The Fast Fourier Transform (FFT) in MATLAB is a powerful function for analyzing the frequency components of signals, and it can be implemented succinctly with the following code snippet:
Y = fft(x); % Computes the FFT of the input signal x
What is FFT?
FFT, or Fast Fourier Transform, is a highly efficient algorithm that computes the discrete Fourier transform (DFT) and its inverse. Its significance lies in transforming data between time (or spatial) domain and frequency domain, making it a cornerstone in fields like signal processing, image analysis, and telecommunications.
Applications of FFT
The applications of FFT are vast, including:
- Audio Processing: Analyzing sound frequencies in music files or environmental sounds.
- Image Processing: Enhancing features in images or performing noise reduction.
- Signal Analysis: Understanding and interpreting signals in data-heavy environments like telecommunications.
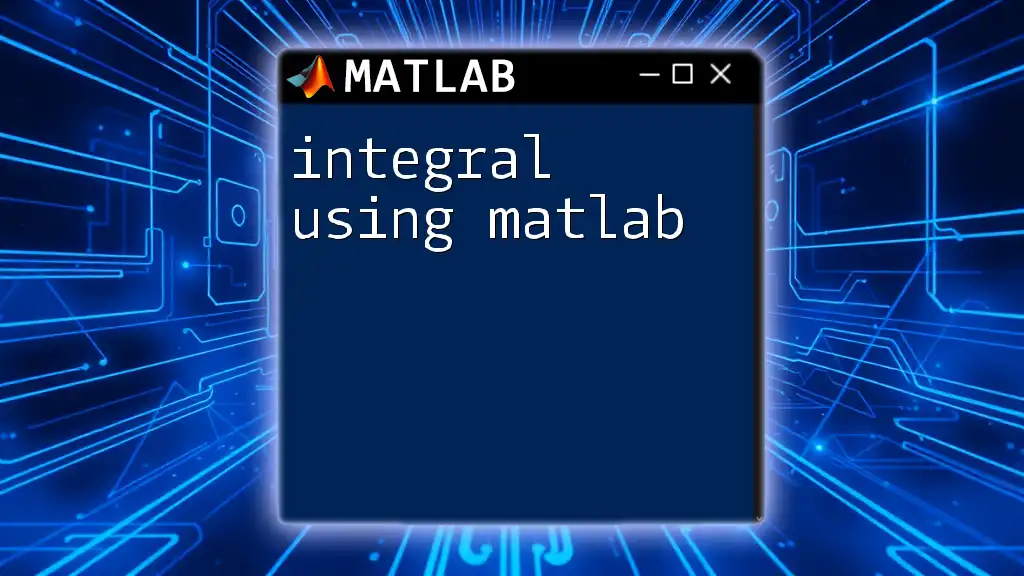
Understanding the Basics of FFT
Fourier Transform Fundamentals
At its core, the Fourier Transform (FT) decomposes a function into its constituent frequencies. It provides insights into how much of each frequency exists in the original signal or image. The distinction between continuous and discrete Fourier Transform is critical, as FFT typically deals with discrete data sets frequently encountered in computer applications.
How FFT Works
FFT simplifies the process of computing DFT, reducing computational complexity from O(N^2) to O(N log N). This efficiency makes FFT the preferred choice for processing large datasets, as it handles big data seamlessly compared to the naive DFT algorithms.
