The reduced row echelon form (RREF) of a matrix in MATLAB can be computed using the `rref` function, which simplifies the matrix to its canonical form, making it easier to solve systems of linear equations.
A = [1 2 -1 2; 2 4 -2 4; -1 -2 1 -2];
RREF_A = rref(A);
What is Reduced Row Echelon Form?
Reduced Row Echelon Form (RREF) is a specific form of a matrix that is utilized in various applications within linear algebra. A matrix is in RREF if it satisfies the following conditions:
- All rows consisting entirely of zeros are at the bottom of the matrix.
- The leading entry (also known as the pivot) in each non-zero row is 1.
- Each leading 1 is the only non-zero entry in its column.
- The leading 1 of a row is to the right of the leading 1 in the previous row.
Understanding RREF is crucial because it allows for the systematic solution of systems of linear equations and helps derive the properties of matrices, such as their rank.
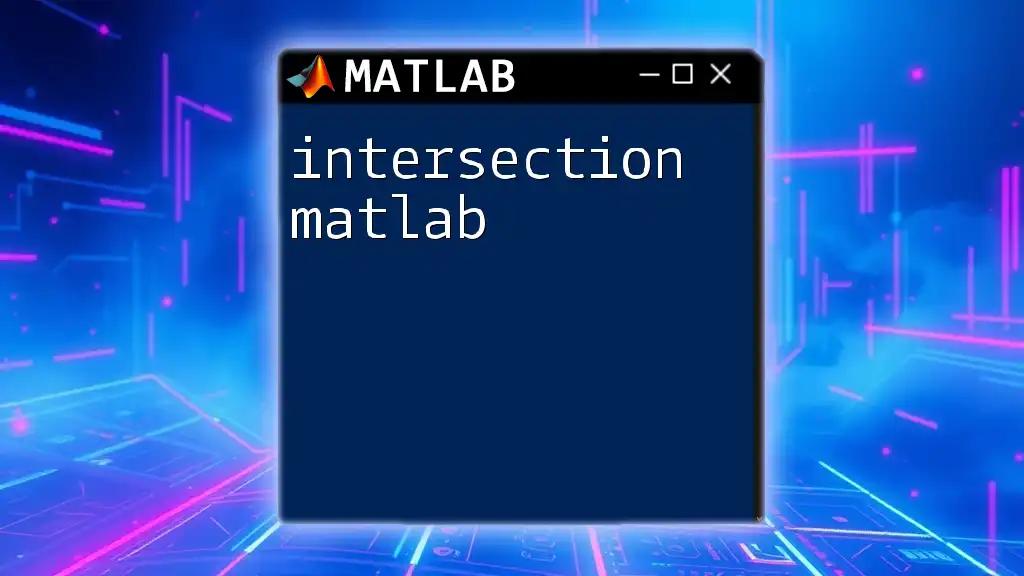
Overview of MATLAB and Its Capabilities
MATLAB, which stands for MATrix LABoratory, is a powerful computing environment widely used in engineering, mathematics, and scientific research. With an extensive range of mathematical functions, MATLAB provides users with efficient tools to manage and manipulate matrices, making it ideal for handling computations involving RREF.
Some features of MATLAB that assist in working with matrices include:
- A rich set of built-in functions for linear algebra operations.
- High-level programming language capabilities for automating processes.
- Excellent visualization options to graphically represent data.
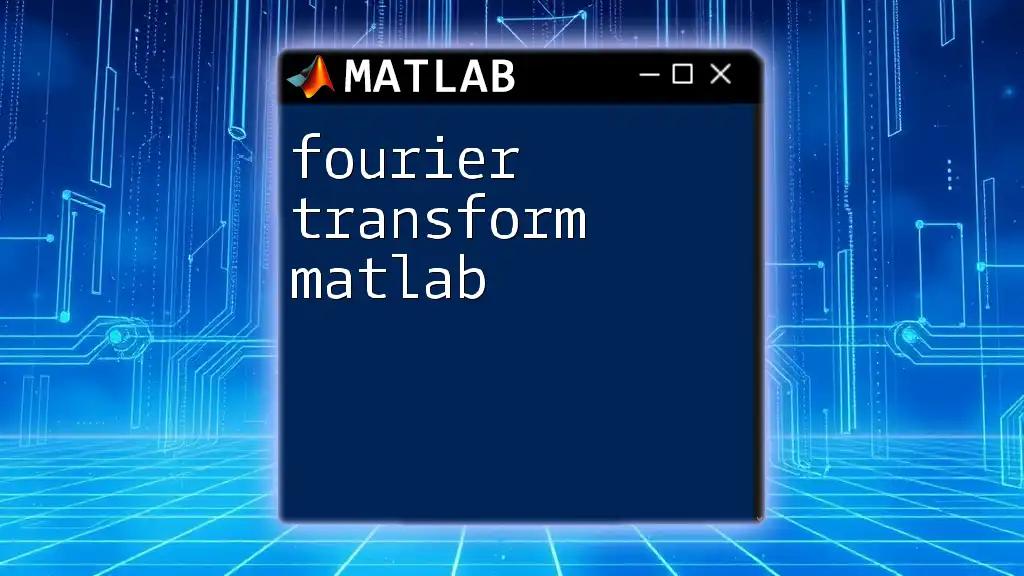
Understanding Reduced Row Echelon Form
Properties of RREF
RREF not only provides solutions to linear equations but also reveals essential attributes of the matrix. Some of these properties include:
- Uniqueness of Solution: A system of linear equations has a unique solution if its RREF has a pivot in every column of the coefficient matrix.
