The Student's t-test in MATLAB is a statistical test used to determine if there is a significant difference between the means of two groups, and it can be performed using the `ttest2` function.
Here’s a code snippet demonstrating how to perform a two-sample t-test:
% Sample data for two groups
group1 = [23, 21, 25, 30, 28];
group2 = [30, 31, 29, 35, 32];
% Perform the two-sample t-test
[h, p] = ttest2(group1, group2);
% Display the results
fprintf('Hypothesis test result (h): %d\n', h);
fprintf('p-value: %f\n', p);
Understanding the Student T-Test
What is the Student T-Test?
The Student T-Test is a statistical method used to determine if there is a significant difference between the means of two groups. It is particularly useful when comparing sample data to a population mean or when assessing differences between two independent or related groups.
There are different types of t-tests:
- One-sample t-test: Compares the mean of a single group to a known value (e.g., a population mean).
- Independent two-sample t-test: Compares the means from two different groups to see if they are significantly different from each other.
- Paired sample t-test: Used when the samples are related or matched, such as measurements taken from the same subjects before and after a treatment.
When to Use a Student T-Test
A t-test is applicable under specific conditions:
- Your data should be approximately normally distributed, especially important when the sample size is small (n < 30).
- The sample sizes should be small (less than 30) or equal, although it can be used for larger sample sizes too.
- The variances of the two groups should be equal if you’re performing an independent t-test. If not, an unequal variance adjustment can be made.
In contrast to other statistical tests like ANOVA, the Student T-Test is particularly advantageous when comparing two groups, providing straightforward interpretation of results.
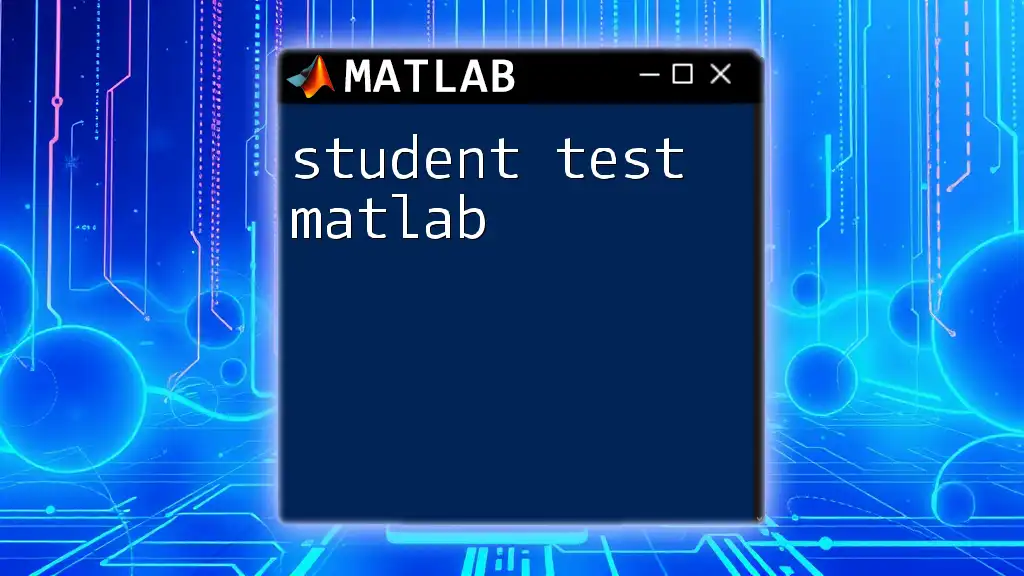
Setting Up MATLAB for T-Test Analysis
Installing MATLAB
To perform a Student T Test in MATLAB, you’ll first need to have MATLAB installed. You can download it from the MathWorks website, ensuring you also have the Statistics and Machine Learning Toolbox, which contains the functions necessary for conducting t-tests.
Essential MATLAB Functions for T-Tests
MATLAB provides several built-in functions that facilitate conducting t-tests:
- `ttest`: For one-sample t-tests.
- `ttest2`: For independent two-sample t-tests.
- `ttest3`: For paired t-tests.
- `mvt`: For multivariate t-tests.
