In this post, we will demonstrate a simple convex optimization problem using the CVX toolkit in MATLAB to solve a semidefinite programming (SDP) example.
% CVX SDP Example: Solving a simple SDP problem
cvx_begin sdp
variable X(2,2) symmetric
minimize( trace(X) )
subject to
X >= eye(2) % X must be greater than or equal to the identity matrix
cvx_end
Getting Started with CVX
What is CVX?
CVX is a powerful modeling system for convex optimization problems within MATLAB. It provides a user-friendly interface to define and solve various optimization problems, especially when dealing with convex sets and functions. CVX abstracts the complex syntax often associated with traditional optimization methods, allowing users to focus on defining their problems rather than wrestling with mathematical intricacies.
Installing CVX
To utilize CVX effectively, you need to install it within your MATLAB environment. Follow these steps:
- Download CVX: Visit the official CVX website and download the appropriate version for your operating system.
- Extract Files: Unzip the downloaded folder into a convenient location on your computer.
- Add CVX to MATLAB Path: Open MATLAB and navigate to the directory where CVX is located. Run the following command to add CVX to your MATLAB path:
addpath(genpath('path_to_cvx_directory'));
Setting Up Your MATLAB Environment
To get started, create a new script in MATLAB. This script will serve as the workspace for defining the SDP problem. It’s essential to ensure that CVX is properly initialized in your script so it can recognize and execute optimization commands.
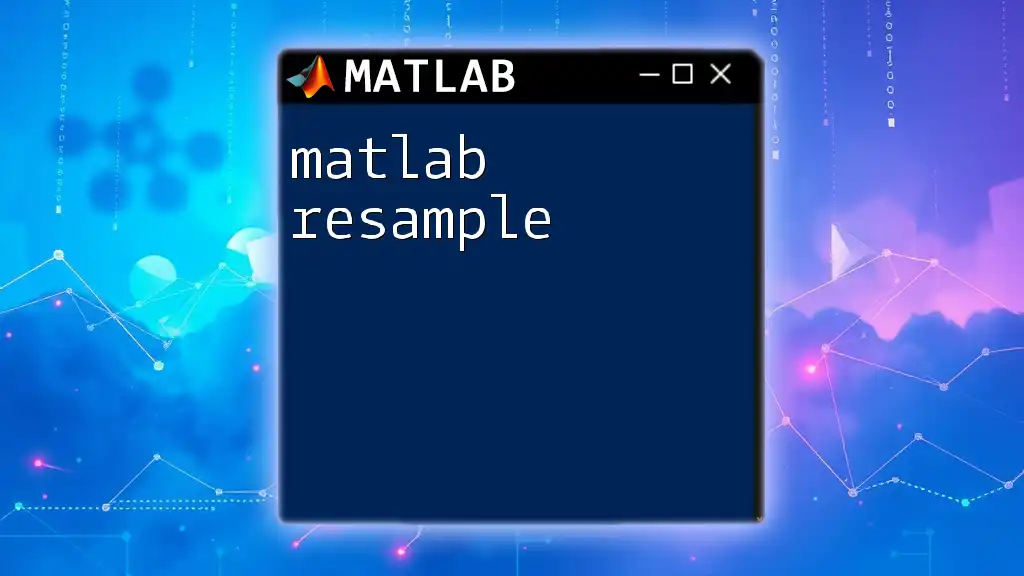
Understanding Semidefinite Programming (SDP)
Basics of SDP
Semidefinite Programming (SDP) is a subset of convex optimization problems that involves optimizing a linear objective function subject to a constraint that a matrix variable is semidefinite. SDP is crucial in various fields, including control theory, signal processing, and combinatorial optimization. Understanding its framework can unlock powerful analytical tools for solving complex real-world problems.
The Mathematical Formulation of SDP
The standard form of an SDP problem can be represented as follows:
- Objective Function: Minimize \( C : X \), where \( C \) is a symmetric matrix and \( X \) is the decision variable.
- Constraints:
- \( A_i : X \leq b_i \)
- \( X \succeq 0 \) (indicating \( X \) must be semidefinite)
In this formulation, \( : \) denotes the trace inner product, and \( \succeq \) indicates that \( X \) is greater than or equal to zero in the semidefinite order.