- Infinite Solutions: If there is at least one free variable in the RREF, it indicates that there could be infinitely many solutions.
- No Solution: If the RREF reveals a contradictory statement (like a row of zeros in the coefficient part and a non-zero in the augmented column), it implies the system of equations has no solutions.
Applications of RREF
RREF serves several important applications:
- Solving Systems of Linear Equations: By transforming the augmented matrix of a system into RREF, you can directly read the solutions.
- Understanding Rank: The number of leading 1s in the RREF gives the rank of the matrix, which is crucial in determining the dimensions of vector spaces.
- Modeling in Various Fields: In engineering and physics, RREF helps design control systems, analyze electrical circuits, and apply optimization techniques.

MATLAB Basics
Getting Started with MATLAB
To use MATLAB effectively:
- Installation: Download MATLAB from the official website and follow the installation instructions.
- Interface: Familiarize yourself with the editor, command window, and workspace to navigate and manage your projects efficiently.
Working with Matrices in MATLAB
Creating and manipulating matrices in MATLAB is straightforward, using simple commands:
-
To create a matrix, use square brackets.
A = [1 2 3; 4 5 6; 7 8 9];
-
MATLAB includes various functions, notably:
- `zeros(n)`: Creates an n-by-n matrix of zeros.
- `ones(n)`: Creates an n-by-n matrix of ones.
- `eye(n)`: Generates an n-by-n identity matrix.
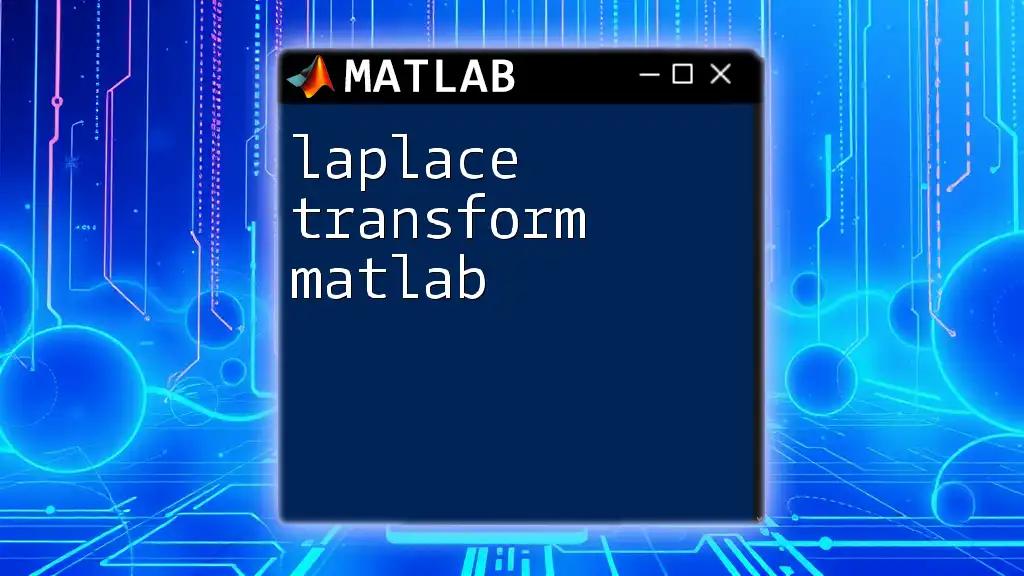
The RREF Function in MATLAB
Using the rref Command
To find the RREF of a matrix, MATLAB offers the built-in function `rref`. The basic syntax is as follows:
R = rref(A)
In this command, `A` is the matrix you wish to convert to RREF, and `R` will store the result.