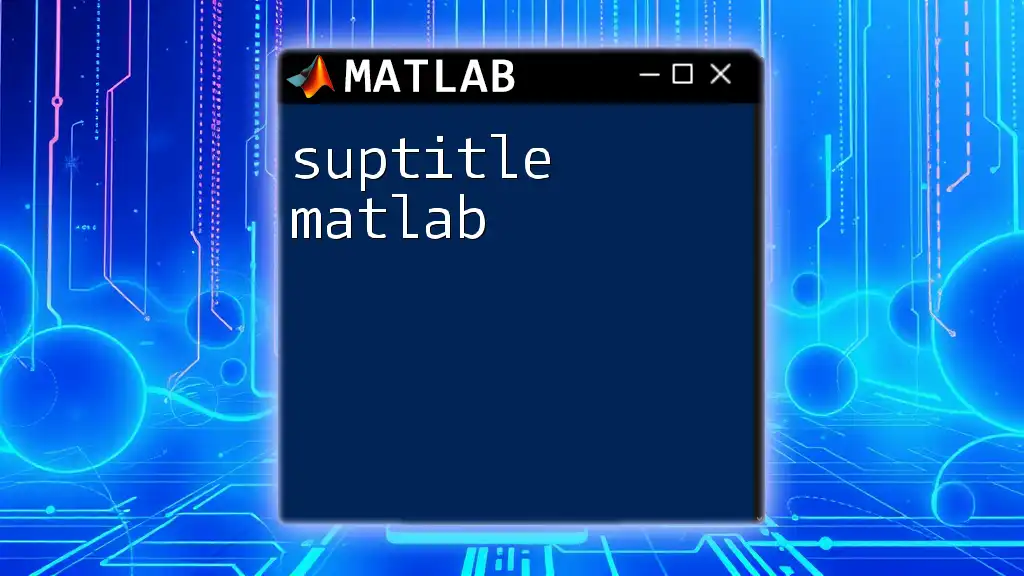
Conducting a One-Sample T-Test in MATLAB
Step-by-Step Guide
To conduct a one-sample t-test, you can start with the following code snippet:
% Sample data
data = [2.3, 2.5, 2.8, 3.1, 3.0];
% Hypothesized mean
mu = 2.8;
% Perform the t-test
[h, p] = ttest(data, mu);
Explanation of Code
- data: This variable contains the sample data you are testing.
- mu: This is the hypothesized mean you are testing against (e.g., a population mean).
- ttest function returns two values:
- h: This is a binary result (1 or 0) indicating whether to reject the null hypothesis (h=1 means reject).
- p: The p-value indicates the probability of observing the data given that the null hypothesis is true.
Example Scenario
Imagine you want to test whether the average test score of your class is significantly different from a known passing score of 2.5. By using a one-sample t-test, you can statistically validate whether your class's average exceeds this threshold.
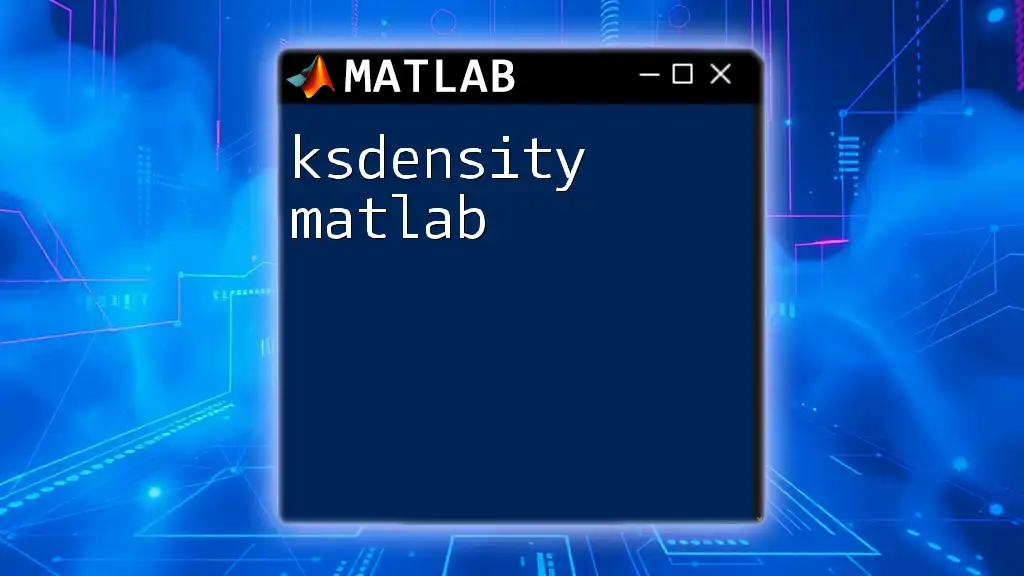
Conducting an Independent Two-Sample T-Test in MATLAB
Step-by-Step Guide
To perform an independent two-sample t-test, you can use the following code:
% Sample data from two independent groups
group1 = [2.3, 2.5, 2.8, 3.1];
group2 = [3.0, 3.2, 3.5, 3.7];
% Perform the t-test
[h, p] = ttest2(group1, group2);
Explanation of Code
Similar to the one-sample test, `ttest2` compares the means of two independent groups:
- group1 and group2 contain the sample data from two different groups you want to compare.
