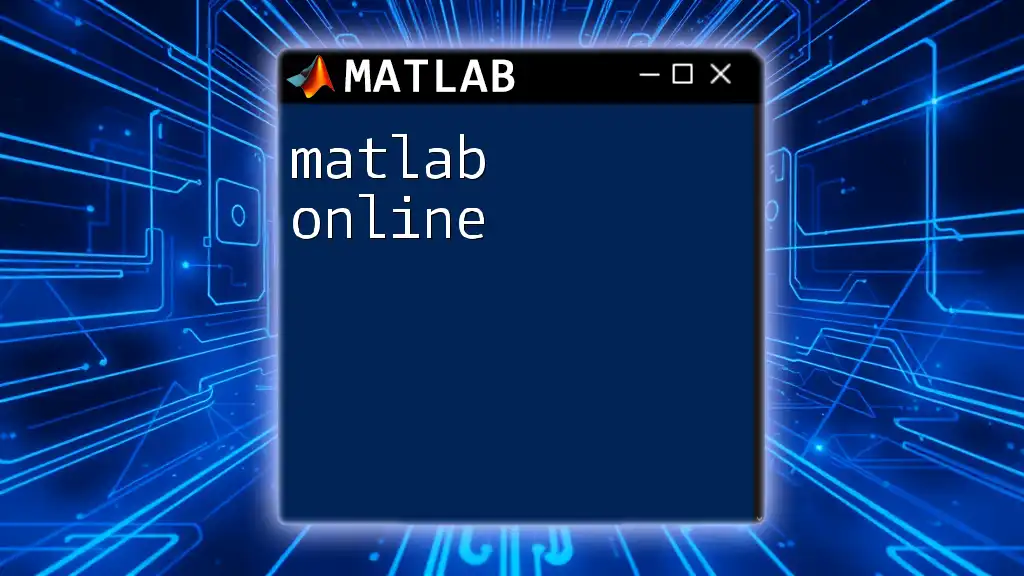
Advanced Usage of `copyfile`
Using `copyfile` in Scripts
In larger projects, integrating `copyfile` into your scripts can greatly enhance efficiency. Imagine an automated script that regularly backs up essential project files. An example snippet looks like this:
sourceFiles = {'data1.mat', 'data2.mat'};
destinationFolder = 'C:\backups\';
for i = 1:length(sourceFiles)
copyfile(sourceFiles{i}, destinationFolder);
end
This loop copies all specified files to the backup folder, automating a normally tedious task.
Copying Files Based on Conditions
Conditional copying can enhance control over your file management. For example, you could implement logic to only copy files that exist:
if exist('sourceFile.txt', 'file')
copyfile('sourceFile.txt', 'backupFolder/');
end
This conditional statement checks for the existence of `sourceFile.txt` before attempting to copy it, thus preventing runtime errors.
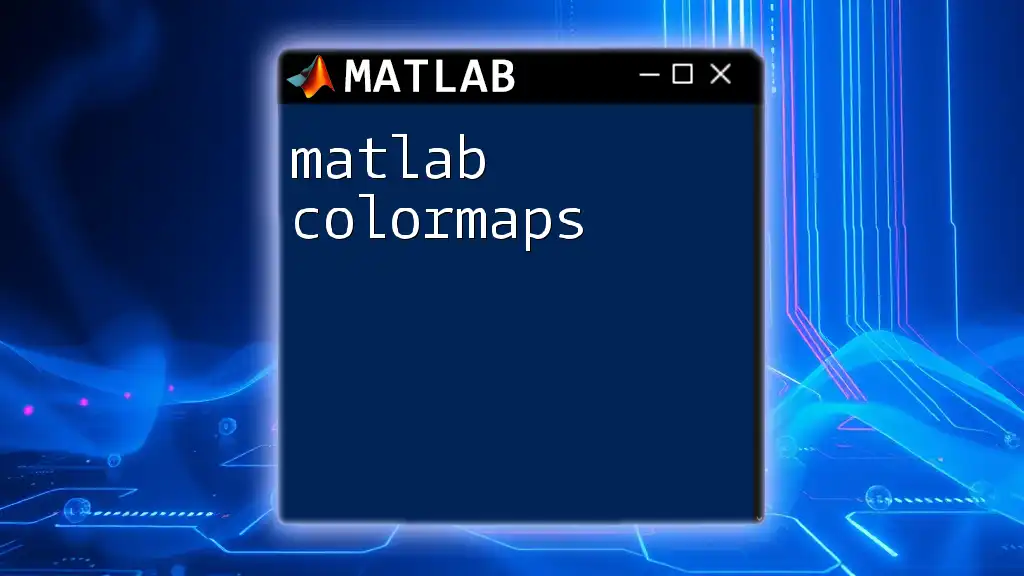
Conclusion
The `copyfile` command in MATLAB is an essential tool for any user dealing with file management. By understanding its various functionalities and syntactical options, you can enhance your workflow, safeguard your data, and automate repetitive tasks efficiently. Don’t hesitate to experiment with the command in different scenarios to learn its power fully!