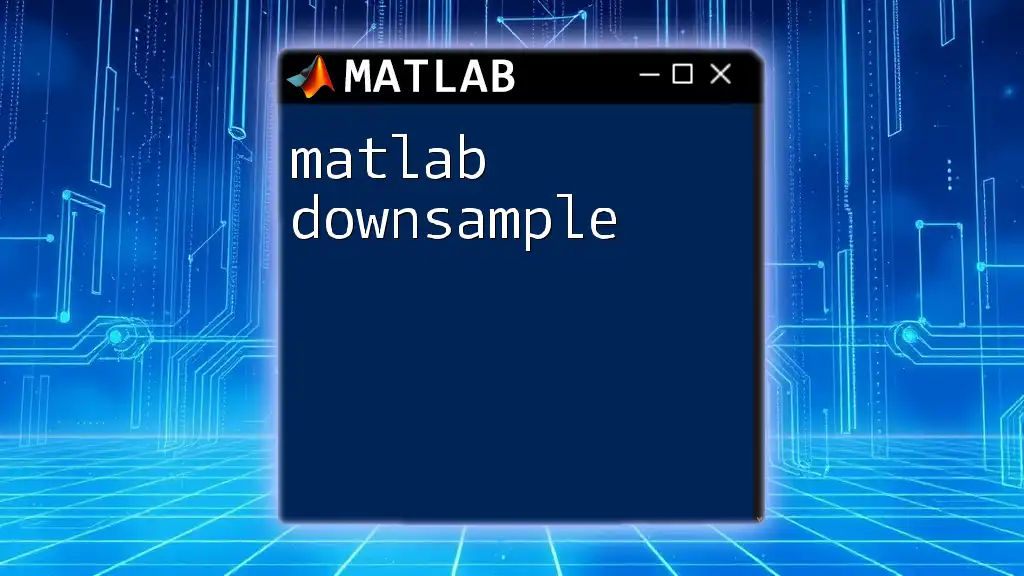
Crafting Your First CVX SDP Example
Problem Definition
Let's consider a practical SDP problem: optimizing the design of a network subject to various constraints. For instance, you want to minimize the total cost associated with assigning connections, ensuring certain criteria such as energy consumption and path reliability are met.
Setting Up the CVX Environment
First, initiate your CVX environment with:
cvx_begin sdp
% Your code here
cvx_end
This code snippet serves as the starting point for any CVX program. You encapsulate your decision variables, objective function, and constraints within the `cvx_begin` and `cvx_end` block.
Defining Decision Variables
When defining decision variables in CVX, it's important to specify their type and dimensions. For this example, let's set up a symmetric matrix \( X \):
variable X(n, n) symmetric;
Here, \( n \) is the size of the matrix, and marking it as `symmetric` ensures that CVX treats it as a semidefinite matrix throughout the optimization process.
Formulating the Objective Function
Next, define the objective function that you wish to minimize:
minimize(trace(C * X));
In this example, the trace function sums the eigenvalues of the product of matrices \( C \) and \( X \). The matrix \( C \) could represent costs or weights associated with connections in your network.
Adding Constraints
Constraints are crucial for ensuring that the solution adheres to your problem’s requirements. For instance, if you want the variable \( X \) to remain semidefinite, you can add:
X >= 0; % X must be positive semidefinite
Additionally, implement other constraints as needed:
A1 * X <= b1; % Example linear constraint
A2 * X >= b2; % Another linear constraint
Ensure each constraint appropriately encapsulates the requirements your model has to fulfill.