Example Scenario
Imagine you’re comparing the effectiveness of two different teaching methodologies by analyzing test scores from students taught with each method. An independent two-sample t-test allows you to determine if the average scores are statistically different.
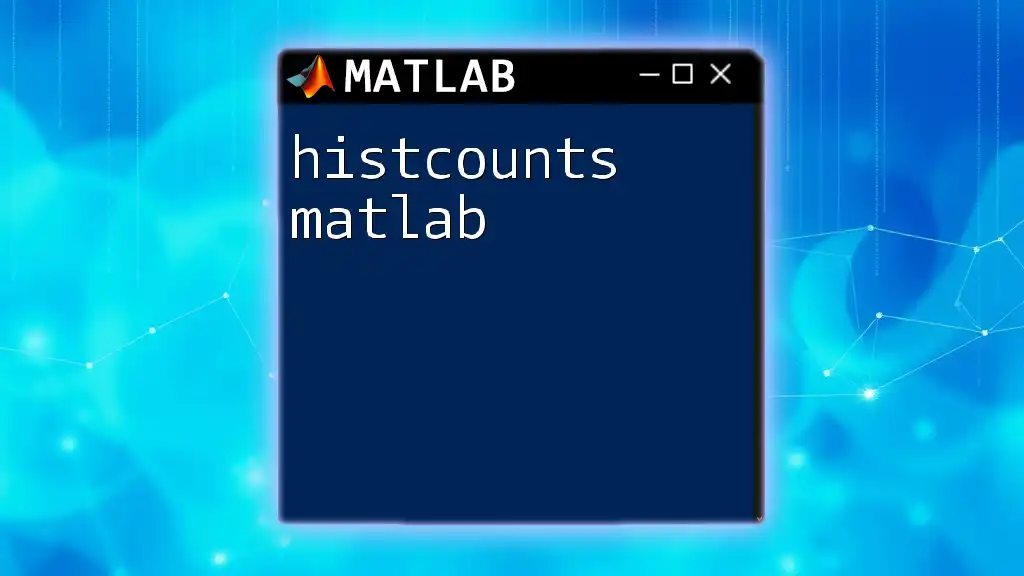
Conducting a Paired Sample T-Test in MATLAB
Step-by-Step Guide
Here’s how to conduct a paired sample t-test:
% Sample data from the same subjects
before = [2.5, 3.0, 2.8, 3.4];
after = [3.5, 3.0, 3.2, 4.0];
% Perform the paired t-test
[h, p] = ttest(before, after);
Explanation of Code
The code above employs the `ttest` function for paired data:
- before and after contain measurements from the same group before and after an intervention.
Example Scenario
Consider a clinical trial where patients' blood pressure is recorded before and after administering a new drug. A paired t-test helps assess whether the new treatment is effective compared to the baseline readings.