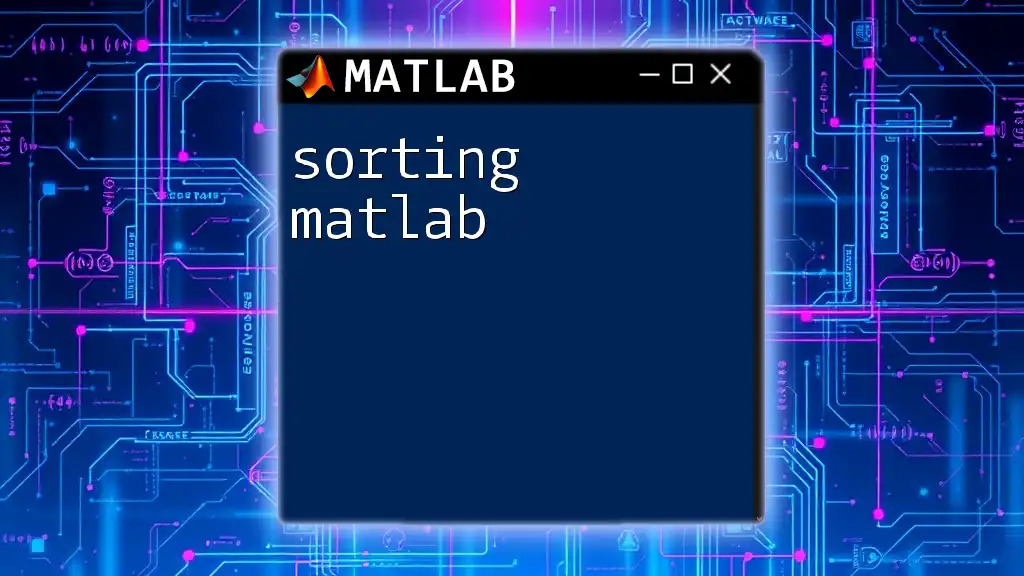
Setting Up Your MATLAB Environment
Installing MATLAB
To get started, you must first install MATLAB. Running the installer is straightforward. After installation, familiarize yourself with crucial features: the Command Window for immediate executing of commands, the Editor for writing scripts, and the Workspace for viewing variables.
Basic Commands and Syntax
Before diving into FFT, knowing MATLAB syntax is essential for seamless integration. Key commands to familiarize yourself with include:
- `plot`: For visualizing data.
- `xlabel`, `ylabel`: To label your axes for clarity.
- `hold on`: To overlay multiple plots on a single graph.
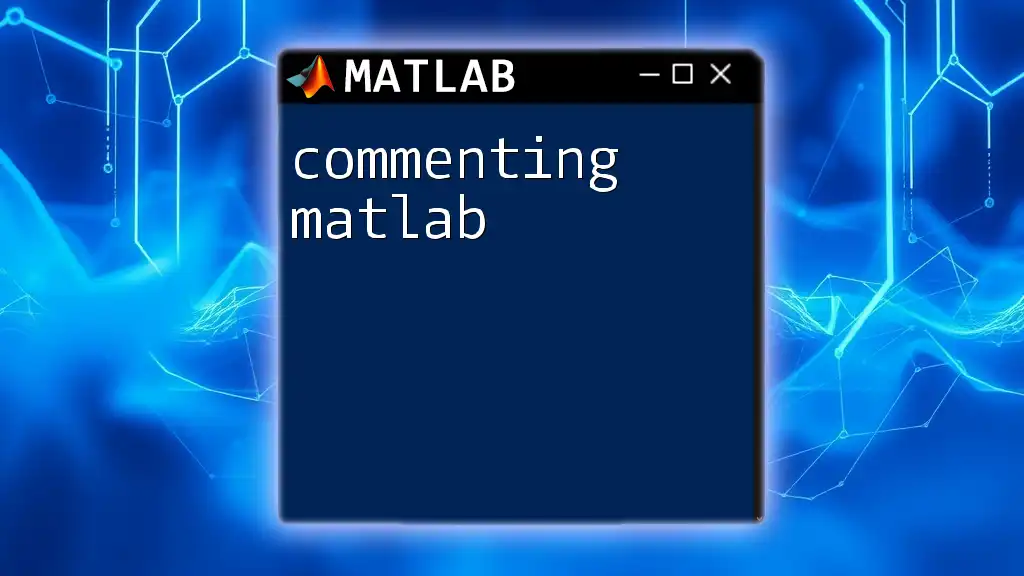
Performing FFT in MATLAB
Basic FFT Command Structure
The basic structure of the FFT command in MATLAB is `Y = fft(X)`, where `X` is your input data, and `Y` will be the output containing the frequency components.