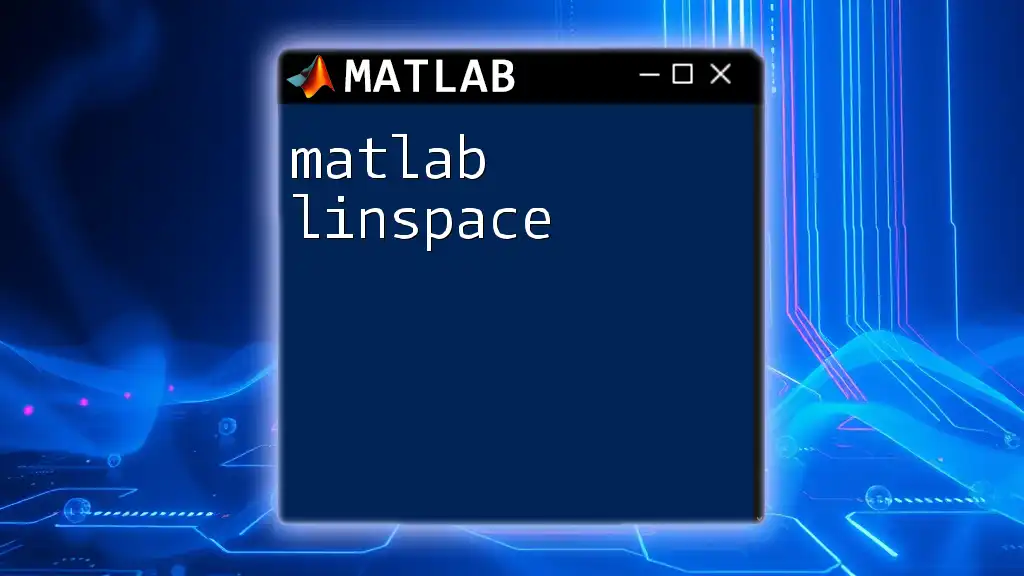
Complete CVX SDP Example
Full Code Implementation
Putting it all together, here’s a complete example of an SDP problem using CVX:
cvx_begin sdp
variable X(n, n) symmetric;
minimize(trace(C * X)); % Objective: minimize the trace
subject to
A1 * X <= b1; % Linear constraint 1
A2 * X >= b2; % Linear constraint 2
X >= 0; % Ensures X is positive semidefinite
cvx_end
Explaining the Complete Code
Each component of the code plays a vital role:
- CVX Environment: Initiates the CVX context.
- Decision Variable Definition: Declares the optimized matrix \( X \).
- Objective Function: Specifies the goal of minimizing costs based on the trace.
- Constraints: Establishes necessary limits to keep your solution feasible and practical.
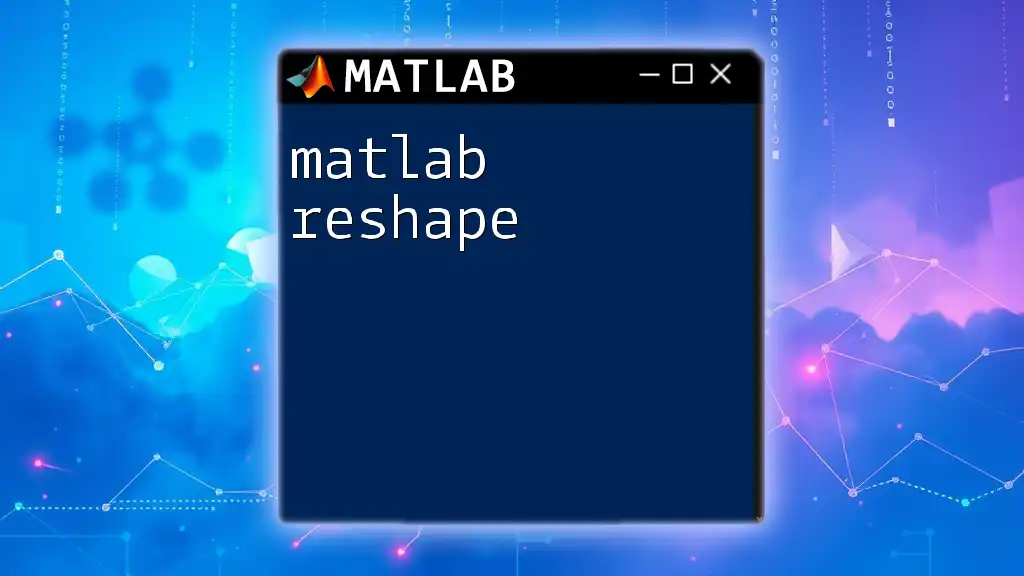
Visualizing Results
Output Interpretation
Once you've solved the SDP problem, CVX will output results that include the optimized value of your objective function and the values of the decision variables. Understand how to relate these values back to your original problem to draw meaningful conclusions from the optimization.
Visualization Techniques
Using MATLAB's built-in plotting functions, you can visualize your results to explore patterns or insights better. For example, consider plotting feasible solutions or trajectories in your network:
plot(X); % This will illustrate the optimized values
Visual aids can enhance comprehension and facilitate communication of results to stakeholders.