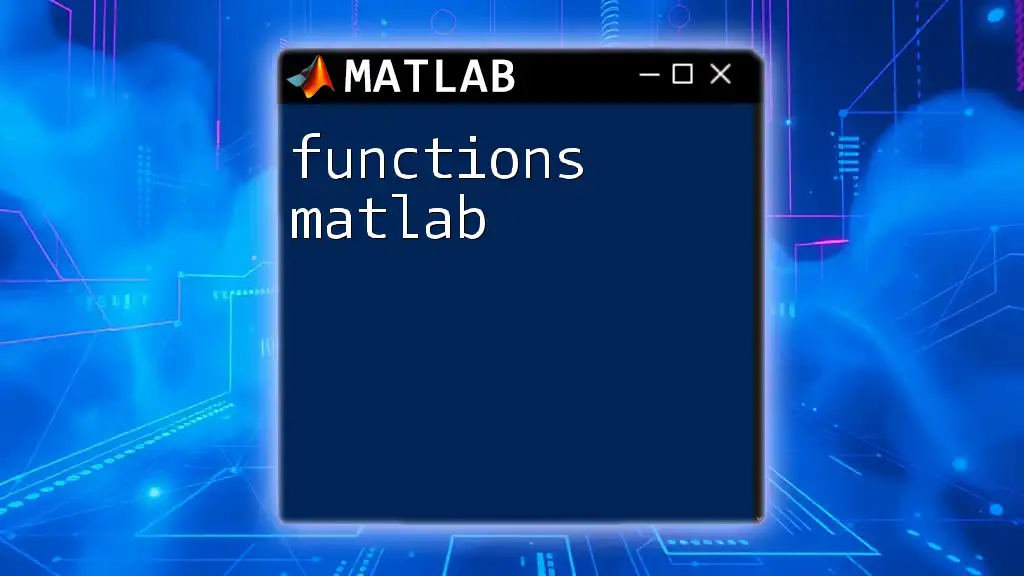
Interpreting T-Test Results
Understanding p-Values
The p-value obtained from the t-test is essential in hypothesis testing. A small p-value (typically ≤ 0.05) indicates strong evidence against the null hypothesis, leading to its rejection. Conversely, a larger p-value suggests that there is insufficient evidence to reject the null hypothesis.
Common Misinterpretations
The interpretation of p-values can often be misleading. It’s crucial to remember:
- The p-value does not indicate the size of the observed effect.
- It does not prove or disprove a hypothesis but merely suggests whether the data observed is consistent with the null hypothesis.
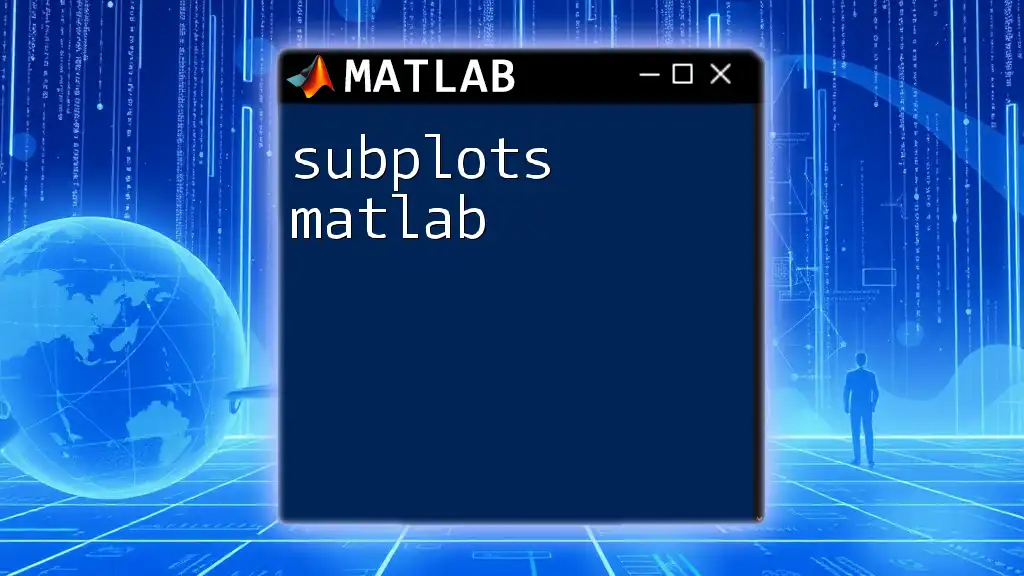
Practical Tips for Using T-Tests in MATLAB
To effectively use t-tests in MATLAB:
- Ensure Data Preparation: Clean and preprocess data adequately to meet t-test assumptions.
- Visualization: Use plots (box plots or histograms) to visualize the distribution of data, which helps in verifying normality assumptions.
