In MATLAB, you can adjust the size of scatter plot markers using the 'SizeData' property in the `scatter` function, allowing for effective visual representation of data points.
% Example of creating a scatter plot with custom marker sizes
x = rand(1, 10);
y = rand(1, 10);
sizes = 100 * rand(1, 10); % Random sizes for the markers
scatter(x, y, sizes, 'filled');
xlabel('X-axis Label');
ylabel('Y-axis Label');
title('MATLAB Scatter Plot with Variable Marker Sizes');
Understanding Marker Size
What is Marker Size in MATLAB?
In MATLAB, the marker size in scatter plots determines the size of the points that represent data values visually. Marker size plays a crucial role in interpreting scatter plots as it allows you to emphasize certain aspects of your data with larger or smaller markers. A larger marker often draws attention to specific data points, while smaller markers can indicate a denser collection of data.
Effect of Marker Size on Plot Interpretation
Varying marker sizes can significantly influence how a viewer interprets the data. For example, using large markers might suggest key data points that need emphasis, while smaller markers may indicate general trends without focusing on individual points.
Imagine you are plotting sales data for different products. A large marker could represent a product with exceptionally high sales, while smaller markers could indicate products with average sales. This differentiation helps viewers quickly identify areas of interest in the plot.
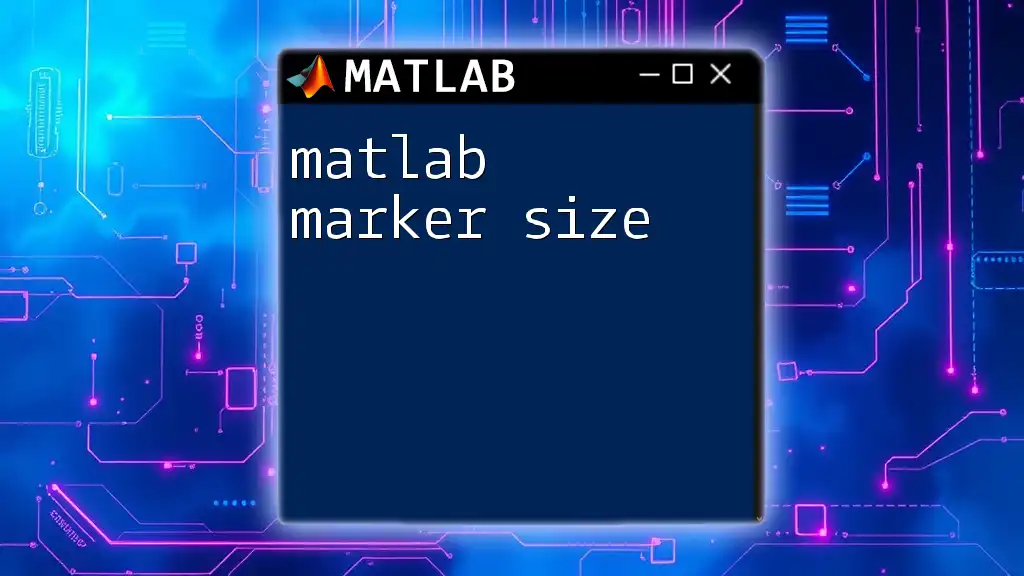
Basic Syntax for Scatter Plots
Creating a Simple Scatter Plot
To get started, consider creating a simple scatter plot. Suppose you have random x and y values:
x = rand(1, 10);
y = rand(1, 10);
scatter(x, y);
This code generates a scatter plot with the default marker size. Understanding this base plot sets the stage for exploring how marker sizes can be adjusted.
Modifying Marker Size
Changing the size of markers is straightforward. Here is an example of how to specify the marker size:
scatter(x, y, 100); % sets marker size to 100
In this snippet, the third argument (100) sets the size of the markers. You can experiment with this value to see how larger or smaller markers affect the overall appearance of the plot. A smaller number results in smaller markers, while a larger number increases the marker size.