Example of the rref Command
Here's a straightforward example of using the `rref` function. Let's take the following matrix:
A = [1 2 3; 2 4 6; 3 6 9];
R = rref(A);
disp(R);
Interpreting Results
The output of this command will be:
1 2 3
0 0 0
0 0 0
This means that the first row can be seen as a leading row, while the rest are entirely zeros, indicating that the original matrix has linearly dependent rows.
Applying RREF to Solve Systems of Equations
To solve a system of equations using RREF, create an augmented matrix that combines the coefficients of the variables and the constants from the equations.
Example: Solving a System of Linear Equations
Consider the following system of equations:
- 2x + y - z = 8
- -3x - y + 2z = -11
- -2x + y + 2z = -3
First, construct the augmented matrix:
A = [2 1 -1; -3 -1 2; -2 1 2];
b = [8; -11; -3];
augmented_matrix = [A b];
R = rref(augmented_matrix);
disp(R);
Conclusion of Example
After executing the above code, you might interpret the RREF to find the variables’ values based on the output. Each leading one corresponds to a variable, and any free variables will indicate how these solutions relate.
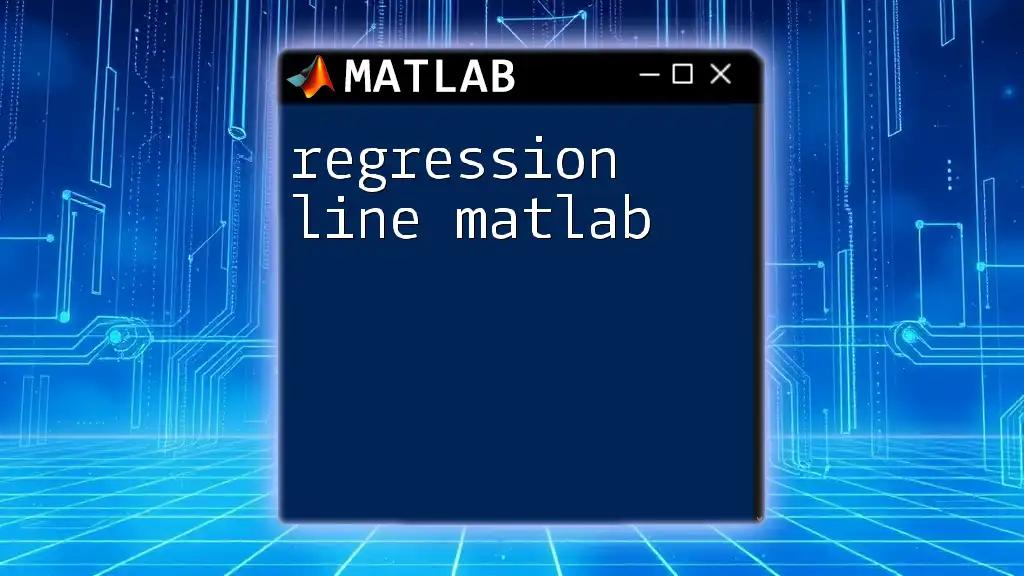
Troubleshooting Common Issues
Common Mistakes When Using rref
When working with the `rref` function, several mistakes could lead to confusion:
- Misinterpreting the Output: Make sure to differentiate between solutions when the RREF contains free variables.
- Augmented Matrix Errors: Forgetting to augment the matrix before using `rref` may yield erroneous or incomplete results.
Tips for Effective Use
- Ensure that the matrix is appropriately formatted. The rows and columns should logically represent your equations.
- Verify that the dimensions match (rows in coefficient matrix and constants).

Visualization and Interpretation
Visualizing Matrices and RREF
MATLAB provides robust plotting capabilities that can help visualize your matrices and their transformations. Typically, you can use functions like `plot`, `scatter`, and `mesh` to represent your findings graphically.
Graphical Representation of Linear Systems
By visually analyzing linear equations and their representations, you gain insights into the relationships between variables, which can aid in understanding the RREF process better.