Example 1: Simple FFT of a Sine Wave
Let’s start with an example of computing the FFT of a sine wave:
t = 0:0.001:1; % time vector
x = sin(2*pi*50*t); % 50 Hz sine wave
Y = fft(x); % Perform FFT
This code generates a 50 Hz sine wave and computes its FFT. The expected output should show peaks at 50 Hz.
Interpreting FFT Output
Interpreting the FFT results requires understanding the frequency spectrum. The output `Y` contains complex numbers representing both magnitude and phase information. To visualize the magnitude spectrum, we can plot the result:
f = (0:length(Y)-1)*fs/length(Y); % frequency axis
plot(f, abs(Y)); % plot frequency magnitude
xlabel('Frequency (Hz)');
ylabel('Magnitude');
This will give you a clear representation of how the sine wave's frequency is distributed.
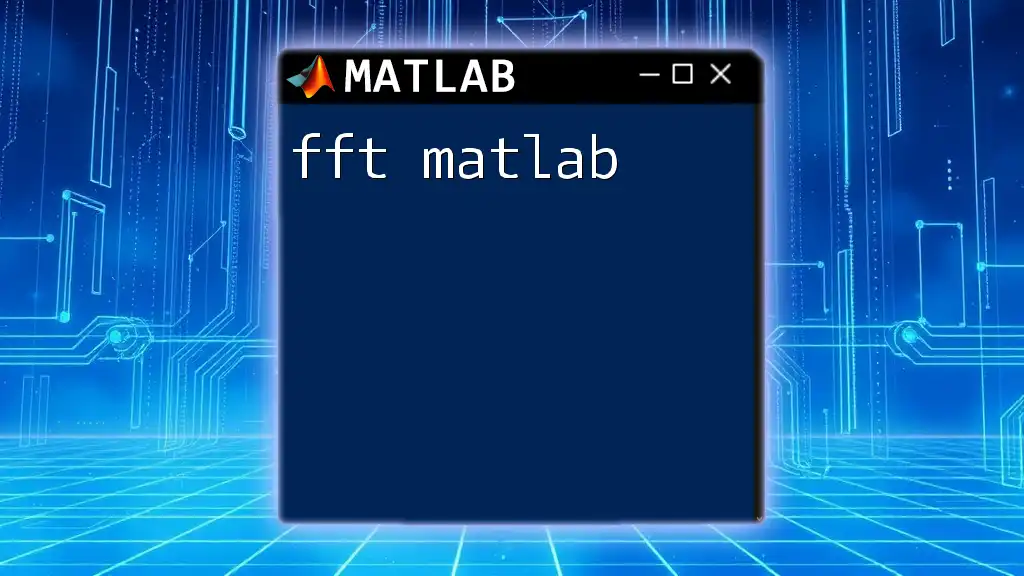
Advanced FFT Techniques
FFT with Different Types of Data
FFT can handle various input types, from real-valued signals to complex numbers. For complex inputs, the output will also be complex. Here's a code snippet to illustrate handling complex data:
x_complex = randn(1, 1024) + 1i * randn(1, 1024);
Y_complex = fft(x_complex);
This generates a random complex signal before applying FFT.
Zero Padding
Zero padding can be a useful technique to increase the frequency resolution of your FFT. By adding zeros to your dataset, you can achieve a higher resolution in the frequency domain. Here's how to implement this:
x_padded = [x, zeros(1, 1024-length(x))];
Y_padded = fft(x_padded);
In this case, if the length of `x` is less than 1024, the code extends it by appending zeros, thereby enhancing frequency resolution in the output.