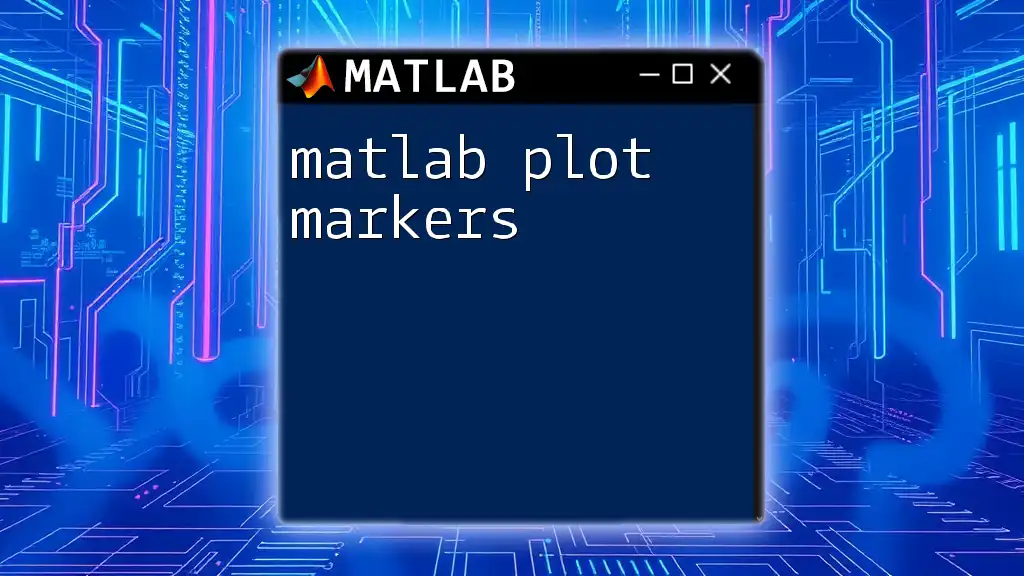
Customizing Marker Size
Using Variable Marker Sizes
One powerful feature of MATLAB scatter plots is the ability to use different sizes for markers based on another variable. This allows you to add a third dimension to your visualization. Here’s an example:
sizes = randi([10, 100], 1, 10); % random sizes
scatter(x, y, sizes);
In this code, `sizes` is an array containing random integers between 10 and 100. The scatter function uses these values to vary the sizes of the markers dynamically. This customization can visually convey information about another variable and highlight its relationship with the x and y data points.
Using a Colormap Based on Marker Size
To enhance your scatter plot further, you can use color to reflect different marker sizes. Using a colormap can add a layer of depth to your visualization:
scatter(x, y, sizes, 'filled');
colormap('jet'); % colorful representation
colorbar; % adds colorbar
By including the 'filled' option, the markers are filled with colors determined by their sizes. The `colormap` function allows you to change the color scheme used for the plot, while the `colorbar` adds a reference for interpreting the colors relative to the sizes.
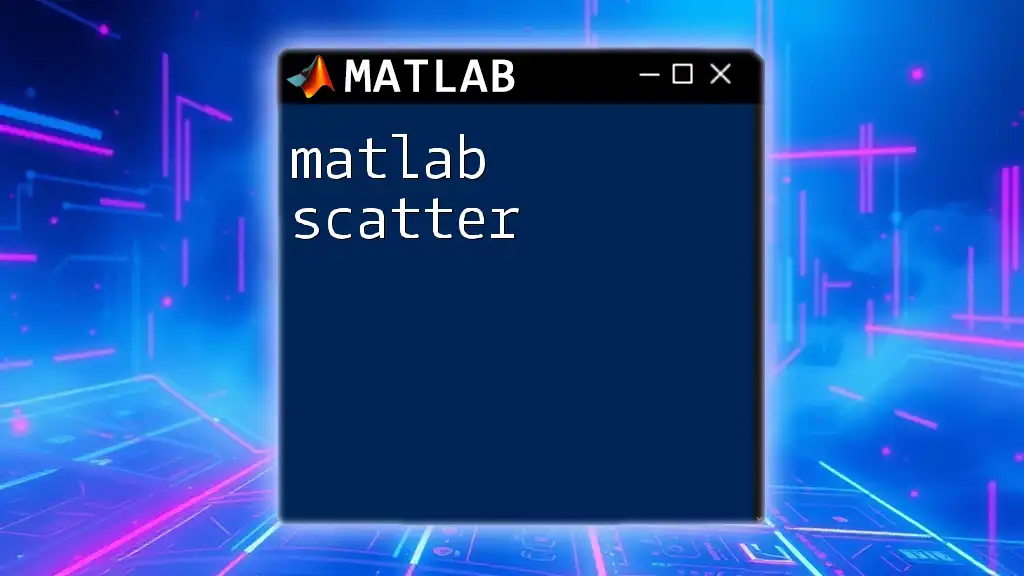
Advanced Marker Size Techniques
Dynamic Marker Sizing with Conditionals
You can make your marker sizes dynamic based on conditions in your data. This technique allows for more nuanced visual storytelling. See the example below:
sizes = ifelse(y > 0.5, 100, 50); % larger size if y>0.5
scatter(x, y, sizes);
In this snippet, if the y-value exceeds 0.5, the marker will be larger than the default size. This method can effectively emphasize outliers or significant values in your data.