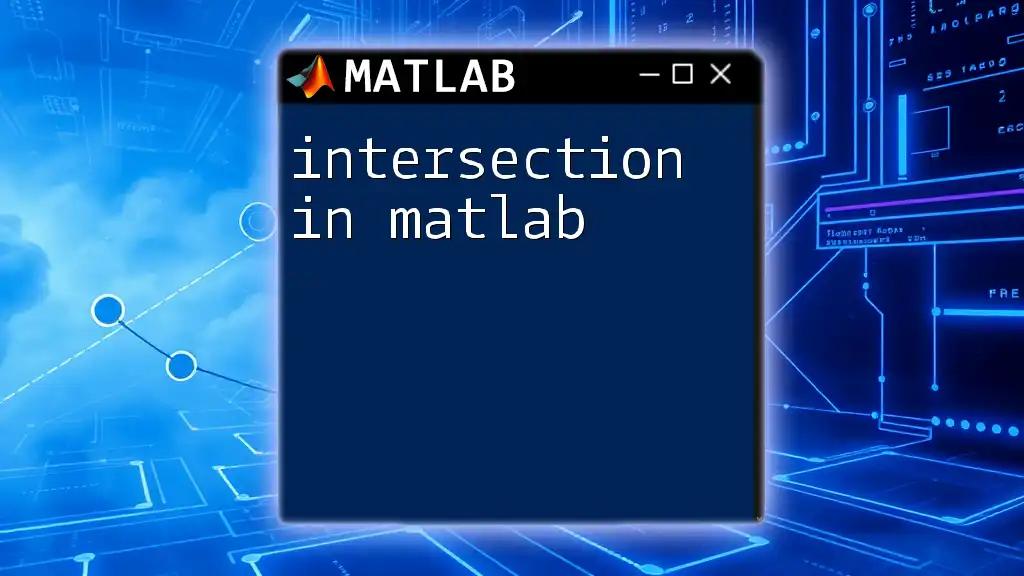
Conclusion
To summarize, understanding reduced row echelon form in MATLAB is a fundamental aspect of linear algebra. Utilizing the `rref` function not only simplifies finding solutions to systems of equations but also enhances the comprehension of a matrix’s properties. As you explore and apply RREF in MATLAB, remember to practice on different matrices to solidify your understanding and advancements in computational mathematics.

Further Resources
For those eager to deepen their understanding of RREF and MATLAB, consider engaging with literature on linear algebra, video tutorials, or online courses, which can provide a more visual and interactive learning experience.
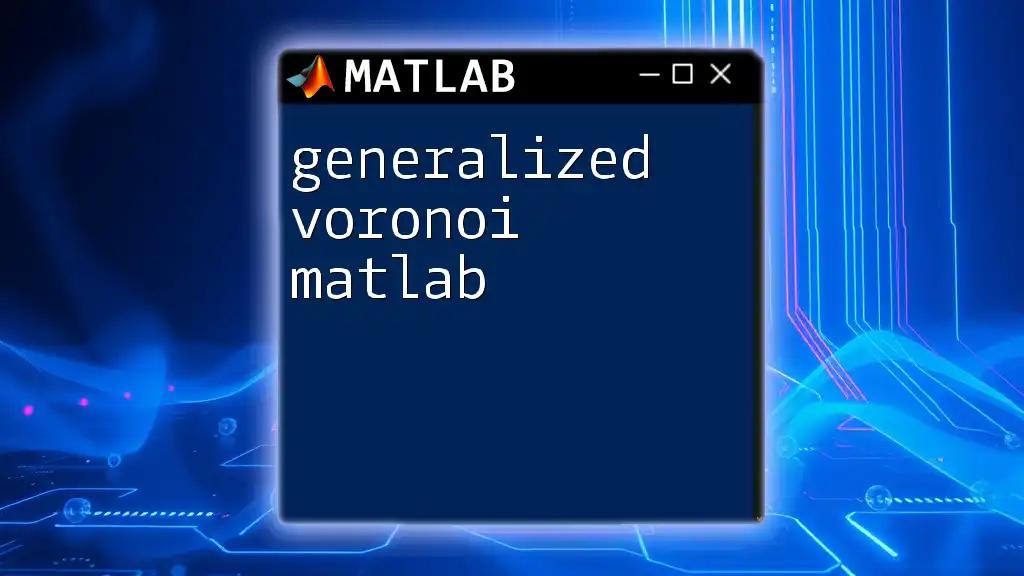
Call to Action
I encourage you to put this knowledge into practice. Try using `rref` on a set of equations or experiment with different matrices to explore the versatility of RREF. Don’t hesitate to share your findings or seek help from MATLAB user communities for additional support and insights as you improve your skills with MATLAB commands.