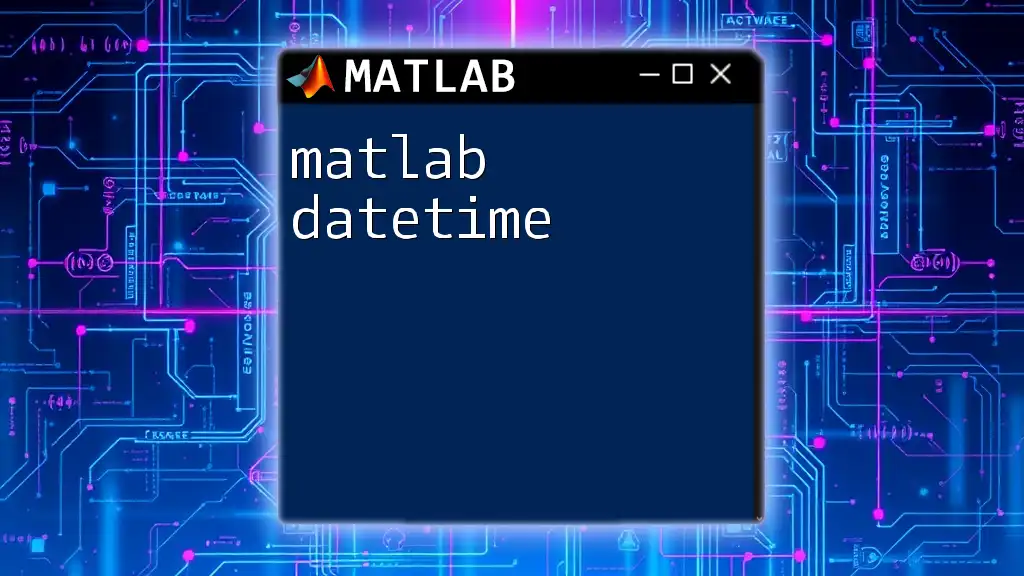
Troubleshooting Common Issues
Common Errors and Their Solutions
- Dimension Mismatch: Ensure all matrices are appropriately sized according to your constraints and objective function.
- Infeasible Problems: Revisit constraints to confirm they are achievable. Adjust as necessary based on your model’s requirements.
Best Practices for Using CVX
- Code Clarity: Structure your code to differentiate between decision variables, objectives, and constraints clearly.
- Commenting: Use comments strategically to explain complex logic or critical points in your code for future reference.
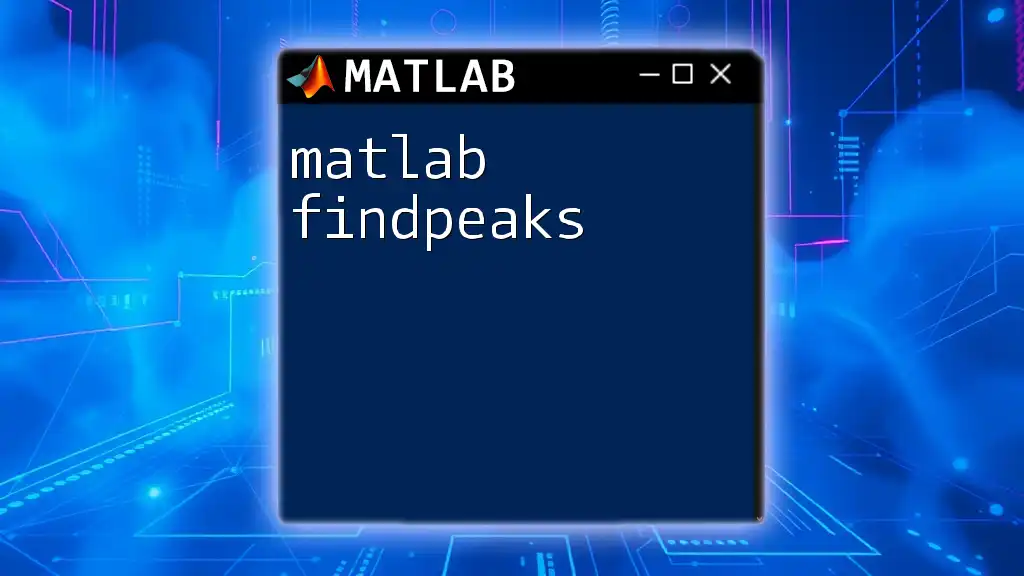
Conclusion
This guide has provided a comprehensive overview of using MATLAB CVX for semidefinite programming. By understanding the structure of your SDP problem and leveraging CVX’s capabilities, you can tackle complex optimization challenges with greater ease and efficiency. The examples outlined here serve as a foundation for further exploration of CVX's rich functionality in various applications.