Interactive Plots with Marker Sizing
MATLAB also supports interactive scatter plots using functions like `gscatter`, which allows for grouping by another variable:
group = [1, 1, 2, 2, 1, 1, 2, 2, 1, 2];
scatter(x, y, sizes, group);
Here, `group` defines the categories for your data points. The ability to group data visually enhances analysis, as observers can see how different groups compare regarding size and position in the plot.
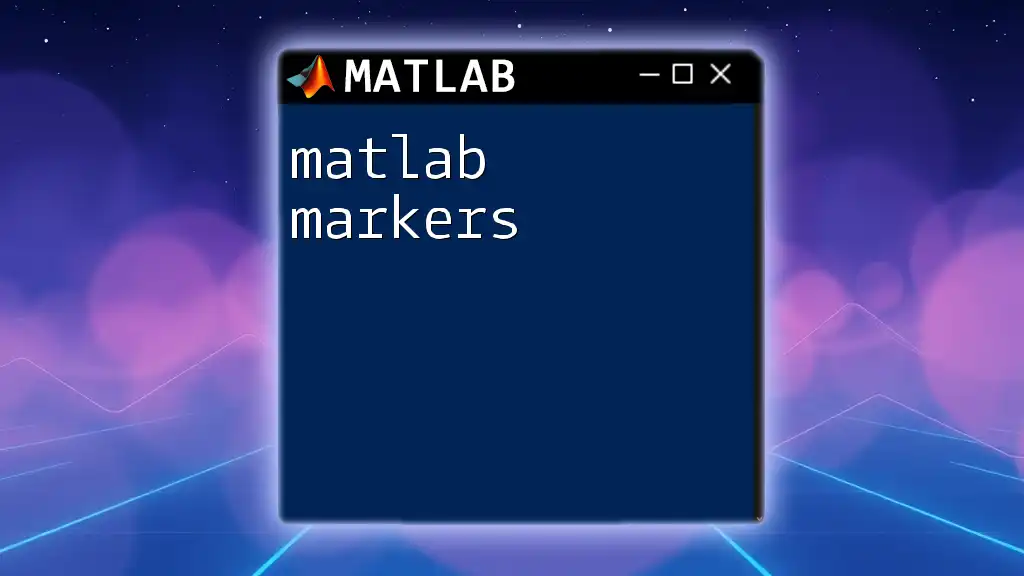
Common Pitfalls and Best Practices
Selecting Appropriate Marker Sizes
When choosing marker sizes, be cautious. Too small markers may become indistinguishable, particularly in dense plots, while too large markers may obscure other data points. Balancing visibility and clarity is key.
Clear Visualizations: Accessibility Considerations
For inclusive data visualizations, consider the appearance of your markers on different backgrounds. Ensure sufficient color contrast for viewers with visual impairments. Utilizing color palettes that are accessible to colorblind users can broaden the reach and effectiveness of your scatter plots.