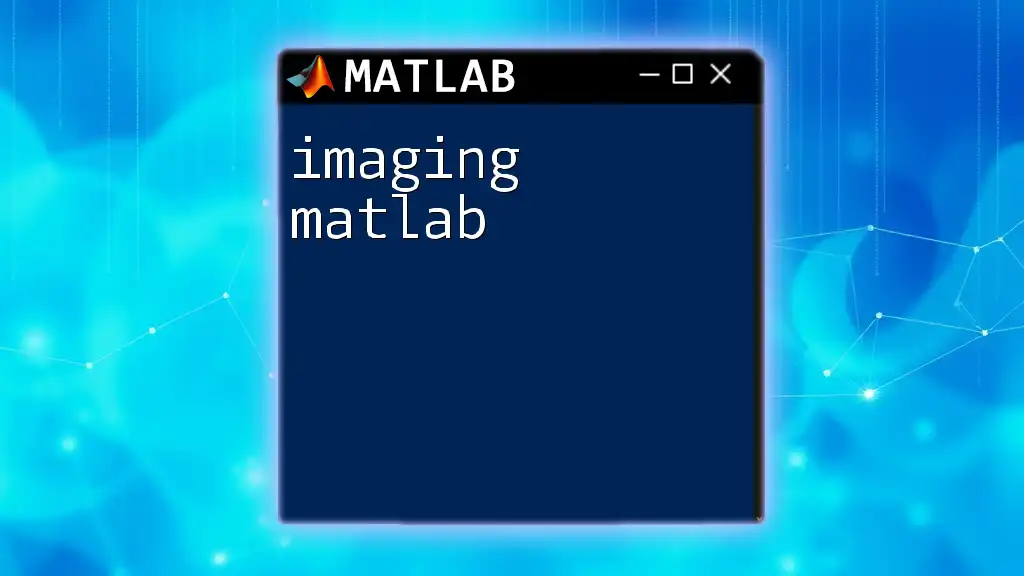
Applying Window Functions
Why Use Window Functions?
Window functions are crucial for reducing spectral leakage, which occurs when signals are clipped. To avoid distortions in the frequency domain, applying the appropriate window function before FFT is essential.
Common Window Functions in MATLAB
MATLAB supports various window functions, including Hamming, Hanning, and Blackman. A straightforward example is illustrated below:
w = hamming(length(x)); % Hamming window
X_windowed = x .* w';
Y_windowed = fft(X_windowed);
This code applies a Hamming window to the sine wave before computing the FFT, improving the accuracy of your frequency analysis.
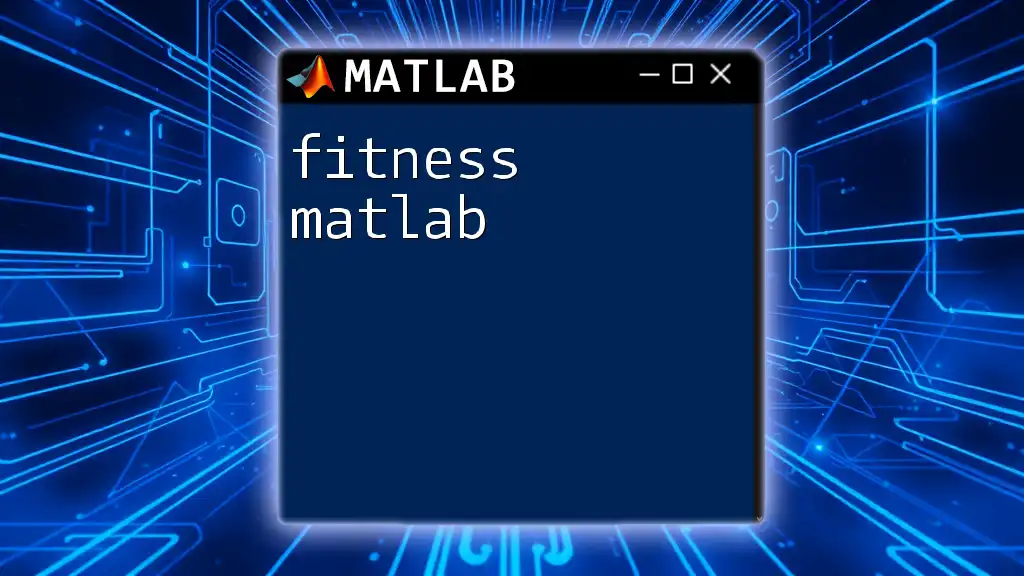
Practical Examples and Case Studies
Example 2: Audio Signal Analysis
FFT is particularly effective in audio signal analysis. Here’s how you can analyze a WAV file:
[y, Fs] = audioread('audiofile.wav');
Y_audio = fft(y);
f_audio = (0:length(Y_audio)-1)*Fs/length(Y_audio);
plot(f_audio, abs(Y_audio));
This script reads an audio file, computes its FFT, and plots the frequency spectrum, allowing you to identify dominant frequencies in the sound.