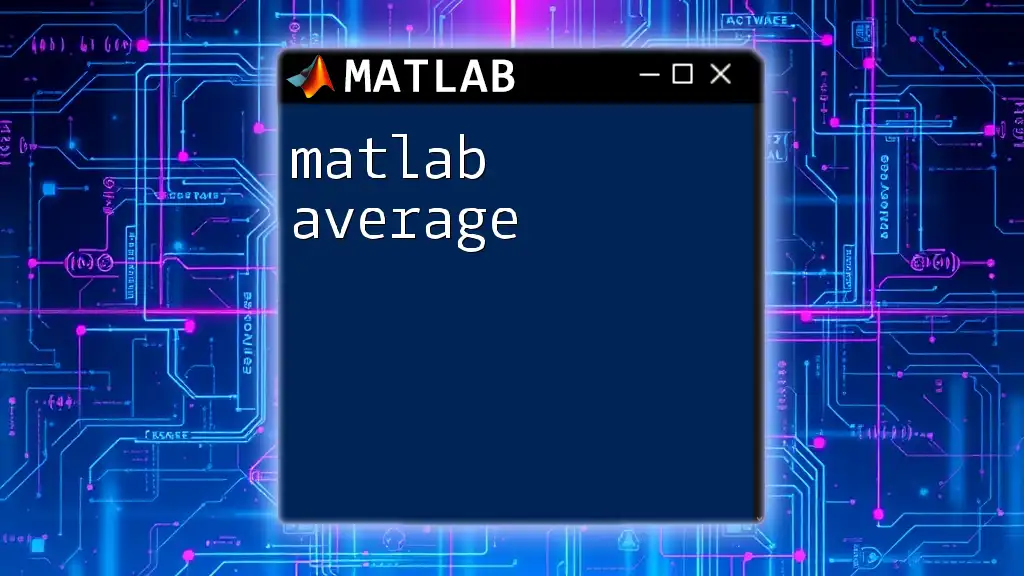
Additional Resources
- For more in-depth learning, refer to the official [CVX documentation](http://cvxr.com/cvx/).
- Consider referring to textbooks and papers on convex optimization and SDP for advanced techniques and theory.
- Engaging with online forums and communities can also provide support and insights from experienced CVX users.
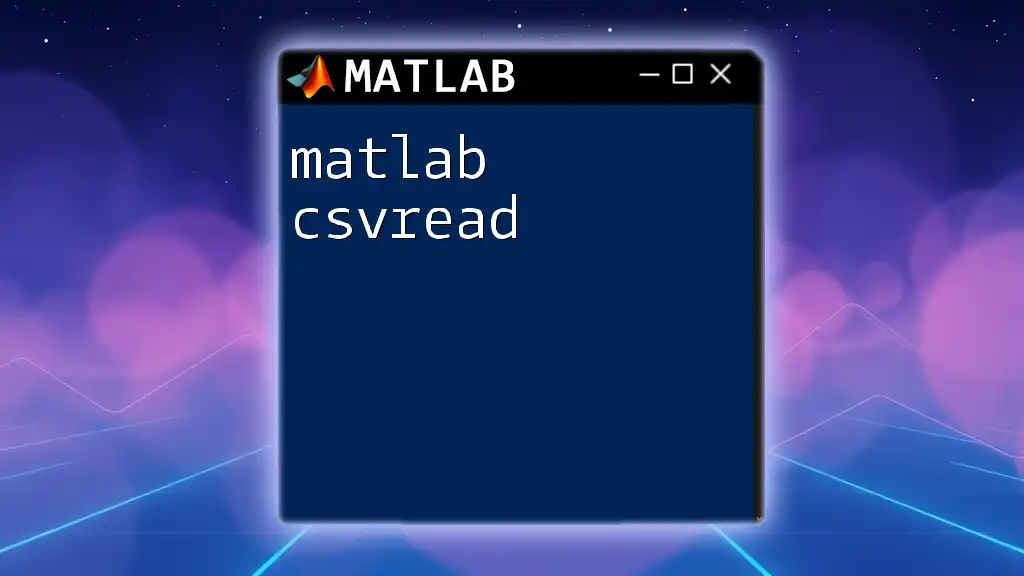
Call to Action
If you found this article helpful, consider subscribing for more concise tutorials and insights into using MATLAB effectively. Share your experiences with CVX and SDP in the comments below—your journey could inspire others in the learning process!