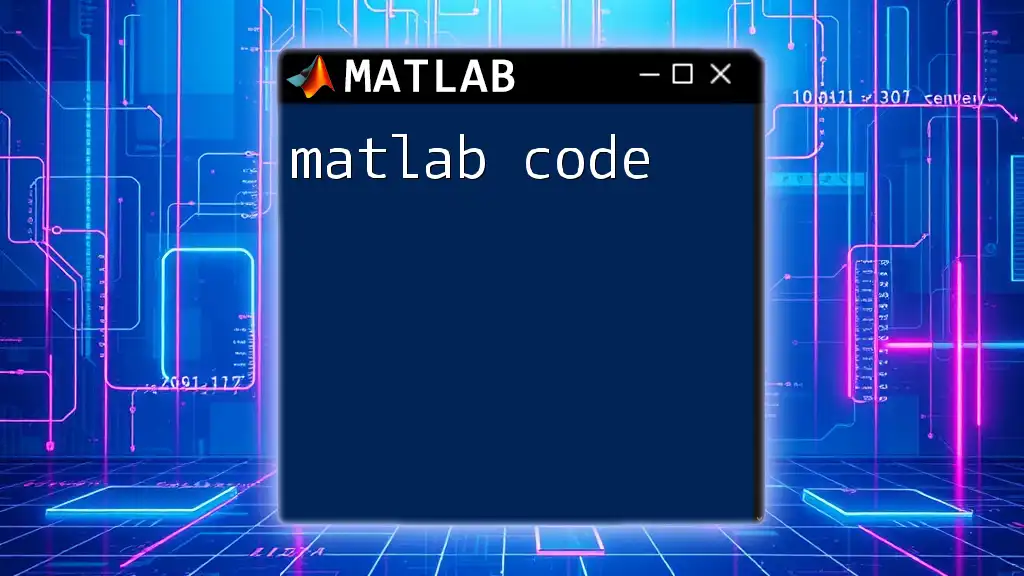
Additional Resources
For more insights and further exploration, consider diving into MATLAB's official documentation. Engaging in online forums can also provide valuable tips and community advice surrounding file handling in MATLAB, expanding your understanding and capabilities.
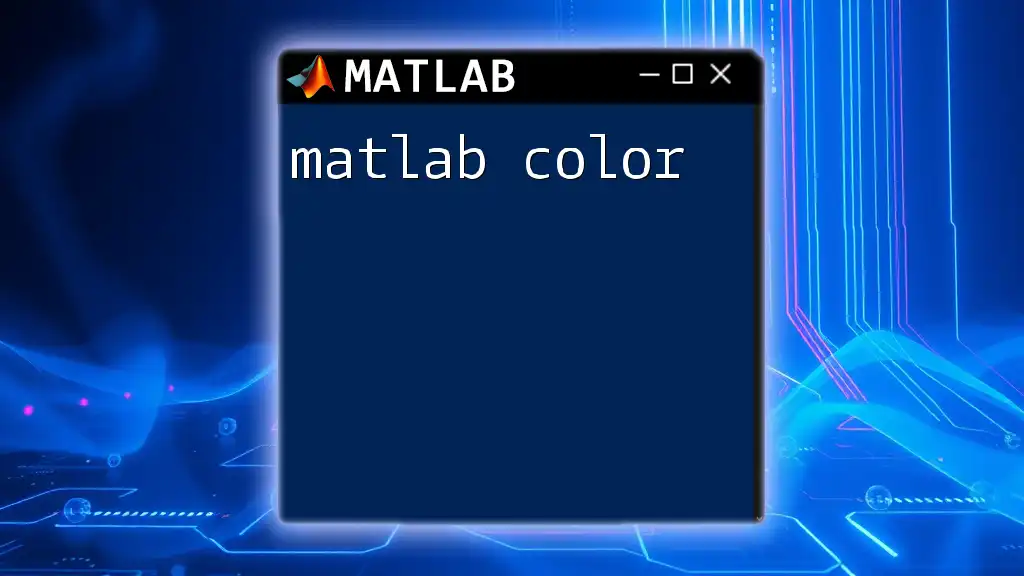
Call to Action
Stay tuned for more MATLAB tips, tricks, and tutorials by subscribing to our updates! Have questions or experiences to share about using matlab copy file? We welcome your comments and look forward to engaging with your insights.