Example 3: Image Analysis Using FFT
FFT is also applicable in image processing. Using two-dimensional FFT, you can analyze and manipulate images:
img = imread('imagefile.png');
img_fft = fft2(img); % 2D FFT for images
This command computes the FFT for the image, allowing further processing like filtering or noise reduction.
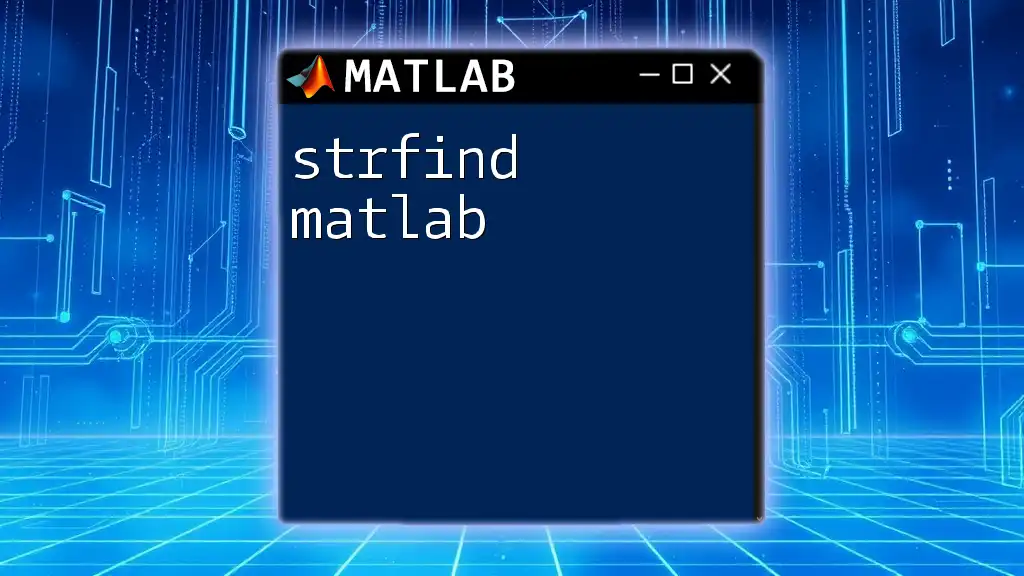
Performance and Optimization
Speeding Up FFT Calculations
For large datasets, optimizing FFT calculations is critical. Use built-in MATLAB functions like `fftw` for enhanced performance on large matrices. The integrated FFTW library allows MATLAB to leverage the fastest algorithms available.
Benchmarking FFT Performance
To gauge the performance of your FFT calculations, timing can be highly informative. Here’s a brief snippet to measure execution time:
tic; Y = fft(x); toc; % Measure execution time
This will help you understand how the implementation performs with different datasets.