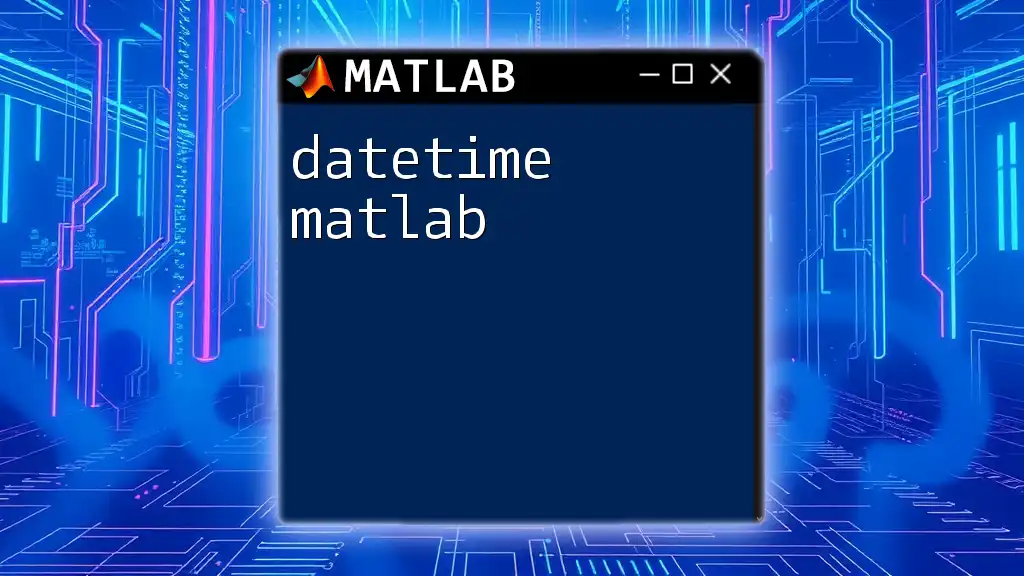
Conclusion
The Student T-Test in MATLAB is a powerful tool for statistical analysis, permitting researchers and data analysts to quickly assess differences between means across various scenarios. By following the provided guidelines and utilizing code snippets effectively, you can apply this statistical test in your datasets with confidence.
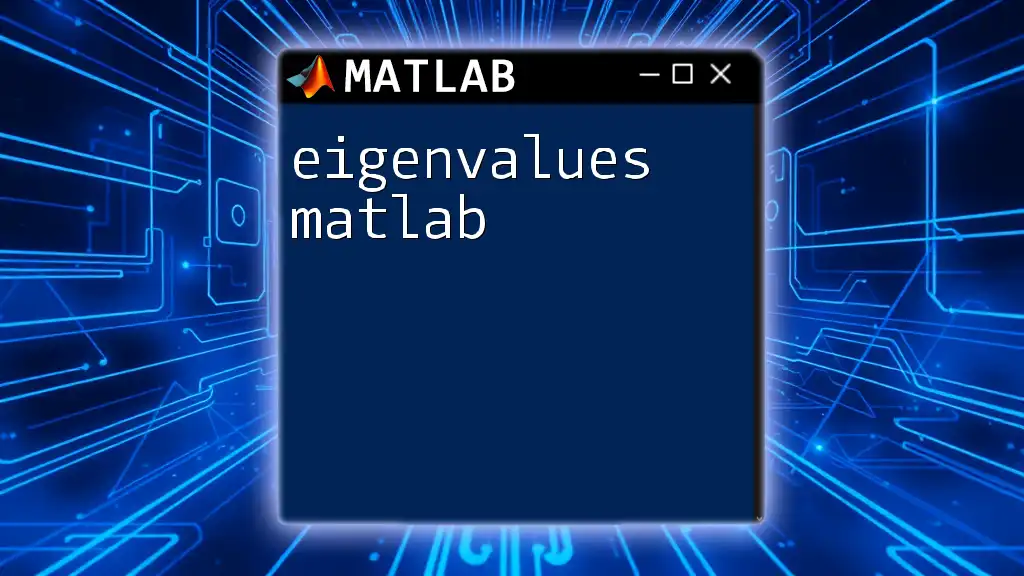
Additional Resources
To deepen your understanding, consider exploring further readings on statistical analysis and reviewing MATLAB's official documentation. This will enhance your overall capability in statistical programming and analysis with MATLAB.