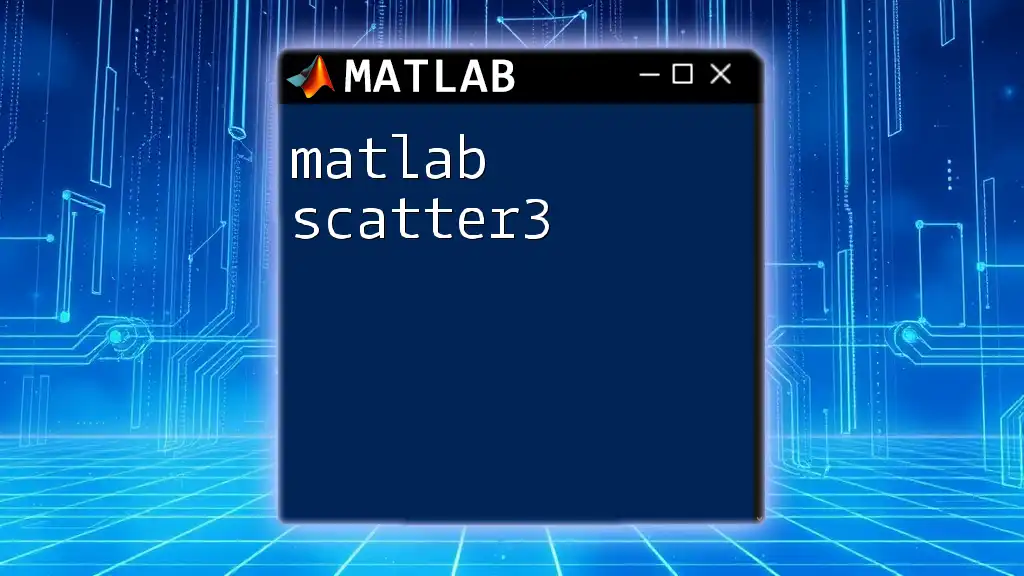
Conclusion
In conclusion, understanding and mastering the MATLAB scatter marker size can transform how you present data visually. The ability to manipulate marker sizes not only enhances informative value but also engages the audience more deeply. Don’t hesitate to experiment with different sizes and color schemes in your projects to maximize clarity and sense.
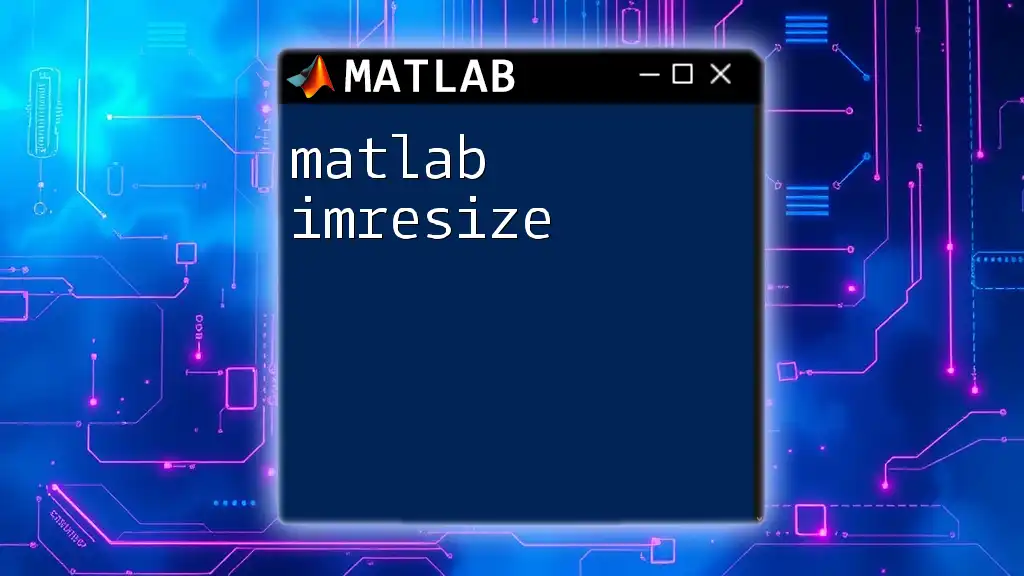
Additional Resources
To enrich your learning, refer to MATLAB’s official documentation for scatter plots, which provides in-depth information and further examples. Additionally, consider exploring online courses or tutorials that focus on data visualization in MATLAB, where you can learn structured techniques.
FAQs
What is the maximum marker size in MATLAB?
The maximum marker size can be quite large, but practical limits depend on the size of your figure and how the markers appear relative to other data points.
Can marker size be used to represent categorical data?
Yes, you can assign different sizes to markers based on categorical data, allowing for effective differentiation within your scatter plots.
How to reset marker size to default?
Simply call the `scatter` function without an explicit size argument to revert to the default marker size.