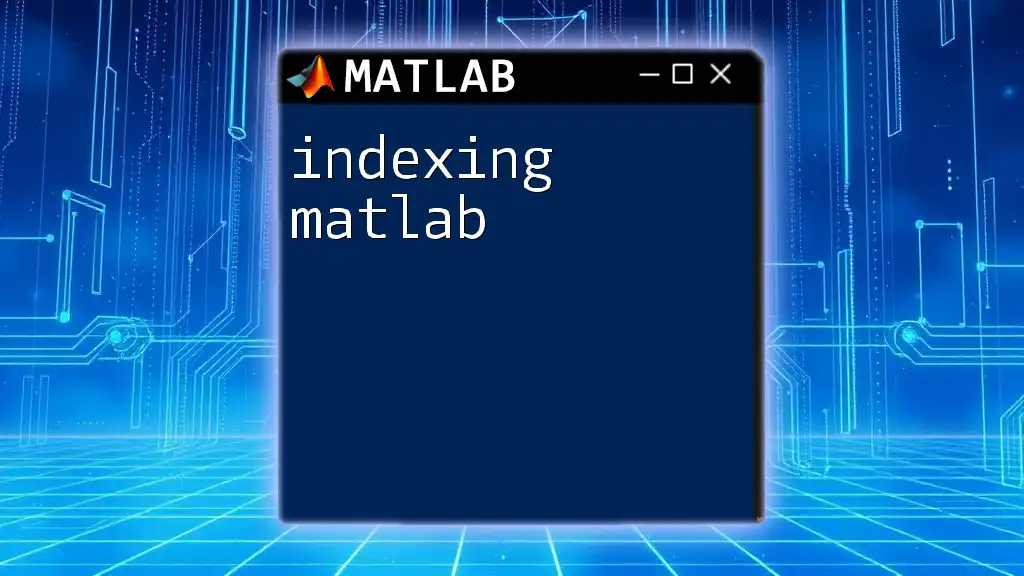
Troubleshooting Common Issues
Common Errors and Solutions
When working with FFT, it's common to misinterpret outputs. To effectively address discrepancies:
- Ensure your input data is correctly formatted.
- If handling real versus complex data, verify that you are expecting complex outputs when necessary.
Resources for Further Learning
For ongoing support, consider using resources such as MATLAB documentation, online tutorials, and community forums to enrich your knowledge base and troubleshoot issues effectively.
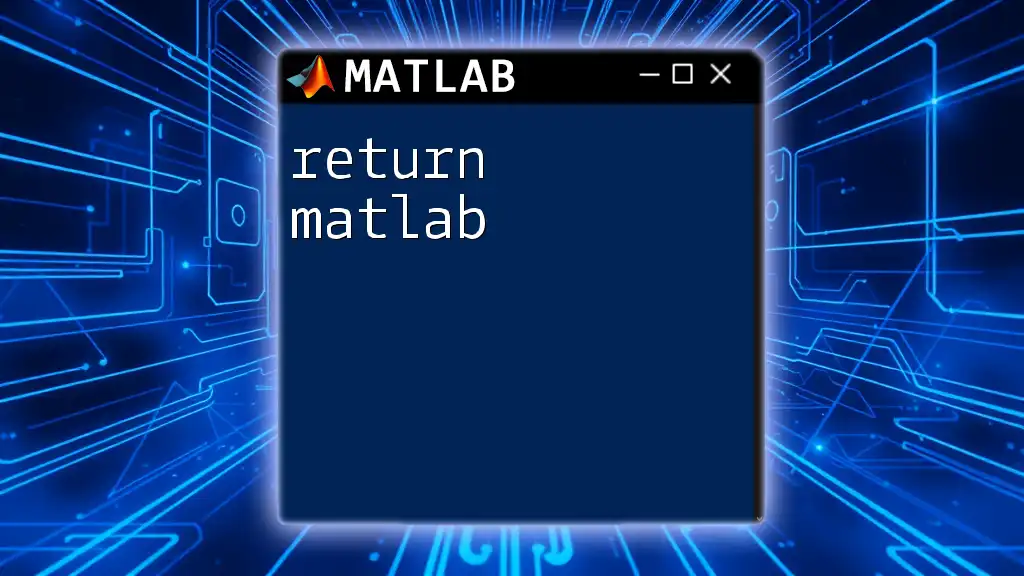
Conclusion
In summary, mastering FFT using MATLAB opens doors to vibrant applications across audio processing, image analysis, and signal interpretation. With practical examples and advanced techniques, MATLAB provides the tools needed for efficient data processing. As you explore and experiment with the techniques outlined, you'll enhance your proficiency and unlock the potential of signal manipulation through FFT.